
September 21, 2018 11:48 by
Peter
What is Load Balancing ?
Distributing network traffic across multiple servers effectively is called Load Balancing.To meet high volumes, it generally requires adding more servers. Load Balancer routes the client requests to the servers in an optimized way and takes care that no server is overworked. If a new server is added to the group, It will start sending requests to this new server.
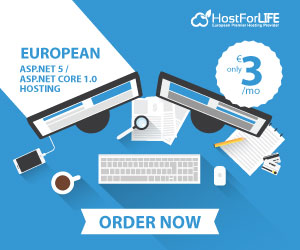
Load-Balancing Algorithms
Why did algorithms come into the picture ? Actually Load Balancer selects the server based on two factors,
- periodically pinging server to check its availability.
- defined algorithms - based on which load-balancer selects the server.
Algo 1 Least Connection (Default algorithm)
The least connection is the default algorithm to select server. Server which has the least number of active transactions is picked by Load Balancer to handle request. Load Balancer maintains records of transactions for each server.
Now discuss this in detail: Let's say all the servers have the same capacity and still some of the servers are overloaded as there may be the situation that the client stayed in those servers for longer duration and connected to other severs for shorter duration. Active connections on the server where client stayed longer will pile up and hence based on the least connection algorithm Load Balancer will route the request in the server with least active connections,
Weighted Least Connections
Now let's consider the above Least Connection algorithm with different server capacities (CPU/RAM etc.). You would definitely want to allocate more requests to the higher capacity server than the lower capacity servers. Weighted Least Connection is the solution.
Let's say there are 2 servers, server 1 and server 2 and server 2 has high configuration.With this algorithm Load balancer will allocate more requests to server 2 to utilize it better. Like Least Connection this also allocates request to the server withthe least number of active connections but the higher configuration server will handle more requests based on the right proportion defined during the Load Balancer setup. Weight proportion is calculated by the server capacity. Server 1 : Capacity X, Server 2 : Capacity : 10X the weight for each server would be 1:10 . There might be the scenario if you don't want to overload any of the servers for some reason, you may use this algorithm to give extra weight to other servers.
Round Robin
Round Robin is a vastly used and simple algorithm. Load Balancer distributes requests in the cyclic order irrespective of server inefficiencies. Both the Servers are ready to take request, suppose request comes and load-balancer routes to Server 1 then if a second request comes it will be routed to Server 2. The third and fourth will be routed to Server 1 and 2 respectively in a cyclic order. Even if one of the server has stronger configuration i.e. RAM, CPU etc. As per Round Robin algorithm Load-Balancer will follow the cyclic order.
Algo 4 Weighted Round Robin
Like Round Robin this is also cyclic but the higher configuration server will handle more requests.Rest it is same as Weighted Least connection algorithm i.e. weight would be defined during Load Balancer setup and high weight server will handle more requests.
i.e. if weight proportion to server 1 and server to is 1:10. first 10 requests will go to server and 11th request will go to server 1 and next 10 request will go to server 2 and so on.
Algo 5 IP Hash
This algorithm generates a hash key using client and server IP addresses which means this key would be assigned to client for subsequent requests which assure that the client is routed to the same server that it was using earlier.This algorithm can be used to achieve Session Persistence or Sticky Session.
Session Persistence
In the multiple server environment a user might experience losing cart items during navigation. Persistence session or sticky session is the culprit. As you know Http is a stateless protocol which means in subsequent requests it doesn't maintain any information about the user so to identify server uses client's ip or cookie to track users session. Sticky session is to make sure all the requests goes to the same server which has its user's session tracking information.
Layer 4 & Layer 7 Load Balancing
In Layer 4 Load Balancing , Load balancer decides the server on which it will redirect the requests on the basis of the IP addresses of the origin and destination servers (Network Layer : Layer 3) and the TCP port number of the applications (Transport Layer : Layer 4).
On the other hand Layer 7 Load Balancing decides routing on the basis of OSI Layers 5, 6, and 7 which together makes Http. To get an overview of OSI Model, please read my post .

September 20, 2018 11:12 by
Peter
The BackgroundWorker allows you to execute operations that take a long period of time such as database transaction or file downloads, asynchronously on a separate thread and allow the user interface to remain responsive.
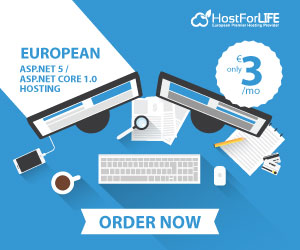
Start an Operation in the Background
First, you need to add an instance of the BackgroundWorker component to your application, if you use visual studio, just drag it from the toolbox to your application or you can create it manually as follow:
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
To start the operation in the background you have to call the RunWorkerAsync() method of the BackgroundWorker, when you call this method the BackgroundWroker starts the execution of the background operation by raising the DoWork event, the code in the DoWork event handler is executed on a separate thread.
