
July 6, 2023 09:26 by
Peter
DALL-E, developed by OpenAI, is an AI model that can generate images from textual descriptions. It combines the power of deep learning and generative modeling to create unique and imaginative visual outputs based on the provided text prompts. This technology has sparked excitement among researchers, artists, and developers alike.
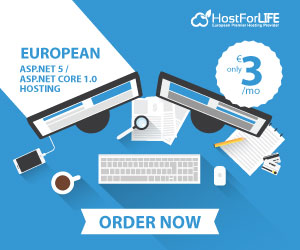
To make the capabilities of DALL-E accessible to developers, OpenAI has released the DALL-E API. This API allows developers to interact with the DALL-E model programmatically; by using this, developers can generate images by giving text prompts. By using Node.js and DALL-E, we can generate images programmatically.
Note. If you want to achieve this using Python, Please read my previous article on how to Generate Image Using DALL-E API and Python.
Prerequisites
It is essential to have a basic understanding of the following concepts and technologies.
Node.js
Node.js is a JavaScript runtime environment that allows you to run JavaScript code outside of a web browser. To use Node.js, you'll need to install it on your machine. Install Node.js from the official website.
Basic API Concepts
A basic understanding of API (Application Programming Interface) concepts is crucial. APIs provide a way for different software applications to communicate and exchange data. Familiarize yourself with concepts like endpoints, HTTP methods (GET, POST, etc.), request/response structure, and how to handle API responses using JavaScript.
Setting Up the Project
Open your terminal or command prompt and follow these steps.
Create a new directory for your Project.
mkdir dalle-image
cd dalle-image
Initialize a new Node.js project by running the following command.
npm init -y
This will create a package.json file, keeping track of your Project's dependencies.
Install Required Dependencies
Install the Axios library to make API requests to the DALL-E API. Axios is a popular JavaScript library for making HTTP requests. It simplifies the process of sending asynchronous requests and handling responses. To install it, use the following command
npm install axios
Now, Axios is added to your Project's dependencies, and you can start using it to interact with the DALL-E API.
Writing the Node.js Code
Write the code to interact with the DALL-E API using Node.js. Follow the steps below.
Setting up the API Endpoint and Authorization
Navigate to the dalle-image directory and create a new JavaScript file, for example, dalle-api.js.
Require the Axios module at the top.
const axios = require('axios');
Next, declare a constant variable to store your DALL-E API key.
const API_KEY = 'YOUR_API_KEY';
Implementing the API Call using Axios
Implement the API call using Axios.
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY'; //replace with your key
const generateImages = async () => {
try {
const response = await axios.post(
'https://api.openai.com/v1/images/generations',
{
prompt: 'A beautiful sunset over a serene lake',
n: 1, //define the number of images
size: '512x512', //define the resolution of image
},
{
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${API_KEY}`,
},
}
);
console.log(response.data);
// Handle the response here, e.g., extract image data and display or save it.
} catch (error) {
console.error('Error:', error.response.data);
}
};
generateImages();
In the code above, we use the Axios. post method to make a POST request to the API endpoint. We pass the request payload as the second argument to the method and include the API key in the request headers.
Now run the Project using the following command.
node dalle-api.js
Check the console output to see the API response or any errors that occurred during the process.
Output

July 3, 2023 08:45 by
Peter
Reactive Extensions for JavaScript (RxJS) have become the backbone of modern Angular applications, enabling developers to handle complex asynchronous operations with elegance and ease. While many developers are familiar with basic RxJS operators, there are advanced patterns that can take your Angular development skills to the next level. In this article, we will explore some lesser-known yet powerful RxJS patterns that can significantly improve your developer experience.
