Preconditions
Basic knowledge of Angular CLI
Bootstrap
Node.js
V.S. Code
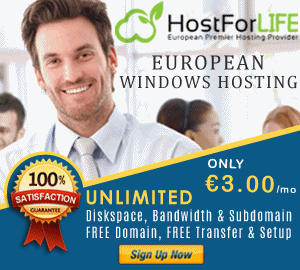
We Covers the belowing things
Create Angular application
Create header,side menu and layout component with admin module.
Angular Routing
Kendo UI setup
Now let's use following command to create angular project,
ng new KendoUI
cd KendoUI
Now install bootstrap by using the following command,
npm install bootstrap --save
Now install KendoUI packages using following command,
npm install --save @progress/kendo-angular-charts @progress/kendo-angular-common @progress/kendo-angular-intl @progress/kendo-angular-l10n @progress/kendo-angular-popup @progress/kendo-drawing hammerjs @progress/kendo-licensing
Now install KendoUI theme using following command, I used default theme,
Default theme
npm install --save @progress/kendo-theme-default
Bootstrap theme
npm install --save @progress/kendo-theme-bootstrap
Material theme
npm install --save @progress/kendo-theme-material
Now Create the dashboard and layout component using the following command,
ng g c dashboard
ng g c layout
Now add the code in the following pages-:
Add this in app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { GridModule } from '@progress/kendo-angular-grid';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { LayoutComponent } from './layout/layout.component';
import { DashboardComponent } from './dashboard/dashboard.component';
import { InputsModule } from '@progress/kendo-angular-inputs';
import { LabelModule } from '@progress/kendo-angular-label';
import { ChartsModule } from '@progress/kendo-angular-charts';
import { ButtonsModule } from '@progress/kendo-angular-buttons';
import { DialogModule } from '@progress/kendo-angular-dialog';
import { UploadModule } from '@progress/kendo-angular-upload';
import { FileSelectModule } from '@progress/kendo-angular-upload';
import { PDFExportModule } from '@progress/kendo-angular-pdf-export';
import { NotificationModule } from '@progress/kendo-angular-notification';
import { NavigationModule } from '@progress/kendo-angular-navigation';
import { IconsModule } from '@progress/kendo-angular-icons';
import { IndicatorsModule } from '@progress/kendo-angular-indicators';
import { LayoutModule } from '@progress/kendo-angular-layout';
@NgModule({
declarations: [
AppComponent,
LayoutComponent,
DashboardComponent
],
imports: [
BrowserModule,
AppRoutingModule,
GridModule,
BrowserAnimationsModule,
InputsModule,
LabelModule,
ChartsModule,
ButtonsModule,
DialogModule,
UploadModule,
FileSelectModule,
PDFExportModule,
NotificationModule,
NavigationModule,
IconsModule,
IndicatorsModule,
LayoutModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Add this in app.component.html
<router-outlet></router-outlet>
Add this in app-routing.module.ts
import {
NgModule
} from '@angular/core';
import {
RouterModule,
Routes
} from '@angular/router';
import {
DashboardComponent
} from './dashboard/dashboard.component';
import {
LayoutComponent
} from './layout/layout.component';
const routes: Routes = [{
path: 'Admin',
component: LayoutComponent,
children: [{
path: 'Dashboard',