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
//start the operation on another thread
private void btnStart_Click(object sender, EventArgs e)
{
backgroundWorker1.RunWokerAsync();
}
//DoWork event handler is executed on a separate thread
private void backgroundWorker1_DoWork(object sender, DoWorkeventArgs e)
{
//a long running operation
Thread.Sleep(5000);
}
You can send a parameter to the background operation using the RunWorkerAsync() method. You can receive this parameter by using the Argument property of the instance of DoWorkEventArgs in the DoWork event handler then you cast it to use it in the background operation.
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
//start the operation on another thread
private void btnStart_Click(object sender, EventArgs e)
{
backgroundWorker1.RunWokerAsync(2000);
}
//DoWork event handler is executed on a separate thread
private void backgroundWorker1_DoWork(object sender, DoWorkeventArgs e)
{
int input = (int)e.Argument;
//a long running operation
Thread.Sleep(input);
}
Reporting progress of a background operation using BackgroundWorker
By using the BackgroundWorker you have the ability to report progress updates of the executing operation. To use this options you must set the WorkerReportsProgress to true.
To start report progress you call ReportProgress() method and use it to pass a parameter that have the value of the percentage of the progress that have been completed. This method raises the BackgroundWorker.ProgressChanged event. In the event handler of the ProgressChanged you can recieve the value of the progress precentage on the main thread using the ProgressPercentage property of the instance of ProgressChangedEventArgs.
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
backgroundWorker1.WorkerReportsProgress = true;
//start the operation on another thread
private void btnStart_Click(object sender, EventArgs e)
{
backgroundWorker1.RunWokerAsync();
}
//DoWork event handler is executed on a separate thread
private void backgroundWorker1_DoWork(object sender, DoWorkeventArgs e)
{
//a long running operation
for (int i = 1; i < 11; i++)
{
Thread.Sleep(2000);
backgroundWorker1.ReportProgress(i*10);
}
}
private void backgroundWorker1_ProgressChanged(object sender, ProgressChangedEventArgs e)
{
progressBar1.value = e.ProgressPercentage;
}
Canceling a background operation using BackgroundWorker
With the BackgroundWorker you have the ability to cancel the background operation, to do this you first must set WorkerSupportsCancellation property to true.
The next step is to call the CancelAsync() method by doing so you set the CancellationPending property to true, by polling the CancellationPending property you can determine whether or not to cancel the background operation.
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
backgroundWorker1.WorkerSupportsCancellation = true;
//start the operation on another thread
private void btnStart_Click(object sender, EventArgs e)
{
backgroundWorker1.RunWokerAsync();
}
//a buttun to cancel the operation
private void btnCancel_Click(object sender, EventArgs e)
{
backgroundWorker1.CancelAsync();
}
//DoWork event handler is executed on a separate thread
private void backgroundWorker1_DoWork(object sender, DoWorkeventArgs e)
{
//a long running operation
for (int i = 1; i < 11; i++)
{
Thread.Sleep(2000);
backgroundWorker1.ReportProgress(i*10);
if(backgroundWorker1.CancellationPending)
{
e.cancel = true;
return;
}
}
}
Alert the user in the completion of the background operation
When the background operation completes, whether because the background operation is completed or canceled, the RunWorkerCompleted event is raised. You can alert the user to the completion by handling the RunWorkerCompleted event.
You can determine if the user canceled the operation by using the Cancelled property of the instance RunWorkerCompletedEventArgs.
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
//start the operation on another thread
private void btnStart_Click(object sender, EventArgs e)
{
backgroundWorker1.RunWokerAsync();
}
//a buttun to cancel the operation
private void btnCancel_Click(object sender, EventArgs e)
{
backgroundWorker1.CancelAsync();
}
//DoWork event handler is executed on a separate thread
private void backgroundWorker1_DoWork(object sender, DoWorkeventArgs e)
{
//a long running operation
for (int i = 1; i < 11; i++)
{
Thread.Sleep(2000);
backgroundWorker1.ReportProgress(i*10);
if (backgroundWorker1.CancellationPending)
{
e.Cancel = true;
return;
}
}
}
private void backgroundWorker1_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
if(e.Cancelled)
{
MessageBox.Show("Operation Cancelled");
}
else
{
MessageBox.Show("OperationCompleted");
}
}
Return a value from the background operation
You can return a value from the background operation that in the DoWork event handler using the Result property of the DoWorkEventArgs instance, and you can receive it in the RunWorkerCompleted event handler using the Result property of the RunWorkerCompletedEventArgs instance.
BackgroundWorker backgroundWorker1 = new BackgroundWorker();
//start the operation on another thread
private void btnStart_Click(object sender, EventArgs e)
{
backgroundWorker1.RunWokerAsync();
}
//DoWork event handler is executed on a separate thread
private void backgroundWorker1_DoWork(object sender, DoWorkeventArgs e)
{
//a long running operation here
Thread.Sleep(2000);
//return the value here
e.Result = 10;
}
private void backgroundWorker1_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
//recieve the return value here
int returnValue = (int)e.Result;
}

September 12, 2018 08:29 by
Peter
In this post we'll take a glance on inheritance of objects in Node.js. we'll learn to use utility module to achieve inheritance in Node.js. but confine mind that you simply will write plain JavaScript to attain inheritance in Node also.
