We know Angular 15 is released on November 2022, so now in this article, we will see how to create an Angular 15 project. If you have already worked with angular previously you know, angular CLI helps us to build an angular application. Creating a new project in angular is now as simple as installing the Angular CLI and running the ng new command.
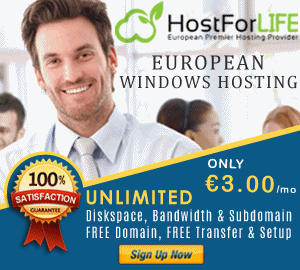
What is Angular CLI?
The Angular CLI helps us to create an Angular application and it uses Typescript, it creates an angular application with all the configuration files and the packages in one single command.
It helps us to add features (components, pipe, services, directives, etc.) to existing or new Angular applications.
How to create a new Angular 15 project
To create a new angular 15 project, first we need to set up the system environment
Installing Visual Studio Code
We need an editor to create the application so for that we need to install VS code. It is easy to install and helps us to create an angular application easily. You can download Visual Studio Code from the following link.
Node.Js
Node.js is the open source, cross-platform javascript run time environment.
Go to https://nodejs.org/en/
And install the LTS version
Install the Package Manager
To install Angular and its dependencies, we now need to install Node Package Manager (NPM).
NPM is the node.js package manager for JavaScript, which is automatically installed when we install node.js.
To see the version of NPM, run the below command on the Node.js command prompt like below
Node -v
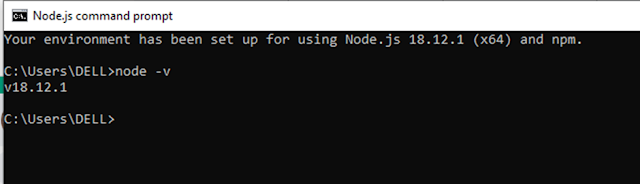
Go to the Node.js command prompt and install typescript using the below command
Npminstall –gTypescript
Angular CLI (command line interface)
It is a tool that allows us to create a project using the command line argument.
So below is the command to create the latest angular project
npminstall -g @angular/cli@latest
Once the above command runs successfully, now check the latest version of angular and run the below command
Ngversion
Since I was already working on angular 14, so for me only I need to run the Angular CLI command.
Below is the image when my angular version is v14,
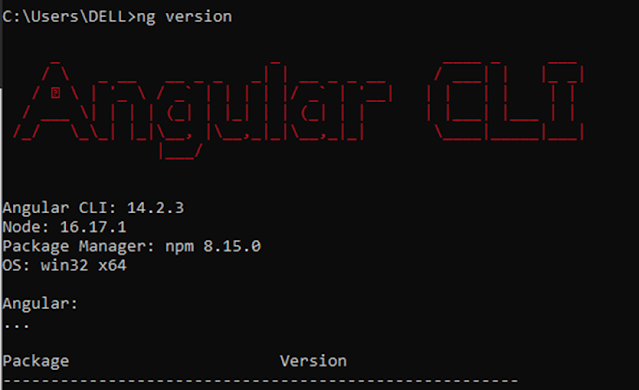
Now I ran below CLI command and then check the angular version like the below image
npminstall -g @angular/cli@latest
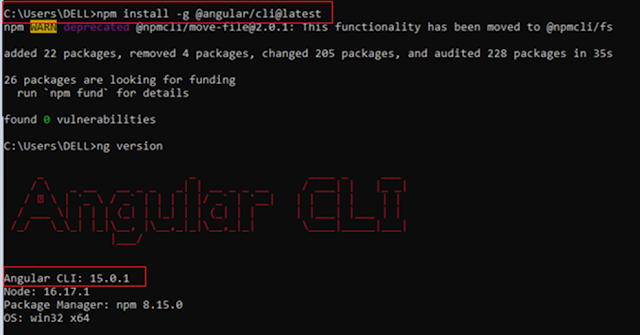
So this is the way we can upgrade the angular version to the latest version. You can also install the older version of Angular as shown below,
MDAngular8
cdAngular8
npmi @angular/[email protected]
Now to create an Angular 15 application run the below command
NgnewAngular15App
Once you run the above command it will ask to install routing like below, give Y and press enter
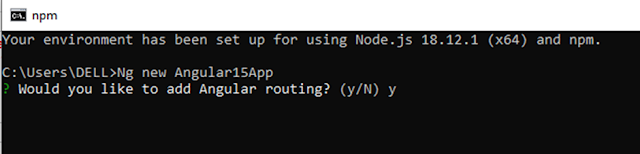
After that, it will ask to add CSS like the below,
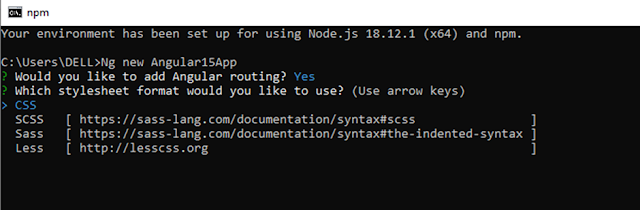
Once you press enter, it will take a few minutes to create the Angular 15 Application.