component: DashboardComponent
}]
}, {
path: '**',
redirectTo: "/Admin/Dashboard",
pathMatch: 'full'
}, {
path: '',
redirectTo: "/Admin/Dashboard",
pathMatch: 'full'
}];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule {}
Add this in layout.component.ts
import {
Component,
OnInit
} from '@angular/core';
@Component({
selector: 'app-layout',
templateUrl: './layout.component.html',
styleUrls: ['./layout.component.css']
})
export class LayoutComponent implements OnInit {
private data: any;
public kendokaAvatar = '/assets/img/0.jpg';
public logout = '/assets/img/logout.png';
constructor() {}
ngOnInit(): void {}
public bottomNavigationItems: Array < any > = [{
text: 'Home',
icon: 'home',
selected: true
}, {
text: 'Calendar',
icon: 'calendar'
}, {
text: 'Notifications',
icon: 'bell'
}];
}
Add this in layout.component.html
<!--The content below is only a placeholder and can be replaced.-->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="">
<title>Kendo</title>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<!-- <kendo-breadcrumb [items]="breadCrumbItems"></kendo-breadcrumb> -->
<!-- <kendo-bottomnavigation [border]="true" [items]="bottomNavigationItems"></kendo-bottomnavigation> -->
<br />
<div id="wrapper">
<!-- Navigation -->
<nav class="navbar navbar-default navbar-static-top" role="navigation" style="margin-bottom: 0">
<!-- <div class="navbar-header"> -->
<!-- <button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button> -->
<!-- <a class="navbar-brand" href="index.html">Kendo Project</a> -->
<!-- </div> -->
<!-- /.navbar-header -->
<!-- /.navbar-top-links -->
<kendo-appbar position="top">
<!-- <kendo-appbar-section>
<button class="k-button k-button-clear">
<kendo-icon name="menu"></kendo-icon>
</button>
</kendo-appbar-section> -->
<!-- <kendo-appbar-section>
<h1 class="title">Kendo Project</h1>
</kendo-appbar-section> -->
<kendo-appbar-spacer width="32px"></kendo-appbar-spacer>
<kendo-appbar-section>
<ul>
<li><a class="DashFont" [routerLink]="['/Admin/Dashboard']">Dashboard</a></li>
<!-- <li><a [routerLink]="['/Admin/userlist']">Users</a></li>
<li><a [routerLink]="['/Admin/profile']">Profile</a></li>
<li><a [routerLink]="['/Admin/userserver']">User List</a></li> -->
</ul>
</kendo-appbar-section>
<kendo-appbar-spacer></kendo-appbar-spacer>
<kendo-appbar-section>
<a [routerLink]="['/Admin/profile']"><kendo-avatar [imageSrc]="kendokaAvatar" shape="circle" width="26px" height="26px"> </kendo-avatar></a>
</kendo-appbar-section>
<kendo-appbar-section class="actions">
<a [routerLink]="['/AdminLogout']"><kendo-avatar [imageSrc]="logout" shape="circle" width="26px" height="26px"> </kendo-avatar></a>
<kendo-badge-container>
<button class="k-button k-button-clear">
<kendo-icon name="bell"></kendo-icon>
</button>
<kendo-badge shape="dot" themeColor="warning" size="small" position="inside"></kendo-badge>
</kendo-badge-container>
<span class="k-appbar-separator"></span>
</kendo-appbar-section>
</kendo-appbar>
<!-- <div class="navbar-default sidebar" role="navigation">
<div class="sidebar-nav navbar-collapse">
<ul class="nav" id="side-menu">
<li>
