In this article, we'll look at how to use Node.js to create a web application. Let's take it one step at a time.
Step 1: Download NodeJS from the NodeJS website.
Download the Windows Installer from the Nodejs website. Check that you have the most recent version of NodeJs. It comes with the NPM package management. Install node js already and skip the preceding step.
Step 2: Ensure that Node and NPM are installed and that their PATHs are configured.
Check If you're not sure whether you installed everything correctly, use "Command Prompt" to double-check.
To verify the installation and confirm whether the correct version was installed,
Enter the following command to Check the node version.
Node --version or Node -v
Check the npm version, and run this command:
npm --version or npm -v
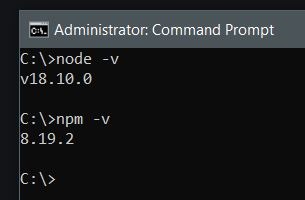
Step 3. Create a new project
You can create a project folder wherever you want on your computer and name it whatever you want.
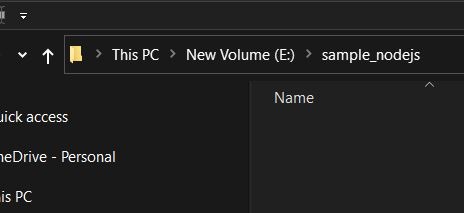
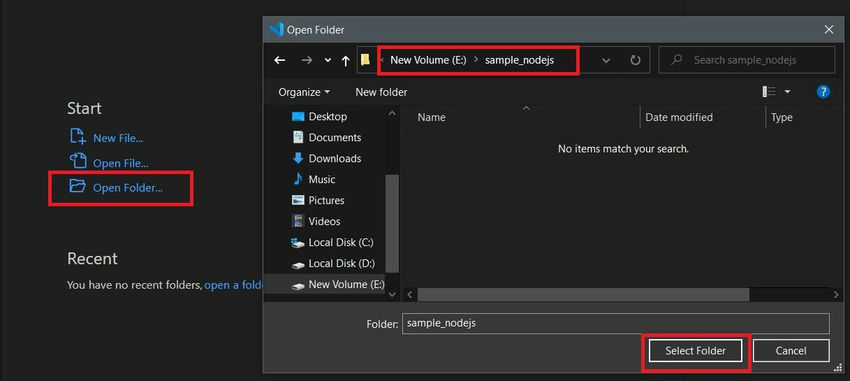
Step 4. Create a new js file
Create a .js File By using the .js file extension
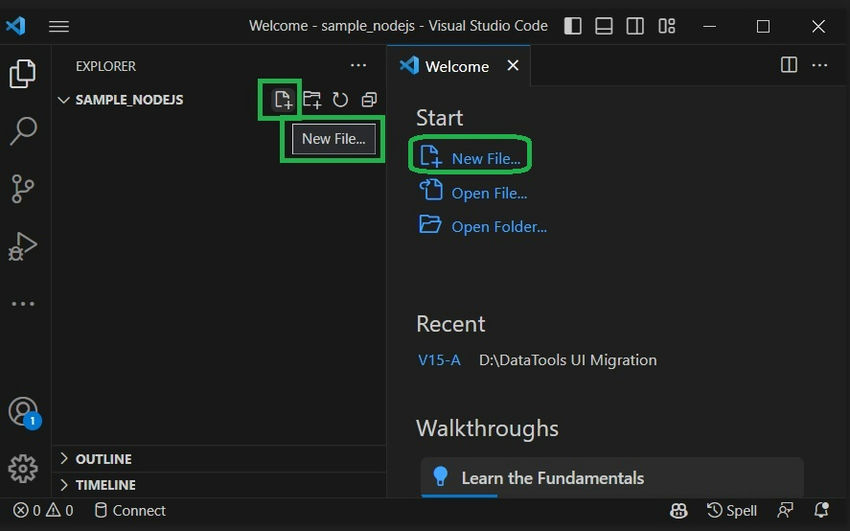
I Create an app.js file in the project folder. Create a simple string variable in app.js and send the contents of the string to the console: save the file.
