What is WCF?
WCF stands for ‘Windows Communication Foundation’. It is a Microsoft platform for building distributed and interoperable applications.
What is a distributed application?
A distributed application is an application where part of it runs on two or more computer nodes. Distributed applications are called ‘Connected systems’ also.
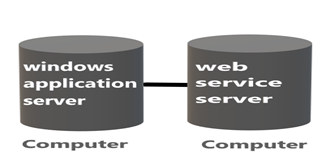
Now, let us see the above diagram where a Windows application is stored on one computer and the web service that it is consuming is running on another computer (which can be situated anywhere in the world). Well, this is a connected system.
Why build distributed applications?
Interdependency
An enterprise application may need to use the service provide by other enterprise, For example, an ecommerce application using Paytm for payments.
For better scalability
Scalability of an application implies the number of visitors an application can handle without degrading the performance. We can break down the application into different layers that run on different computers. Each of these computers will have its own memory and processor, which helps with improving the scalability of the application.
What is an interoperable application?
An application that can communicate with any other application that is built on any platform is called an interoperable application. Web Services can communicate with any application built on any platform, whereas .NET remoting service can be consumed only by another .NET application.
Why should we use WCF?
Without WCF,
Consider a situation where we have 2 clients and we need to implement a service for them.
The first client is using a Java application to interact with our services. This client wants a message to be in XML format and the protocol to be in HTTP. Without WCF, to satisfy the first client requirement, we will have to create an ASMX web service.
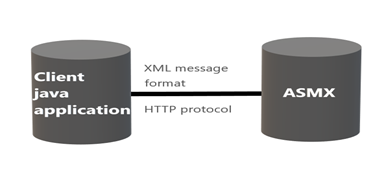
The second Client is using .NET and for better performance, this client wants a message format in binary format and protocol to be in TCP. Without WCF, to satisfy the second client requirement, we will create a .NET Remoting service.
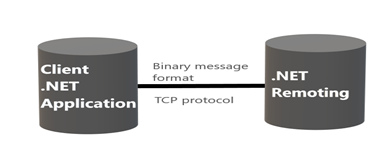
.NET remoting and ASMX are two different technologies, and have completely different programming models. Therefore, developers have to learn two different technologies, which is not only time and cost consuming but needs two people with expertise.
So to unite and bring all these communication technologies under one roof, Microsoft has come up with a single programming model that is called WCF. WCF is going to unify everything, such as .NET Remoting, IPC, MSMQ queue, TCP, Peer networking, and all other communication technologies we have.
With WCF
With WCF, for both the clients, we will implement only one service and to satisfy the requirement of different clients, we will configure different end points.
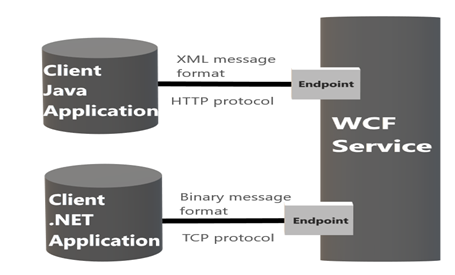
What Is WCF? Why Should We Use WCF?
Here, the first endpoint will transport a message in XML format over HTTP protocol and the second endpoint will transport the message in binary format over TCP protocol. Here, we don’t need to change the service code to configure these endpoints. Now, if we have a third client who needs a binary message over HTTP protocol, to satisfy that client requirement, all we need to do is to create a new endpoint.
So, we have a single service but then to satisfy the requirements of different clients, we are creating/configuring different endpoints.
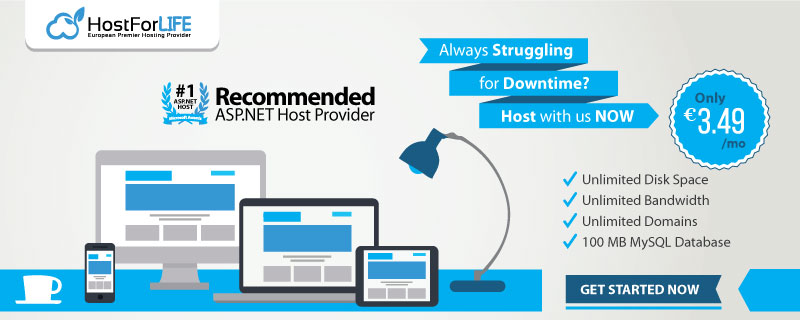