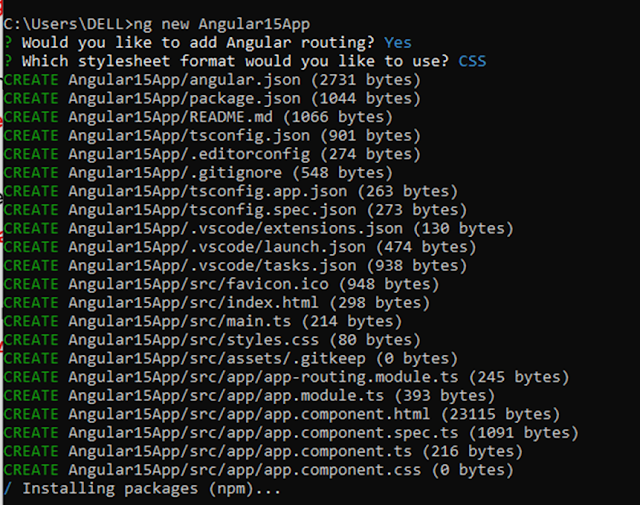
So from above, we can see, the angular 15 project creation is in progress.
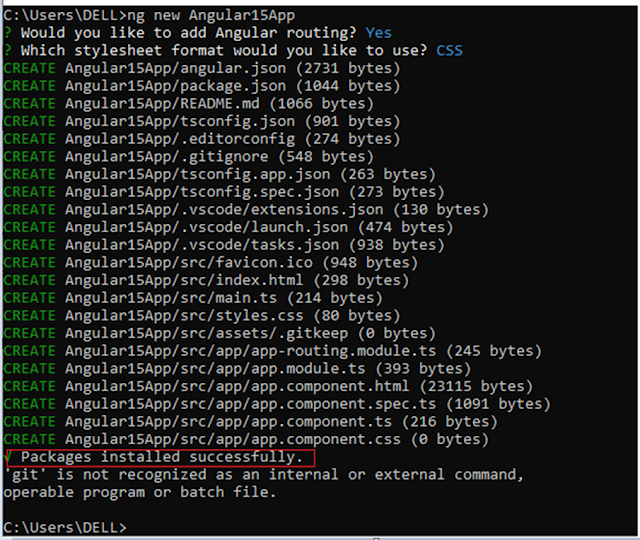
From the above image, we can see, the angular 15 projects was created successfully. After creating this project, now let's open the VS code, go File tab then select the folder, and go to the directory where we created the angular 15 project, from there, select the angular15 project folder. Once you will load the project, you will see all files in VS code.
Now run the ng serve –o command, it will open the angular15 project like below
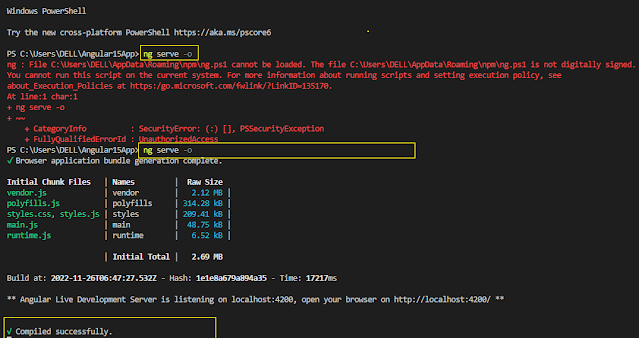
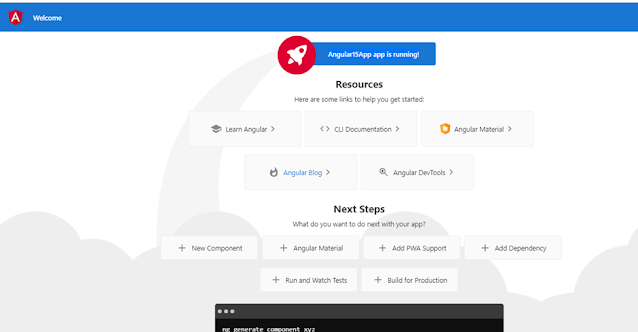
If you face an error like below then delete ng.ps1 file
PS C:\Users\DELL\Angular15App> ng serve -o
ng : File C:\Users\DELL\AppData\Roaming\npm\ng.ps1 cannot be loaded. The file C:\Users\DELL\AppData\Roaming\npm\ng.ps1 is not digitally signed.
You cannot run this script on the current system. For more information about running scripts and setting execution policies, see
about_Execution_Policies at https:/go.microsoft.com/fwlink/?LinkID=135170.
Then follow the below points then run ng serve –o again,
Delete ng.ps1 from the directory C:\Users\% usernam%\AppData\Roaming\npm\
Try to clear the npm cache directory C:\Users\%usernam%\AppData\ Roaming\npm-cache\
Note - Sometimes without clearing the cache also, the above error is resolved.
Now to verify that this application is created in Angular15, just go to the package.json file and see all the libraries are in v15 like below.
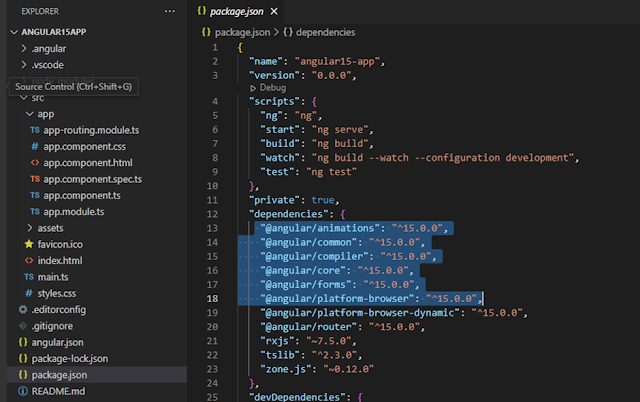
To build the application below is the command
ng build
To run the application below is the command
ng serve
after serving the application, go to the browser and enter the below URL, this is the default URL where the angular application run.
http://localhost:4200/