If you've used Angular, you've probably run into the problem of rendering content in a situation other than the one in which it was defined. For instance, you might not want to include the dialog markup in the main component's design but still want to display a dialog or popover that covers a portion of the page. In the past, this needed laborious and error-prone workarounds, but now that Angular gateways are available, it's simpler than ever.
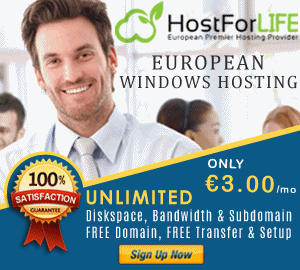
You can render a portion of your application in a different location in the DOM tree than where it is defined using Angular portals, also referred to as portal components. When you need to display content in a new context, this can help you design more adaptable and reusable components.
In this post, we'll define Angular portals, describe how they operate, and give usage examples.
An Angular portal can render content in a location other than the one where it was defined, in theory. To do this, a portal component that acts as a placeholder for the content must be created, and it must then be attached to the desired spot in the DOM tree using a portal outlet.
The portal component defines the syntax and behavior of the content you want to render and is a typical Angular component. The main distinction is that the portal component offers a place where the content will be added rather than rendering the material directly. The ng-content directive is used to define this slot.
A directive known as the portal outlet specifies where the portal will be presented. An Angular component or DOM element can serve as the outlet. One of the main advantages of using a portal outlet is that you can render the content in a different place in the DOM tree than where the portal component was declared. This can be helpful in situations where UI elements such as tooltips, modals, and others need to be rendered in a different context than where they were created.
How Do Angular Portals Work?
You must adhere to a few steps in order to use Angular portals,
Define the portal component in Step 1
The portal component that will act as a placeholder for the material you wish to render must first be created. Although it shouldn't render the content itself, this component should specify the markup and behavior of the content. Instead, it ought to have a space where the content can be added.
Here is an illustration of a straightforward message-display portal component.
import { Component } from '@angular/core';
@Component({
selector: 'app-message-portal',
template: `
<div class="message">
<ng-content></ng-content>
</div>
`,
})
export class MessagePortalComponent {}
The MessagePortalComponent uses the ng-content directive in this example to define a slot for the content. The content will be rendered inside this slot when the portal is connected to a portal output.
Step 2: Construct the Portal
The following step is to create an instance of the portal component that will be rendered. Typically, this is done in the component that will host the portal.
The ComponentPortal class from the @angular/cdk/portal package can be used to generate the portal instance. The portal component type is passed as an input to this class.
The following is an example of how to build a portal instance.
import { ComponentPortal } from '@angular/cdk/portal';
import { MessagePortalComponent } from './message-portal.component';
export class MyComponent {
private portal = new ComponentPortal(MessagePortalComponent);
}
In this example, the MyComponent component creates a MessagePortalComponent portal instance.
Step 3: Connect the Portal to the Portal Outlet
Once you've created a portal instance, you must connect it to a portal outlet in the desired DOM tree location. This may be accomplished by utilizing the PortalOutlet class from the @angular/cdk/portal package.
Here's an illustration of how to make a gateway outlet.
import { Component, ViewChild, AfterViewInit } from '@angular/core';
import { CdkPortalOutlet } from '@angular/cdk/portal';
@Component({
selector: 'app-my-component',
template: `
<div>
<h1>Welcome to my app</h1>
<ng-container #portalOutlet></ng-container>
</div>
`,
})
export class MyComponent implements AfterViewInit {
@ViewChild(CdkPortalOutlet)
portalOutlet: CdkPortalOutlet;
ngAfterViewInit() {
this.portalOutlet.attach(this.portal);
}
}
In this example, the CdkPortalOutlet directive is used by the MyComponent component to specify a portal outlet. This directive produces a placeholder for the portal's rendering.
The portal is associated with the portal output using the attach method in the ngAfterViewInit lifecycle hook. This method accepts as an argument the gateway instance established in step 2.
Step 4: Utilize the Portal
Finally, you can use the portal by populating it with content. This is accomplished by including the content to be rendered within the portal component tags.
Here's an example of how to show a message using the gateway.
<app-my-component>
<app-message-portal>
Hello, world!
</app-message-portal>
</app-my-component>
In this example, the app-my-component component hosts the portal, and the material to be rendered is contained within the app-message-portal tags.
Advantages of Using Angular Portals
Using Angular portals has various advantages.
Separation of concerns: You may isolate the markup and behavior of the content from the area where it will be rendered by building a portal component that provides a slot for the content.
Portals can be utilized in numerous situations, allowing you to create more flexible and reusable components.
Improved performance: Portals leverage the Angular Change Detection system to efficiently update content when it changes, resulting in better performance when compared to other approaches.
Improved accessibility- By incorporating a portal component that defines the semantics and accessibility of the material, you can ensure that the rendered content is accessible and adheres to best practices.
Use of Angular Portals Examples
Here are some examples of how Angular portals can be used in your applications:
Modals- Use a portal to display a modal dialog that overlays a portion of the page.
Tooltips- Use a portal to display a tooltip when the user hovers over an element.
Popovers- Render a popover that displays additional information when the user clicks on an element using a portal.
Use a gateway to render form fields based on user input or other conditions.
This article has discussed what Angular portals are, how they work, and provided examples of how to use them. You may increase the efficiency and accessibility of your applications by leveraging portals to create more flexible and reusable components. With these advantages, it's evident that Angular portals are a valuable tool that every Angular developer should have in their toolbox.