Enterprise resource planning (ERP) technologies are essential for optimizing business operations in the quickly changing digital landscape of today. Robust ERP solutions are becoming more and more necessary as businesses expand. With its cutting-edge functionality, improved security features, and speed gains, Angular is a formidable framework for creating cutting-edge ERP apps. This article will explore how Angular may boost ERP projects, emphasizing how it integrates with.NET Core online apps effortlessly and offers enhanced features, security advancements, and speed gains.
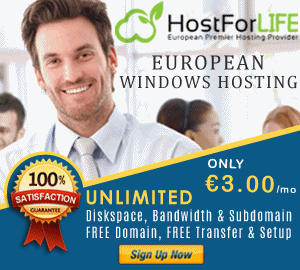
Advanced Functionalities
The extensive feature set of Angular enables developers to design complex ERP solutions that are suited to certain business requirements. Let's look at an actual instance of Angular integration in an inventory management ERP project.
// Angular component for inventory management
import { Component, OnInit } from '@angular/core';
import { InventoryService } from './inventory.service';
import { InventoryItem } from './inventory-item.model';
@Component({
selector: 'app-inventory',
templateUrl: './inventory.component.html',
styleUrls: ['./inventory.component.css']
})
export class InventoryComponent implements OnInit {
inventoryItems: InventoryItem[];
constructor(private inventoryService: InventoryService) { }
ngOnInit(): void {
this.loadInventory();
}
loadInventory(): void {
this.inventoryService.getInventoryItems()
.subscribe(items => this.inventoryItems = items);
}
}
Security Enhancements
Security is paramount in ERP systems handling sensitive business data. Angular provides robust security features such as built-in cross-site scripting (XSS) protection, strict contextual auto-escaping for templates, and a powerful HTTP client module for secure communication with backend services. Let's integrate authentication and authorization using Angular's router guards in our ERP project.
// Angular router guard for authentication
import { Injectable } from '@angular/core';
import { CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot, Router } from '@angular/router';
import { AuthService } from './auth.service';
@Injectable({
providedIn: 'root'
})
export class AuthGuard implements CanActivate {
constructor(private authService: AuthService, private router: Router) { }
canActivate(next: ActivatedRouteSnapshot, state: RouterStateSnapshot): boolean {
if (this.authService.isAuthenticated()) {
return true;
} else {
this.router.navigate(['/login']);
return false;
}
}
}
Performance Improvements
Angular's latest versions come with performance optimizations such as Ahead-of-Time (AOT) compilation, lazy loading modules, and tree-shaking for efficient bundle sizes. These optimizations ensure smooth user experiences, especially in large-scale ERP applications. Let's leverage Angular's performance improvements by lazy loading modules in our ERP project.
// Angular lazy loading module for improved performance
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
const routes: Routes = [
{
path: 'dashboard',
loadChildren: () => import('./dashboard/dashboard.module').then(m => m.DashboardModule)
},
{
path: 'inventory',
loadChildren: () => import('./inventory/inventory.module').then(m => m.InventoryModule)
},
// Other lazy-loaded modules
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Integration with .NET Core Web Application
Integrating Angular with a .NET Core web application is seamless, thanks to Angular's flexible architecture and .NET Core's robust backend capabilities. You can use ASP.NET Core Web API to provide data services to your Angular frontend. Here's a basic example of how you can set up a .NET Core Web API to serve data to our Angular ERP application.
// .NET Core Web API controller for inventory management
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
using System.Linq;
namespace ERP.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class InventoryController : ControllerBase
{
private readonly InventoryContext _context;
public InventoryController(InventoryContext context)
{
_context = context;
}
[HttpGet]
public ActionResult<IEnumerable<InventoryItem>> Get()
{
return _context.InventoryItems.ToList();
}
// Other CRUD operations
}
}
Conclusion
Because of its sophisticated ERP application development features, security upgrades, and speed advantages, Angular is a great option. Through the integration of Angular with.NET Core web apps, developers can fully utilize the capabilities of both platforms to provide safe and efficient ERP systems that are customized to fit the specific requirements of contemporary enterprises.