
August 25, 2023 08:22 by
Peter
ng-container
By enabling you to apply Angular directives without including additional items in the DOM (Document Object Model), the ng-container functions as a kind of transparent container. It is frequently employed when you wish to structure or conditionally include elements in your template.
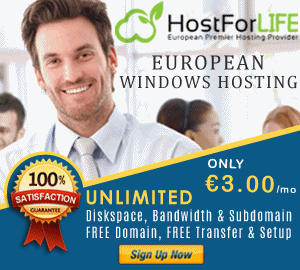
As an illustration, consider creating a product page. If the product is in stock, you want to display the product details; otherwise, you want to display a notification stating the product is unavailable.
<ng-container *ngIf="productAvailable; else outOfStockTemplate">
<div>Product Name: {{ productName }}</div>
<div>Price: {{ productPrice }}</div>
</ng-container>
<ng-template #outOfStockTemplate>
<div>This product is currently out of stock.</div>
</ng-template>
ng-template
The ng-template is like a template blueprint that doesn't get displayed immediately. It's used to define sections of your template that can be reused and conditionally rendered using structural directives.
Example: Let's say you're creating a list of blog posts. You want to show different styles for featured posts and regular posts.
<ul>
<ng-container *ngFor="let post of posts">
<ng-container *ngTemplateOutlet="post.featured ? featuredTemplate : regularTemplate; context: { post: post }"></ng-container>
</ng-container>
</ul>
<ng-template #featuredTemplate let-post>
<li class="featured">{{ post.title }}</li>
</ng-template>
<ng-template #regularTemplate let-post>
<li>{{ post.title }}</li>
</ng-template>
ng-content
The ng-content is used to allow external content to be included within the template of your component. It's ideal for making more adaptable components that can be used in a variety of situations. Consider designing a card component that can display a variety of content.
<div class="card">
<div class="card-header">
<ng-content select=".card-title"></ng-content>
</div>
<div class="card-content">
<ng-content></ng-content>
</div>
<div class="card-footer">
<ng-content select=".card-actions"></ng-content>
</div>
</div>
Now, when you use this component, you can provide custom content for each section:
<app-card>
<div class="card-title">Card Title</div>
<p>This is the content of the card.</p>
<div class="card-actions">
<button>Edit</button>
<button>Delete</button>
</div>
</app-card>
By combining these concepts, you can create components that are more dynamic and adaptable. ng-container, ng-template, and ng-content help you organize and structure your templates in a way that makes your code more readable and maintainable.

August 21, 2023 08:54 by
Peter
RxJS (Reactive Extensions for JavaScript) is a sophisticated tool used in Angular applications for handling asynchronous and event-based programming with observables. RxJS provides a diverse set of operators for manipulating, transforming, combining, and managing observables in a flexible and functional manner. These operators facilitate reactively working with data streams and asynchronous processes.
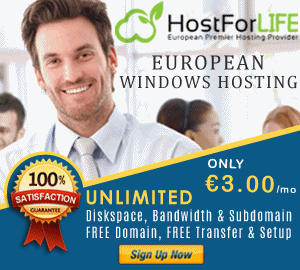
Here's a rundown of some of the most common RxJS operators and how they can be utilized in Angular:
map
The map operator is used to transform observable values into new ones. This is frequently used to do data transformations.
import { map } from 'rxjs/operators';
observable.pipe(
map(data => data * 2)
).subscribe(result => console.log(result));
filter
The filter operator is used to remove values from an observable depending on a criterion.
import { filter } from 'rxjs/operators';
observable.pipe(
filter(data => data > 5)
).subscribe(result => console.log(result));
mergeMap (flatMap)
The mergeMap operator is used to merge multiple observables into a single observable by applying a function to each emitted value and flattening the resulting observables.
import { mergeMap } from 'rxjs/operators';
observable.pipe(
mergeMap(data => anotherObservable(data))
).subscribe(result => console.log(result));
switchMap
The switchMap the operator is similar to mergeMap, but it switches to a new inner observable whenever a new value is emitted from the source observable, canceling the previous inner observable.
import { switchMap } from 'rxjs/operators';
observable.pipe(
switchMap(data => anotherObservable(data))
).subscribe(result => console.log(result));
debounceTime
The debounceTime operator delays emitted values for a specified amount of time and only emits the last value within that time frame.
import { debounceTime } from 'rxjs/operators';
inputObservable.pipe(
debounceTime(300)
).subscribe(value => console.log(value));
combineLatest
The combineLatest operator combines the latest values from multiple observables whenever any of the observables emit a new value.
import { combineLatest } from 'rxjs/operators';
combineLatest(observable1, observable2).subscribe(([value1, value2]) => {
console.log(value1, value2);
});
catchError
The catchError operator is used to handle errors emitted by an observable by providing an alternative observable or value.
import { catchError } from 'rxjs/operators';
import { of } from 'rxjs';
observable.pipe(
catchError(error => of('An error occurred: ' + error))
).subscribe(result => console.log(result));
These are just a few examples of the many RxJS operators available. Using RxJS operators effectively can help you manage complex asynchronous workflows, handle data transformations, and create more responsive and reactive Angular applications.
