As we know, Google has released a new version of Angular i.e. Angular 15 recently. As per the expectation, the Angular team majorly focussed on the stability and performance improvement of the framework. So, in this article, we will discuss the new features introduced in Angular 15 and also discuss how to upgrade any existing applications to Angular 15. Angular 15 is released on November 16, 2022. As we already mentioned, this version mainly contains many features related to the performance and stability of the application.
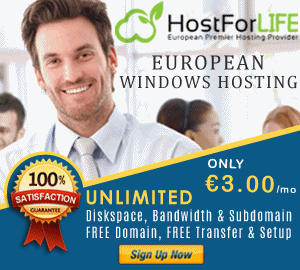
What is New in Angular 15?
The Client-Side Web-based application development process changed a lot once the Angular team introduced the new versions of Angular a few years back. All the current versions of Angular are based on TypeScript languages and are open-source-based frameworks. As developers, we can use develop any client-based SPA or Single-Page Application very easily with the help of Angular. On November 2022, Google released the new version of the Angular framework, i.e. Angular 15. Angular 15 is released with many stable standalone API supports which helps developers to bootstrap any web application by using a single component. As we know, the previous version Angular 14 is the most feature-based release by the Angular team and it also supports the latest TypeScript version i.e. TypeScript 4.7. As per the earlier release, the Angular team also declared that by removal of Angular’s Legacy Compiler and the rendering pipeline in the past releases, improves the performance of the application along with the developer’s experience.
Some of the key functionalities and features included in the Angular 15 are as follows –
Standalone Stable APIs
Directive Composition API
Improve Stack Traces for Debugging
Http with provideHttpClient
Stable Image Directives
Dependency Injection
Functional Route Guards
Automatic Imports in Language Service
Angular CLI improvements
CDK Based Listbox
With the latest release of Angular 15, we can use the single component concepts in Router, Angular Element, HttpClient, and other areas. So, if we use the Standalone Component, then it makes the development process much easier and also can provide us with an alternative way of using ngModule. So, the developer can develop any application without the ngModules which reduces the unnecessary complex design of any application.
Different Characteristics of Angular 15
So, while we discuss the Angular 15-related new characteristics or features, we should understand the changes done in Angular 15 compared to the previous versions of Angular Framework. Through this article, our primary focus is to discuss related the key features or characterize of the Angular frameworks. So, as a Framework, Angular 15 has many more features than those mentioned in this article. But, here, we only focus on some key or important features related to Angular 15 with this article.
01. Stable Standalone API
At the time of the Angular 14 version release, the Angular team officially announced that they are going to remove Angular’s legacy compiler and rendering pipeline implementation to improve the developer experience and performance. For the same purpose, in Angular 14 they release the Developer Preview version of Angular Standalone API. With the help of this, developers can develop any application without defining any Ng Modules. That, also helps us to create an app with less boilerplate code which improve the performance of the applications. Now, in Angular 15, Angular Standalone API is released as a stable version as it is now graduated from the preview version. The standalone API is not fully functional in many areas of angular framework like HttpClient, Angular Router, Elements, and many more. Now, in Angular 15, we can directly bootstrapping any application through a single component with the help of a Standalone API.
import { bootstrapApplication } from '@angular/platform-browser';
import { Component } from '@angular/core';
import { AppComponent } from'./app.component';
@Component({
standalone: true,
selector: 'sample-component',
imports: [ AppComponent ],
template: `<h1>Welcome to Angular 15</h1>`,
})
export class SampleComponent {
// component logic
}
bootstrapApplication(SampleComponent);
As per the above example, we can use the reference of the Standalone directives or components or pipes under the import function. If we mark the component as a “standalone: true” the under the component metadata, then we do not need to declare or use that component within NgModules. Also, if we require to use any specific module within the standalone component, then we can use the import function to import that module directly within that function like “import : [module_name]”.
02. Directive Composition API
Directive Composition API is one of the new features introduced in the Angular 15 release. This feature is introduced in Angular 15 due to the popular feature request on GitHub by the developer community. With the help of this, we can reuse our code. Directive Composition API helps developers to use host elements with the existing directives and in this way, it helps us to implement code reusability functionality within the application. This feature also helps developer to save time during the development process. While we use directive composition API, that particular directive acts as a Standalone directive. It needs to be remembered that all the functionality related to the directory composition API is possible with the help of the Angular compiler.
import { Component } from '@angular/core';
@Component({
selector: 'app-menu',
hostDirectives: [
HasColor,
{
directive: CdkMenu,
inputs: ['cdkMenuDisabled: disabled'],
outputs: ['cdkMenuClosed: closed']
}
]
})
class AppMenu {
}
In the above example, we can see AppMenu Component is created with the help of two different hostDirectives i.e. HasColor and CdkMenu. Due to this technical enhancement in Angular 15, we can reuse the properties of any existing directives. Here as per the example, the AppMenu component will inherit all the input, and output properties along with the business logic of HasColor Directive and input, and for CdkMenu, it will inherit the input properties and the business logic.