<a [routerLink]="['/Admin/Dashboard']"><i class="fa fa-edit fa-fw"></i>Dashboard</a>
</li>
<li>
<a [routerLink]="['/Admin/userlist']"><i class="fa fa-edit fa-fw"></i>Users List</a>
</li>
<li>
<a [routerLink]="['/Admin/userserver']"><i class="fa fa-edit fa-fw"></i>Users Server List</a>
</li>
<li>
<a [routerLink]="['/Admin/imagegrid']"><i class="fa fa-edit fa-fw"></i>Image Grid</a>
</li>
<li>
<a [routerLink]="['/AdminLogout']"><i class="fa fa-edit fa-fw"></i>Logout</a>
</li>
</ul>
</div>
</div> -->
</nav>
<div id="page-wrapper">
<div class="row">
<div class="col-lg-12">
<router-outlet></router-outlet>
</div>
<!-- /.col-lg-12 -->
</div>
<!-- /.row -->
</div>
</div>
<!-- /#page-wrapper -->
<!-- /#wrapper -->
</body>
</html>
Add this inlayout.component.css
: host {
padding: 0;
}
kendo - appbar.title {
font - size: 18 px;
margin: 10 px;
}
kendo - badge - container {
margin - right: 8 px;
}
kendo - appbar ul {
font - size: 14 px;
list - style - type: none;
padding: 0;
margin: 0;
display: flex;
}
kendo - appbar li {
margin: 0 9 px;
}
kendo - appbar li: hover {
cursor: pointer;
color: #d6002f;
}
kendo - appbar.actions.k - button {
padding: 0;
}
kendo - breadcrumb {
margin - left: 30 px;
}.DashFont {
font - size: 16 px;
font - family: 'Segoe UI', Tahoma, Geneva, Verdana, sans - serif;
}
Add this in dashboard.component.ts
import {
Component,
OnInit
} from '@angular/core';
@Component({
selector: 'app-dashboard',
templateUrl: './dashboard.component.html',
styleUrls: ['./dashboard.component.css']
})
export class DashboardComponent implements OnInit {
ngOnInit(): void {}
constructor() {}
public events: string[] = [];
public series: any[] = [{
name: "India",
data: [
3.907, 7.943, 7.848, 9.284, 9.263, 9.801, 3.89, 8.238, 9.552, 6.855,
],
}, {
name: "Russian Federation",
data: [4.743, 7.295, 7.175, 6.376, 8.153, 8.535, 5.247, -7.832, 4.3, 4.3],
}, {
name: "Germany",
data: [
0.01, -0.375, 1.161, 0.684, 3.7, 3.269, 1.083, -5.127, 3.69, 2.995,
],
}, {
name: "World",
data: [
1.988, 2.733, 3.994, 3.464, 4.001, 3.939, 1.333, -2.245, 4.339, 2.727,
],
}, ];
public categories: number[] = [
2002, 2003, 2004, 2005, 2006, 2007, 2008, 2009, 2010, 2011,
];
public onRender(): void {
this.log("render");
}
public onAxisLabelClick(e: any): void {
this.log("axisLabelClick", e);
}
public onLegendItemClick(e: any): void {
this.log("legendItemClick", e);
}
public onLegendItemHover(e: any): void {
this.log("legendItemHover", e);
}
public onPlotAreaClick(e: any): void {
this.log("plotAreaClick", e);
}
public onPlotAreaHover(e: any): void {
this.log("plotAreaHover", e);
}
public onSeriesClick(e: any): void {
this.log("seriesClick", e);
}
public onSeriesHover(e: any): void {
this.log("seriesHover", e);
}
private log(event: string, arg: any = null): void {
this.events.push(`${event}`);
console.log(arg);
}
public data: any[] = [{
kind: 'Hydroelectric',
share: 0.175
}, {
kind: 'Nuclear',
share: 0.238
}, {
kind: 'Coal',
share: 0.118
}, {
kind: 'Solar',
share: 0.052
}, {
kind: 'Wind',
share: 0.225
}, {
kind: 'Other',
share: 0.192
}];
public labelContent(e: any): string {
return e.category;
}
}
Add this in dashboard.component.html
<kendo-chart>