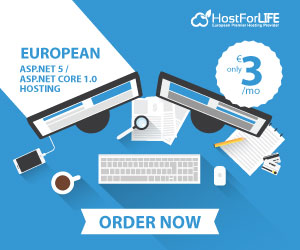
You'll use Object.create() to inherit one object to a different in Node.js also.
In this post we'll learn to use util module to achieve inheritance in Node.js. First, you should to import util module in your application.
var util= require(‘util’);
After importing util module, allow us to say you have got an object as below,
function Student()
{
this.name = "G Block";
this.age = 40;
};
Just for demonstration allow us to add function in object using prototype,
Student.prototype.Display= function(){
console.log(this.name + " is " + this.age + " years old");
};
Next we tend to we progressing to make ArtsStudent object which is able to inherit Student object.
function ArtsStudent()
{
ArtsStudent.super_.call(this);
this.subject = "music";
};
util.inherits(ArtsStudent,Student);
Second line of code in ArtsStudent object is very important,
ArtStudent.super_.call(this);
If you don’t call constructor of parent object as shown in above code snippet then on making an attempt to access properties of parent object will come undefined. In last line ArtStudent inherits Student using util.inherits() function ,
util.iherits(ArtsStudent,Student);
Next you can create instance of ArtsStudent and call function of parent object as below,
var a = new ArtsStudent();
a.Display();
Inheritance will be chained to any order. If you want you can inherit object from ArtsStudent as well. Inherited object will contain properties from both ArtsStudent and Student objects. So let us consider one more example,
function ScienceStudent()
{
ScienceStudent.super_.call(this);
this.lab = "Physics";
}
util.inherits(ScienceStudent,ArtsStudent);
var b = new ScienceStudent();
b.Display();
On this example ScienceStudent object inherits both Student and ArtsStudent objects. With this example, you can work with inheritance in Node.js using util module. I hope it works for you!
HostForLIFE.eu Node.js Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.


September 7, 2018 11:16 by
Peter
The below powershell cmdlets are used to create csv files by querying the SQL server.
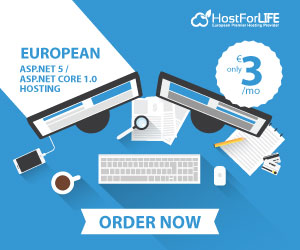
All the variables containing the server name and DB details:
#Variable to hold variable
$SQLServer = "XX.XX.XXX.XX"
$SQLDBName = "TestDB"
$uid ="domain\userID"
$pwd = "password123"
#SQL Query
$SqlQuery = "SELECT * from tableName;"
$SqlConnection = New-Object System.Data.SqlClient.SqlConnection
$SqlConnection.ConnectionString = "Server = $SQLServer; Database = $SQLDBName; Integrated Security = True;"
$SqlCmd = New-Object System.Data.SqlClient.SqlCommand
$SqlCmd.CommandText = $SqlQuery
$SqlCmd.Connection = $SqlConnection
$SqlAdapter = New-Object System.Data.SqlClient.SqlDataAdapter
$SqlAdapter.SelectCommand = $SqlCmd
#Creating Dataset
$DataSet = New-Object System.Data.DataSet
$SqlAdapter.Fill($DataSet)
$DataSet.Tables[0] | out-file "\\location\test.csv"
Note
When Intergrated Security ="true" is used, the cmdlets use current logged in user credentials - network credentails if Integrated Security ="false" we need to use the declared $uid and $pwd while establishing the connection.
The File is saved in the location or FTP path as test.csv
The SFTP is Secured FTP; the following powershell command helps to move any file to the SFTP location and it needs "Posh-SSH module" We need to install this module.https://github.com/darkoperator/Posh-SSH
The Powershell variable mentioned in the below code snippnet stores all the details of the SFTP or FTP server, where the files need to be moved.
#Declaring Variable
$sourceSFTPIP = "xx.xx.xxx.xx"
#IP address of the SFTP server
$LocalFilePath = "C:\test.csv";
$SFTPPath = ".\sharedFilePath\"
# folder location inside SFTP server
$secpasswd = ConvertTo - SecureString "password" - AsPlainText - Force
# the below object is used to key in the username and password automatically rather than promt the user to username and password
$mycreds = New - Object System.Management.Automation.PSCredential("username", $secpasswd)
# Module need to use SFTP Path
Install - Module - Name Posh - SSH# get sftp password this command can be used
if user need to feed in the user name and password at the time of running the script
# $credential = Get - Credential# Creating PS session to be used
$session = New - SFTPSession - ComputerName $sourceSFTPIP - Credential $mycreds - AcceptKey
# Move File using ps session
Set - SFTPFile - SessionId $session.SessionId - LocalFile $LocalFilePath - RemotePath $SFTPPath
$session.Disconnect()
We need to disconnect the session that was created to do this operation. Once it has run, the file that is present in sharepath is moved to the SFTP server location.