Happy Learning :)

August 8, 2023 08:27 by
Peter
In this article, we will go over the fundamentals of observable and subject. The distinction between them is further demonstrated through practical examples and the various types of subjects.
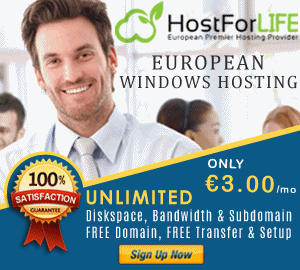
What exactly is Angular?
Angular is a popular open-source JavaScript web application framework. It was created by Google and is presently maintained by the Google Angular Team. Angular enables developers to create dynamic, single-page applications (SPAs) and offers a disciplined way to developing complicated online apps.
Visible in Angular
- Observables are a component of the Reactive Extensions for JavaScript (RxJS) library in Angular.
- Observables allow you to send messages between components of your program.
- Observables are a useful tool in reactive programming that is widely used to handle asynchronous actions and data streams.
- Observables allow you to subscribe to and get notifications when new data or events are emitted, allowing you to react in real-time to changes.
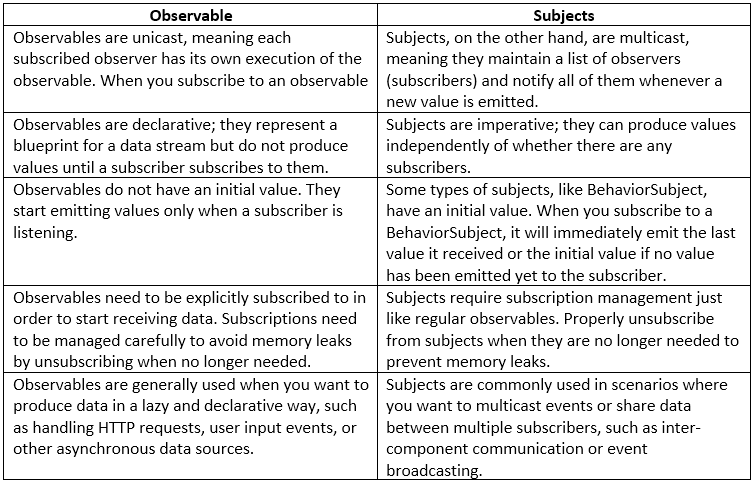
//------------------Observables are unicast-----------------
//observable
let observable = new Observable<number>(ele =>
ele.next(Math.random()))
//first subscriber
observable.subscribe(result => {
this.first_subscriber_observable = result;
console.log(result)
})
//second subscriber
observable.subscribe(result => {
this.second_subscriber_observable = result;
console.log(result)
})
//third subscriber
observable.subscribe(result => {
this.thrid_subscriber_observable = result;
console.log(result)
})
//--------------------------------------------------------
//------------------Subjects are multicast-----------------
//subject
let subject = new Subject<number>()
//first subscriber
subject.subscribe(result => {
this.first_subscriber_subject = result;
console.log(result)
})
//second subscriber
subject.subscribe(result => {
this.second_subscriber_subject = result;
console.log(result)
})
//third subscriber
subject.subscribe(result => {
this.third_subscriber_subject = result;
console.log(result)
})
subject.next(Math.random())
//--------------------------------------------------------
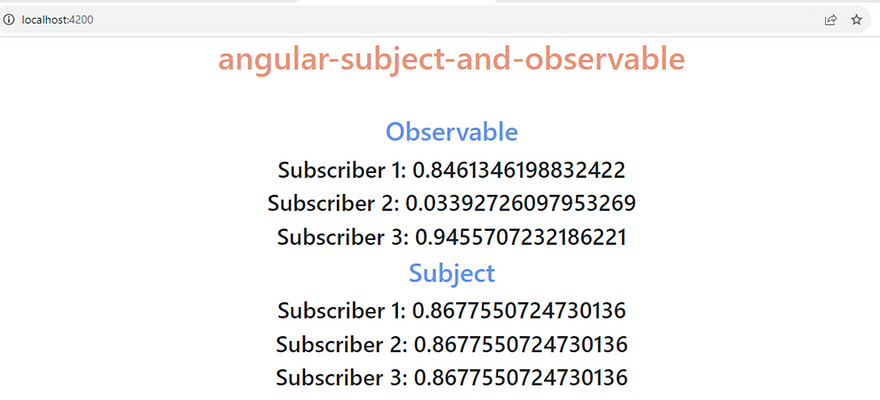
2. BehaviorSubject
The behavior subject is another type of subject in RxJS.
It has an initial value and will immediately emit the initial value to any subscriber as soon as they subscribe, even if no values have been emitted yet using the next() method.
After the initial value is emitted, it behaves like a regular Subject and notifies subscribers about new values emitted using next().
This is useful when you want to provide the last known value to new subscribers, such as the current state of an application or the latest data fetched from an API.
