
August 25, 2023 08:22 by
Peter
ng-container
By enabling you to apply Angular directives without including additional items in the DOM (Document Object Model), the ng-container functions as a kind of transparent container. It is frequently employed when you wish to structure or conditionally include elements in your template.
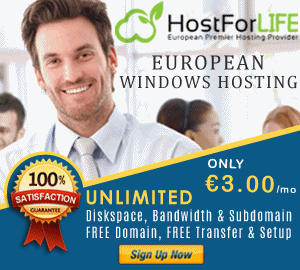
As an illustration, consider creating a product page. If the product is in stock, you want to display the product details; otherwise, you want to display a notification stating the product is unavailable.
<ng-container *ngIf="productAvailable; else outOfStockTemplate">
<div>Product Name: {{ productName }}</div>
<div>Price: {{ productPrice }}</div>
</ng-container>
<ng-template #outOfStockTemplate>
<div>This product is currently out of stock.</div>
</ng-template>
ng-template
The ng-template is like a template blueprint that doesn't get displayed immediately. It's used to define sections of your template that can be reused and conditionally rendered using structural directives.
Example: Let's say you're creating a list of blog posts. You want to show different styles for featured posts and regular posts.
<ul>
<ng-container *ngFor="let post of posts">
<ng-container *ngTemplateOutlet="post.featured ? featuredTemplate : regularTemplate; context: { post: post }"></ng-container>
</ng-container>
</ul>
<ng-template #featuredTemplate let-post>
<li class="featured">{{ post.title }}</li>
</ng-template>
<ng-template #regularTemplate let-post>
<li>{{ post.title }}</li>
</ng-template>
ng-content
The ng-content is used to allow external content to be included within the template of your component. It's ideal for making more adaptable components that can be used in a variety of situations. Consider designing a card component that can display a variety of content.
<div class="card">
<div class="card-header">
<ng-content select=".card-title"></ng-content>
</div>
<div class="card-content">
<ng-content></ng-content>
</div>
<div class="card-footer">
<ng-content select=".card-actions"></ng-content>
</div>
</div>
Now, when you use this component, you can provide custom content for each section:
<app-card>
<div class="card-title">Card Title</div>
<p>This is the content of the card.</p>
<div class="card-actions">
<button>Edit</button>
<button>Delete</button>
</div>
</app-card>
By combining these concepts, you can create components that are more dynamic and adaptable. ng-container, ng-template, and ng-content help you organize and structure your templates in a way that makes your code more readable and maintainable.