var message= “Hi C# Corner”;
console.log(message);
Step 5. Run the App
It's simple to run app.js with Node.js. From a terminal, just type: Node .\app.js
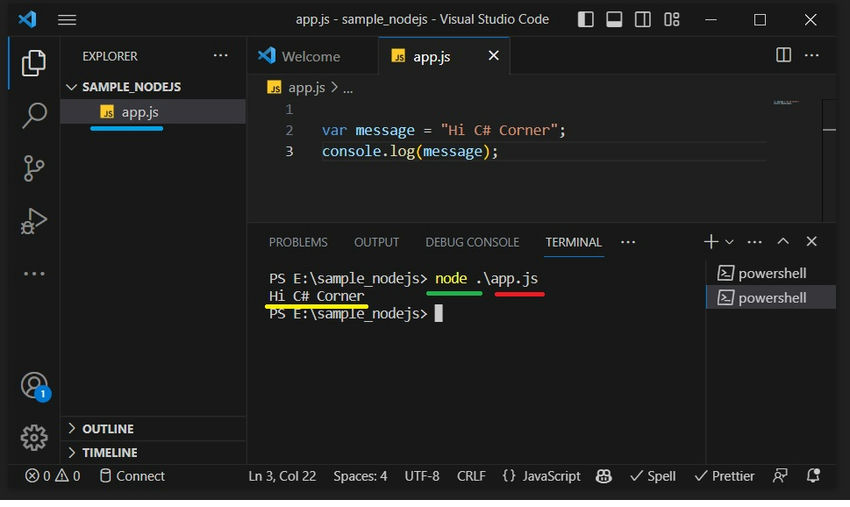
You can see the "Hi C# Corner" output to the terminal, and then Node.js returns
Step 6. Install NPM Packages
install npm package Go to the terminal and type npm init -y this command line, we highlight -y when we install in npm' npm init' Does that time ask a question: package name? We can give the package name as yourself. Otherwise, it takes the default name Project Folder Name. If entered, the command line npm init -y takes the saver name as the default.
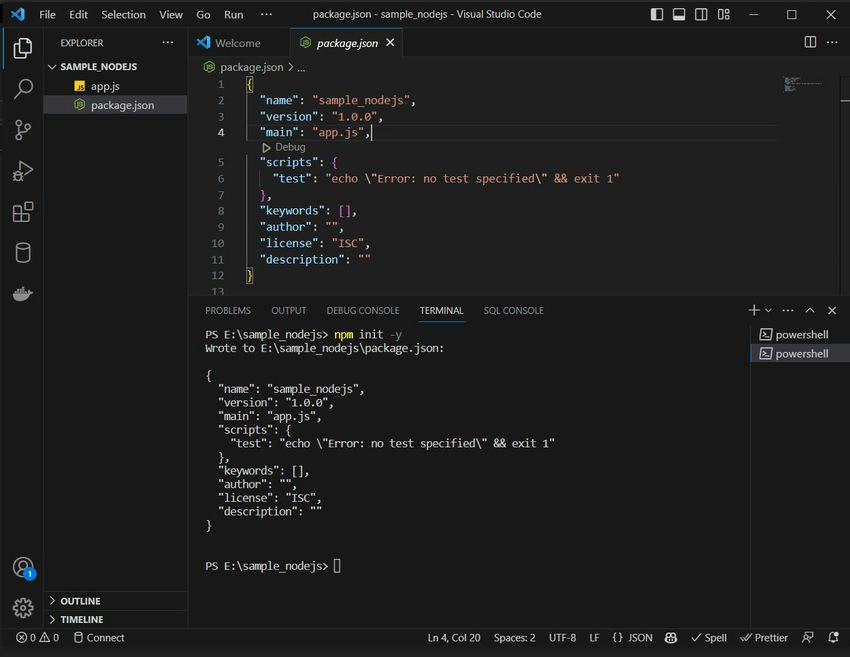
I'm going to run this Project in package.js, So I have to add a line in scripts at package.js "serve": "node app.js".
