This article explains how to retrieve the browser name and version from the Angular application. In certain cases, you may also need to obtain the browser name in Angular, depending on your requirements. If the Angular application is open on Internet Explorer Legacy, Edge, Firefox, Chrome, Safari, Opera, or another browser, we can quickly identify it. This article walks us through the process of adding a reusable method to an Angular application and demonstrates how to quickly identify the browser and version.
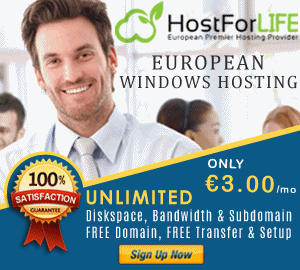
Note: Before beginning this session, please read my earlier essay (linked below) about Angular applications.
D:\EmployeeManagement\CallWebAPIAngular> ng g s services/detect-browser
Then you can service and its related files are shown below
PS D:\EmployeeManagement\CallWebAPIAngular> ng g s services/detect-browser
CREATE src/app/services/detect-browser.service.spec.ts (393 bytes)
CREATE src/app/services/detect-browser.service.ts (142 bytes)
Step 2. Configure browser name with version detection method
After creating the DetectBrowserService, add the getBrowserNameAndVersion() method to return an object with name and version keys. This method will fetch the User-Agent strings on the browser in which the Angular application is loaded.
Open the ~app\services\detect-browser.service.ts file and add as shown below.
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class DetectBrowserService {
constructor() { }
getBrowserNameAndVersion() {
var ua = navigator.userAgent,
tem,
M = ua.match(/(opera|chrome|safari|firefox|msie|trident(?=\/))\/?\s*(\d+)/i) || [];
if (/trident/i.test(M[1])) {
tem = /\brv[ :]+(\d+)/g.exec(ua) || [];
return { name: 'IE', version: (tem[1] || '') };
}
if (M[1] === 'Chrome') {
tem = ua.match(/\bEdg\/(\d+)/);
if (tem != null) {
return { name: 'Edge(Chromium)', version: tem[1] };
}
tem = ua.match(/\bOPR\/(\d+)/);
if (tem != null) {
return { name: 'Opera', version: tem[1] };
}
}
M = M[2] ? [M[1], M[2]] : [navigator.appName, navigator.appVersion, '-?'];
if ((tem = ua.match(/version\/(\d+)/i)) != null) {
M.splice(1, 1, tem[1]);
}
return {
name: M[0],
version: M[1]
};
}
}
Here, we detect the name and version of the browser by creating a new service with a common method. The getBrowserNameAndVersion method returns an object with name and version keys. This method is fetching the User-Agent string that provides the browser information.
Step 3. Detect browser name with version
Now, we will import the DetectBrowserService in the AppComponent‘s constructor function. The service instance method named getBrowserNameAndVersion() will assign the name and version object to the browserNameAndVersion variable.
Open the app.component.ts file and add as shown below
import { Component } from '@angular/core';
import { DetectBrowserService } from './services/detect-browser.service';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Display Browser Name & Version';
public requiredVersion = 98;
public isBrowserOutdated: boolean | undefined;
public browserVersion = '';
browserNameAndVersion: any;
constructor(
detectBrowserService: DetectBrowserService
) {
this.browserNameAndVersion = detectBrowserService.getBrowserNameAndVersion();
this.browserVersion = this.browserNameAndVersion.version;
if (parseFloat(this.browserNameAndVersion.version) < this.requiredVersion) {
this.isBrowserOutdated = true;
}
}
}
Here we configure for displaying browser name and version along with browser up-to-date or not.
this.browserNameAndVersion = detectBrowserService.getBrowserNameAndVersion();
this.browserVersion = this.browserNameAndVersion.version;
if (parseFloat(this.browserNameAndVersion.version) < this.requiredVersion) {
this.isBrowserOutdated = true;
}
Step 4. HTML template to show browser name with version
Update the HTML template of AppComponent to display the browser name and version. Open the app.component.html file and update it as shown below:
<div class="card bg-danger text-white">
<div class="card-body text-center">
<h1>{{ title }}</h1>
</div>
<table class="table table-striped mt-5">
<thead>
</thead>
<tbody>
<tr>
<td><h4>Browser Name: {{ browserNameAndVersion.name | titlecase }}</h4></td>
<td><h4>Current Browser Version: {{ browserNameAndVersion.version | titlecase }}</h4></td>
<td><h4>Required Version: {{ requiredVersion }}</h4></td>
</tr>
</tbody>
</table>
</div>
<div class="toast show" style="position: fixed; top: 60%; left: 50%; width: 30em; height: 17em; margin-top: -9em; margin-left: -15em; border: 1px solid #ccc; background-color: #f3f3f3; color: red; font-weight: 900; font-size: larger;">
<div class="toast-header">
Angular Detect Browser Name and Version
<button type="button" class="btn-close" data-bs-dismiss="toast"></button>
</div>
<div class="toast-body">
<div class="base-block" *ngIf="isBrowserOutdated">
<h1>Oops</h1>
<h3>Browser is outdated</h3>
<h5>
Your {{ browserNameAndVersion.name }} browser is outdated ({{ browserNameAndVersion.version }}) as less than required Version
({{ requiredVersion }}) and no longer supported, please update your browser.