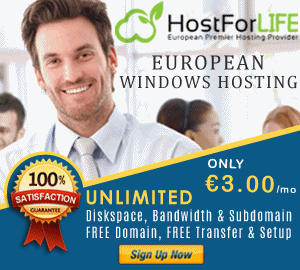
1. Canceling Previous Requests with SwitchMap
One common scenario is handling user input, such as search queries, that trigger API calls. To prevent outdated responses and save bandwidth, we can use the switchMap operator. It automatically cancels previous requests when a new request is made.
Let's take a look at an example.
import { fromEvent } from 'rxjs';
import { switchMap } from 'rxjs/operators';
// Get the search input element
const searchInput = document.getElementById('search-input');
// Create an observable from input events
const searchObservable = fromEvent(searchInput, 'input').pipe(
map((event: any) => event.target.value),
switchMap(searchTerm => makeApiCall(searchTerm))
);
searchObservable.subscribe(response => {
// Handle the API response
});
2. Throttling API Calls with DebounceTime
In scenarios where user input or events trigger API calls, we might want to throttle the rate of API requests to avoid overloading the server. The debounceTime operator can help us achieve this by delaying the emission of values from an Observable. Let's see it in action.
import { fromEvent } from 'rxjs';
import { debounceTime, distinctUntilChanged } from 'rxjs/operators';
// Get the search input element
const searchInput = document.getElementById('search-input');
// Create an observable from input events
const searchObservable = fromEvent(searchInput, 'input').pipe(
map((event: any) => event.target.value),
debounceTime(300),
distinctUntilChanged(),
switchMap(searchTerm => makeApiCall(searchTerm))
);
searchObservable.subscribe(response => {
// Handle the API response
});
3. Sharing Cached Results with ShareReplay
Sometimes, we want multiple subscribers to receive the same cached result from an Observable. The shareReplay operator enables us to achieve this by caching and sharing emitted values. This is useful in scenarios where we want to avoid redundant API calls. Let's take a look.
import { interval } from 'rxjs';
import { take, shareReplay } from 'rxjs/operators';
// Create an interval observable that emits values every second
const sourceObservable = interval(1000).pipe(
take(5),
shareReplay(1) // Cache and share the emitted values
);
// Subscriber 1
sourceObservable.subscribe(value => {
console.log(`Subscriber 1: ${value}`);
});
// Subscriber 2
setTimeout(() => {
sourceObservable.subscribe(value => {
console.log(`Subscriber 2: ${value}`);
});
}, 3000);
4. Sequential Requests with ExhaustMap
In certain scenarios, we may need to perform a series of actions that depend on the completion of previous actions. The exhaustMap operator allows us to achieve this by ignoring new source values until the current inner Observable completes. Let's see it in action.
import { fromEvent } from 'rxjs';
import { exhaustMap } from 'rxjs/operators';
// Get the button element
const button = document.getElementById('button');
// Create an observable from button click events
const clickObservable = fromEvent(button, 'click').pipe(
exhaustMap(() => performAction())
);
clickObservable.subscribe(() => {
// Handle the action completion
});
5. Error Handling and Retries with RetryWhen
Handling errors and implementing retry logic is essential for robust applications. The `retryWhen` operator allows us to handle errors and retry the Observable based on specific conditions. Let's take a look.
import { throwError, timer } from 'rxjs';
import { mergeMap, retryWhen, delayWhen } from 'rxjs/operators';
// Create an observable that throws an error
const errorObservable = throwError('Something went wrong!');
// Retry the observable after a delay
errorObservable.pipe(
retryWhen(errors =>
errors.pipe(
mergeMap((error, index) => {
if (index === 2) {
return throwError('Max retries reached'); // Throw error after 3 attempts
}
return timer(2000); // Retry after a 2-second delay
})
)
)
).subscribe({
next: () => console.log('Success!'),
error: (error) => console.log(`Error: ${error}`)
});
By harnessing the power of advanced RxJS patterns in Angular, we can greatly enhance our developer experience. The switchMap, debounceTime, shareReplay, exhaustMap, retryWhen, and other operators provide us with powerful tools to handle complex asynchronous scenarios, improve performance, and build robust applications.