//----------Behavior Subject has default or last emitted value---------------
var behaviorSubject = new BehaviorSubject<number>(123)
behaviorSubject.subscribe(ele => {
this.first_subscriber_behaviorSubject = ele
console.log(`first subscriber ${ele}`)
})
behaviorSubject.next(456)
behaviorSubject.subscribe(ele => {
this.second_subscriber_behaviorSubject = ele
console.log(`second subscriber ${ele}`)
})
//--------------------------------------------------------------------------

3. ReplaySubject
The ReplaySubject is a subject that can buffer and replay a specific number of values to new subscribers.
When you create a ReplaySubject, you can specify the buffer size, which determines how many previous values should be replayed to new subscribers.
This is useful when you want to provide a history of values to new subscribers or when you need to cache values for later use.
//---------------Replay subject buffers old values not default one-----------
const replaySuject = new ReplaySubject(2) //If we we want to show only last 2 buffered value otherwise it will show all
replaySuject.next(111)
replaySuject.next(222)
replaySuject.next(333)
replaySuject.subscribe(e => {
console.log(`First Subscriber ${e}`)
this.first_subscriber_replaySubject.push(e);
})
//new values show to existing subsriber
replaySuject.next(444)
replaySuject.subscribe(e => {
console.log(`Second Subscriber ${e}`)
this.second_subscriber_replaySubject.push(e);
})
replaySuject.next(555)
//--------------------------------------------------------------------------
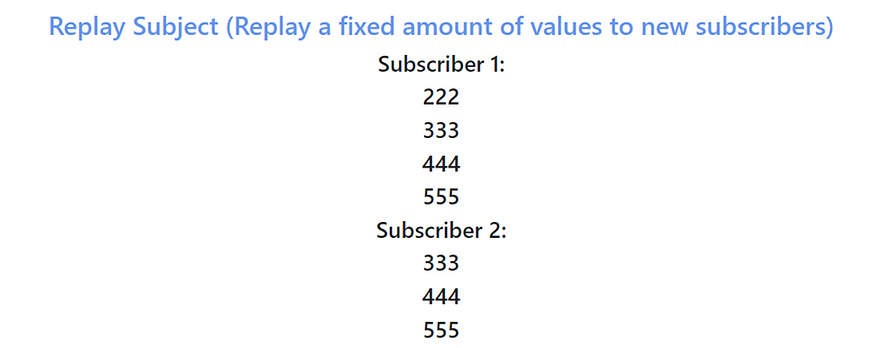
4. AsyncSubject
- The AsyncSubject is a subject that only emits the last value when it completes.
- It will not emit any values until the subject’s complete() method is called. When completed, it will emit the last value (if any) to subscribers.
- This is useful when you need to wait for an operation to complete before emitting a final value, such as waiting for an HTTP request to finish and emitting the response as a single value.
//---------------Async subject sends the latest value to subscribers when it's completed-----------
const asyncSubject = new AsyncSubject<number>();
asyncSubject.subscribe(e =>
{
console.log(`First Subscriber: ${e}`)
this.first_subscriber_asyncSubject=e;
});
asyncSubject.next(111);
asyncSubject.next(222);
asyncSubject.next(333);
asyncSubject.next(444);
asyncSubject.subscribe(e => {
console.log(`Second Subscriber: ${e}`)
this.second_subscriber_asyncSubject=e;
});
asyncSubject.next(555);
asyncSubject.complete();
//--------------------------------------------------------------------------


August 2, 2023 10:51 by
Peter
In this article, learn about ng-container, ng-template, and ng-content in angular
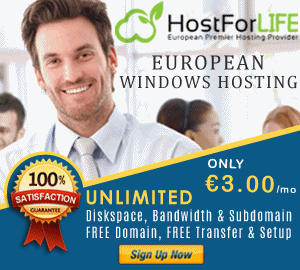
In the context of Angular, ng-container, ng-template, and ng-content are three different Angular directives that serve different purposes. Let's explore each one:
ng-container
The ng-container directive is a non-rendered element that is used to group and manage structural directives, such as *ngIf, *ngFor, etc. It acts as a placeholder or wrapper for these structural directives when they need to be applied to multiple elements or when you want to use them without adding an additional DOM element. The ng-container itself doesn't create any extra elements in the final DOM, making it a useful tool for organizing and applying structural directives without affecting the layout.
<ng-container *ngIf="condition">
<!-- Elements to show when the condition is true -->
</ng-container>
ng-template
The ng-template directive defines an Angular template that can be used to encapsulate HTML markup and Angular code that won't be rendered immediately. It acts as a template placeholder and can be referenced using the *ngTemplateOutlet directive or by using the <ng-container> element with the *ngTemplateOutlet attribute. ng-template is particularly useful when you want to reuse a piece of HTML code with its associated logic in multiple places.
<ng-template #myTemplate>
<!-- Template content here -->
</ng-template>
<ng-container *ngTemplateOutlet="myTemplate"></ng-container>
ng-content
The ng-content directive is used to create content projection slots within a component. It allows you to pass content from the parent component to the child component's template. When you define <ng-content></ng-content> in the child component's template, it acts as a placeholder for the content that will be projected into it from the parent component.
Example. Parent Component Template.
<app-child>
<!-- Content to project into the app-child component -->
</app-child>
As per the above snippet, the content is within the <app-child> tag.
Happy learning :)