</h5>
</div>
<div class="base-block" *ngIf="!isBrowserOutdated">
<h1>Cheers!</h1>
<h3>Browser is up to date</h3>
<h5>
Your {{ browserNameAndVersion.name }} browser is updated ({{ browserNameAndVersion.version }}).
</h5>
</div>
</div>
</div>
Use the interpolation to display the browser name and version with the help of the interpolation. An Interpolation is defined using the double curly braces that permit the user to bind a value to a UI element. Also, we will use the angular pipe to transform the value in the title case.
<td>
<h4>Browser Name: {{ browserNameAndVersion.name | titlecase }}</h4>
</td>
<td>
<h4>Current Browser Version: {{ browserNameAndVersion.version | titlecase }}</h4>
</td>
<td>
<h4>Required Version: {{ requiredVersion }}</h4>
</td>
Check browser is up-to-date or not based on some condition using the *ngIf directive.
<div class="toast-body">
<div class="base-block" *ngIf="isBrowserOutdated">
<h1>Oops</h1>
<h3>Browser is outdated</h3>
<h5>
Your {{ browserNameAndVersion.name }} browser is outdated ({{ browserNameAndVersion.version }}) as less than required Version
({{ requiredVersion }}) and no longer supported, please update your browser.
</h5>
</div>
<div class="base-block" *ngIf="!isBrowserOutdated">
<h1>Cheers!</h1>
<h3>Browser is up to date</h3>
<h5>
Your {{ browserNameAndVersion.name }} browser is updated ({{ browserNameAndVersion.version }}).
</h5>
</div>
</div>
Step 5. Add Bootstrap to make the page responsive
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Title Service Example</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<app-root></app-root>
</body>
</html>
Output
Finally, run the application by executing the ng serve command in the terminal window. It opens the application at the following URL: http://localhost:4200/
Checking Chrome Browser is up to date i.e. based required Version
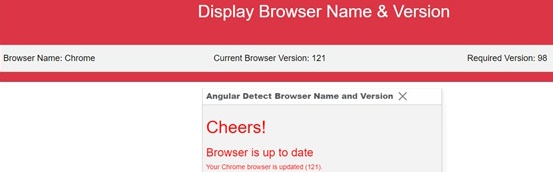
Checking whether Chrome Browser is up to date or not i.e. based on the Required Version
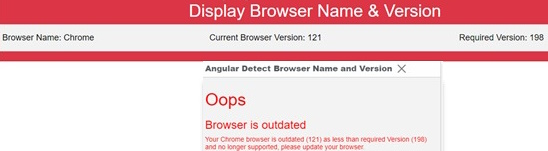
Checking whether another Browser is up to date or not i.e. based on the Required Version
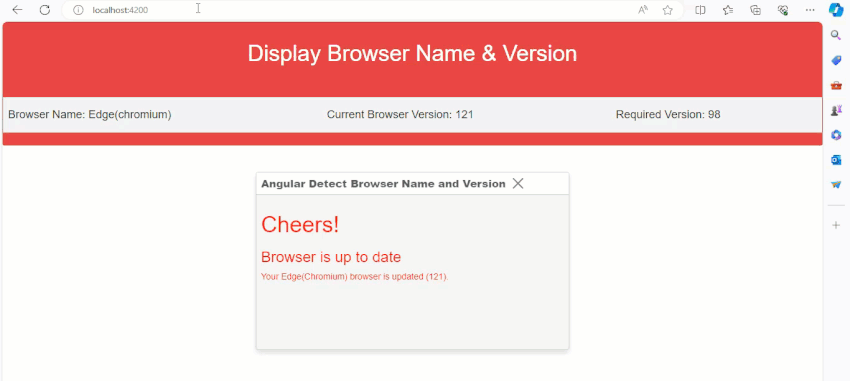
Why is it necessary to set up the browser detection procedure?
To let users know that their current browser or version isn't compatible with correctly loading the application, we need to identify the name and version of their browser. They can then use the newest browsers that are out there. Modern Javascript and CSS principles are supported by a wide range of web browsers accessible today, all of which are completely compatible with the most recent version of WebKit. In this situation, we must identify the browser's name and version in order to let users know that their current browser or a different one isn't compatible with correctly loading the program and that they can upgrade to the newest version.