<kendo-chart-title text="Units sold"></kendo-chart-title>
<kendo-chart-category-axis>
<kendo-chart-category-axis-item
[categories]="['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'august' , 'september' , 'October', 'november', 'December']"
[title]="{ text: 'Months' }">
</kendo-chart-category-axis-item>
</kendo-chart-category-axis>
<kendo-chart-series-defaults [highlight]="{ inactiveOpacity: 0.3 }">
</kendo-chart-series-defaults>
<kendo-chart-series>
<kendo-chart-series-item type="area" [data]="[123, 276, 310, 212, 240, 156, 98, 210, 200, 220, 180, 170]">
</kendo-chart-series-item>
<kendo-chart-series-item type="area" [data]="[165, 210, 287, 144, 190, 167, 212, 190, 210, 250, 260, 220]">
</kendo-chart-series-item>
<kendo-chart-series-item type="area" [data]="[56, 140, 195, 46, 123, 78, 95, 240, 250, 210, 280, 290, 320]">
</kendo-chart-series-item>
</kendo-chart-series>
</kendo-chart>
<hr>
<kendo-chart>
<kendo-chart-series>
<kendo-chart-series-item
type="donut" [data]="data"
categoryField="kind" field="share">
<kendo-chart-series-item-labels
[content]="labelContent"
color="#fff" background="none">
</kendo-chart-series-item-labels>
</kendo-chart-series-item>
</kendo-chart-series>
<kendo-chart-legend [visible]="false"></kendo-chart-legend>
</kendo-chart>
JavaScript
Add this in package.json dependencies
"private": true,
"dependencies": {
"@angular/animations": "~12.1.0-",
"@angular/common": "~12.1.0-",
"@angular/compiler": "~12.1.0-",
"@angular/core": "~12.1.0-",
"@angular/forms": "~12.1.0-",
"@angular/localize": "~12.1.0-",
"@angular/platform-browser": "~12.1.0-",
"@angular/platform-browser-dynamic": "~12.1.0-",
"@angular/router": "~12.1.0-",
"@progress/kendo-angular-buttons": "^7.0.0",
"@progress/kendo-angular-charts": "^6.0.0",
"@progress/kendo-angular-common": "^2.0.0",
"@progress/kendo-angular-dateinputs": "^6.0.0",
"@progress/kendo-angular-dialog": "^6.0.1",
"@progress/kendo-angular-dropdowns": "^6.0.0",
"@progress/kendo-angular-excel-export": "^4.0.0",
"@progress/kendo-angular-grid": "^6.0.1",
"@progress/kendo-angular-icons": "^1.0.0",
"@progress/kendo-angular-indicators": "^1.1.2",
"@progress/kendo-angular-inputs": "^8.0.0",
"@progress/kendo-angular-intl": "^3.0.0",
"@progress/kendo-angular-l10n": "^3.0.0",
"@progress/kendo-angular-label": "^3.0.0",
"@progress/kendo-angular-layout": "^6.5.0",
"@progress/kendo-angular-navigation": "^1.1.4",
"@progress/kendo-angular-notification": "^3.0.4",
"@progress/kendo-angular-pdf-export": "^3.0.0",
"@progress/kendo-angular-popup": "^4.0.3",
"@progress/kendo-angular-progressbar": "^2.0.3",
"@progress/kendo-angular-treeview": "^6.0.0",
"@progress/kendo-angular-upload": "^8.0.0",
"@progress/kendo-data-query": "^1.0.0",
"@progress/kendo-drawing": "^1.0.0",
"@progress/kendo-licensing": "^1.0.2",
"@progress/kendo-svg-icons": "^0.1.2",
"@progress/kendo-theme-default": "^5.0.0",
"rxjs": "~6.6.0",
"tslib": "^2.2.0",
"zone.js": "~0.11.4"
},
Now add the attached images
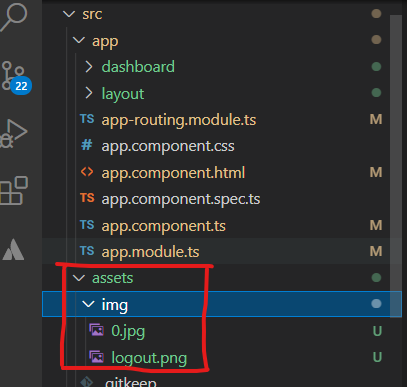
Now run the following command.
npm i
Now, please run the application using the 'npm start' command and check the result.
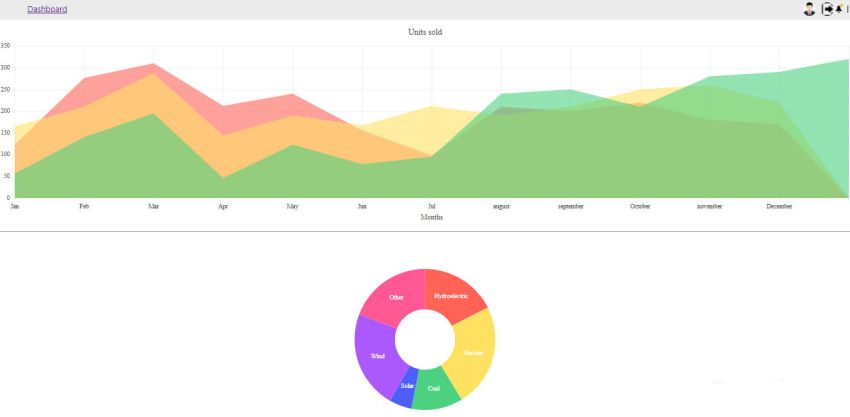