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"serve": "node app.js"
},
I'm going to run the application with this command npm run serve.
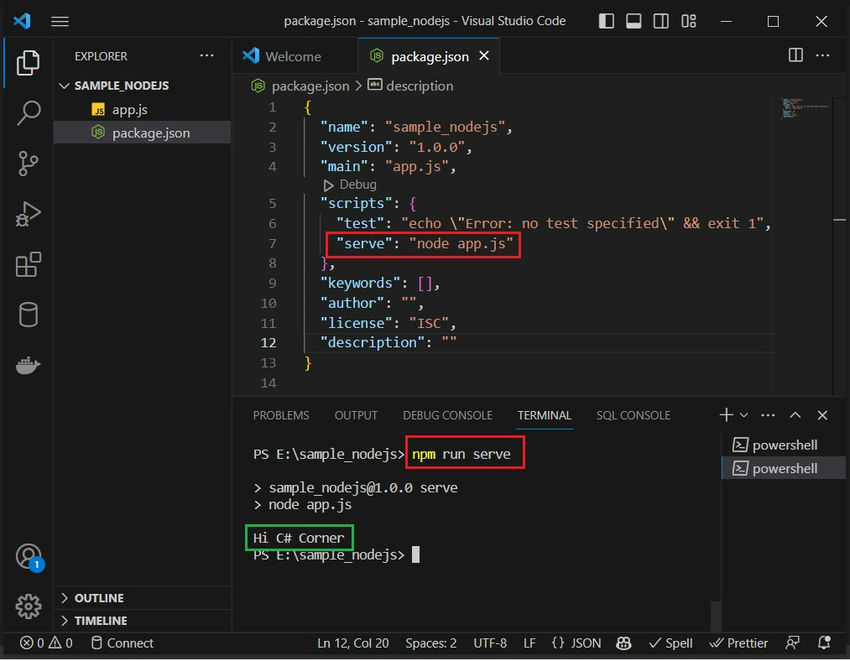
What Is Express JS?
Express is a node js web application framework that provides broad features for building web and mobile applications. It is used to build a single page, multipage, and hybrid web application.
Features of Express Framework
Allows to set up middlewares to respond to HTTP Requests.
Defines a routing table that is used to perform different actions based on HTTP Method and URL.
Allows to dynamically render HTML Pages based on passing arguments to templates
npm install express or npm i express
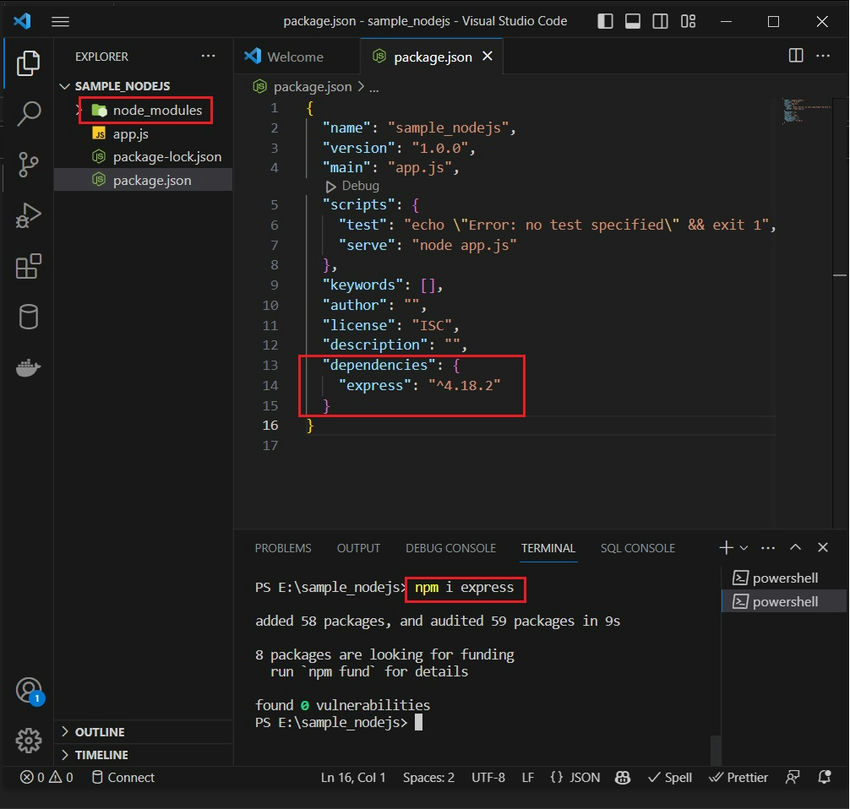
I recommend the nodemon package for Node. js-based applications by automatically restarting the node application when the file changes in the directory are detected.
nodemon will be installed globally on your system path.
npm install -g nodemon # or using yarn: yarn global add nodemon
Step 8. Write down the following code in the app.js file
const express = require("express");
const app = express();
const port = 3000;
//routes
app.get("/", (req, res) => {
res.send("HI C# Corner!");
});
app.listen(port, () => {
console.log(`My Sample Nodejs App Listening On Port ${port}`);
});
Following is a very basic Express app that starts a server and listens on port 3000 for the connection.
app.listen(port, () =>
{console.log(`My Sample Nodejs App Listening On Port ${port}`)
})
The app responds with "HI C# Corner!" for requests to the root URL (/) or route.
app.get('/', (req, res) => {
res.send('HI C# Corner!')
})
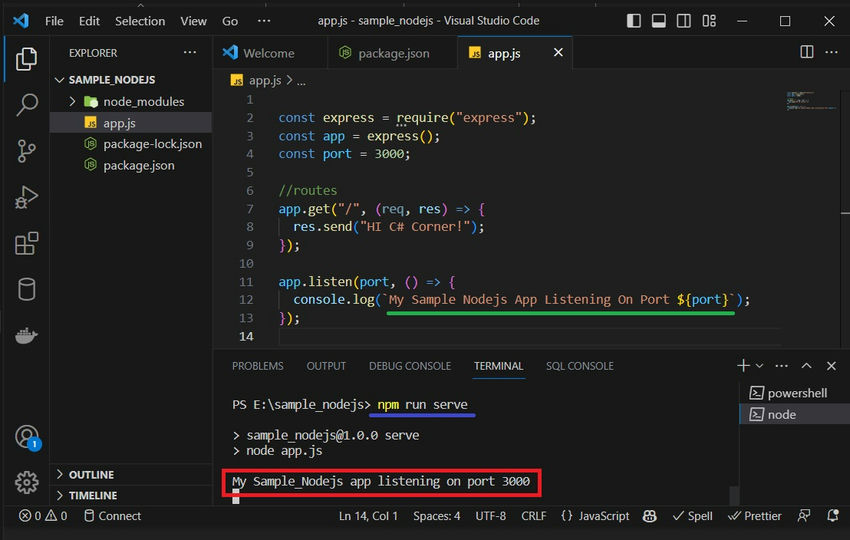
`npm run serve`
This app starts a server and listens on port 3000 for connections.
Step 9. Now you can see the output on your browser
Now go to http://localhost:3000/ in the browser, and you will see the following output:
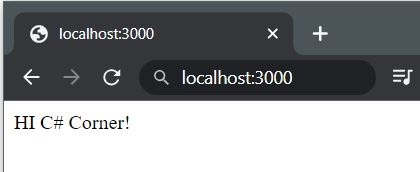
In this article, we will learn how to create a Node project and how we can develop a web application using Node.js. It is a runtime environment and library for running JavaScript code outside the browser. It must be installed on the system by downloading the software from the Node.js website and setting the path in the environment variables section.