03. Image Directive
The concept of Image Directive (or NgOptimized Image) is first introduced in Angular 14 along with Chrome Aurora to improve the performance of image loading. After that release, developers experience around 75% of improvement in the LCP. Now, in Angular 15, the same Image directives become much more stable along with some new features. Now, in this directive, we can take benefit of the automatic generation functionality of the SRC set. It helps us to upload and return the appropriate size of the images whenever we require. Due to this, the download time of the image is reduced. Also, we can use another feature called Fill Mode (currently it is released as an experimental mode). With the help of this, we do not need to declare the image dimensions. It is quite helpful when we try to migrate the CSS-based background image to use this directive or when we don’t know the exact dimension of the image.
This directive, i.e. NgOptimized can be used directly either in the NgModule.
import { NgOptimizedImage } from '@angular/common';
// Include it in the necessary NgModule
@NgModule({
imports: [NgOptimizedImage],
})
class AppModule {}
Also, we can use it within the Angular component directly. While we need to use the Angular Image directives within a component, we need to replace the src attribute of the image tag with ngSrc, so that the image directive can prioritize the attribute to optimize the speed of the LCP images.
import { NgOptimizedImage } from '@angular/common';
@Component({
standalone: true
imports: [NgOptimizedImage],
})
class MyComponent {}
04. Functional Route Concept
The angular team introduced a new concept in the implementation process of route guards in Angular 15. In the earlier version, we normally use the class-based implementation for the route guards. But now in Angular 15, it is marked as @deprecated as per the Angular documentation. A new approach called Functional Route Guards has been introduced in place of the class-based approach. By using the new approach, we need to define the functions in place of the class-based concept while defining route guards and it helps us to implement route guards in a much more efficient and streamlined way. For this purpose, we can use the inject function from the @angular/core package which helps us with the dependency injection. This implementation helps us to create a reusable and composable function that we can pass as an argument within the Angular Router’s canActivate or canActivateChild functions.
As per the Angular documentation, the benefits of the functional route guards are as follows –
We can define the route guards and their resolvers as a JavaScript functions as well.
It reduced the boilerplate code as functional route guards do not require to define different guard class definitions.
Functional route guards increase flexibility as they can be defined as an inline function also.
Performance wise functional route guards are faster as compared to class-based guards. It is because it does not require creating or initiating the separate class objects instance.
05. Http with provideHttpClient
One of the new improvements in Angular 15 is that we can now use the provideHttpClient option to use HttpClient in place of using HttpClientModule. As we know that in Angular 15, modules are becoming optional now. So, in that case, taking the support of the HTTP module is a stress. So, now we can use use the provideHttpClient() which offers the support of the HttpClient.
06. Language Service
Now, in Angular 15, we can import such components directly with the help of language services that are not either mentioned as a standalone component or within the NgModules. Now angular in-language support service provides an option called Quick Fix. Through this, we can write down our angular code much more confidently and we can use this automatic import component and its fixes in the template.
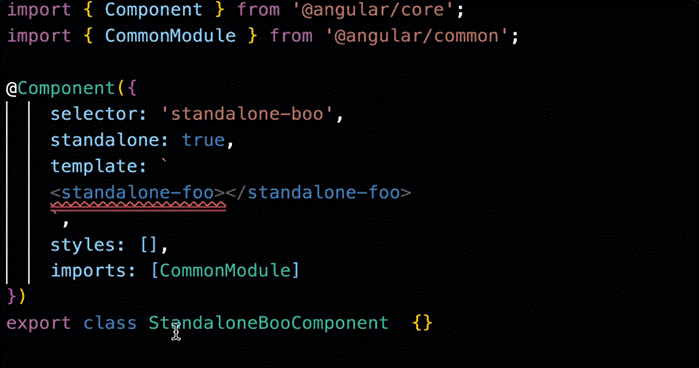
07. Dependency Injection
In Angular 15, there are some changes done in the case of Dependency Injection. They are –
- In the case of the @Injectable() decorator, it is no more supported with the provided: _NgModule syntax. In this case, we need to use providedIn : “root”.
- We can also use the NgModule.providers if we want to limit the dependency injection to a single NgModule.
- Also, the syntax provided: ‘any’ is also deprecated in Angular 15.
08. Improved Stack Trace
Now, in Angular 15 we can take benefit of the better Stack Traces which help us to identify any error or the location of any error. This feature is developers for to debug any issue during the development process. Now, during the execution of any application if an error occurred, then Angular will show the development code along with the library or packages which it calls. In the earlier version, developers faced difficulties to understand the error snippers as –
- Most of the error message-related information is generated from third-party dependencies like Angular framework, zone.js, and Rx.js.
- Also, the error message does not provide any information about which line or block code encountered this error.
But now, after a long time of collaboration between the Angular and Google Chrome DevTool team, they integrate those third-party libraries with the help of node_modules, zone.js, etc to raise proper error messages by using stack trace. So, now through this error message information, the developer can easily identify the exact code block of that error and fix it quickly.
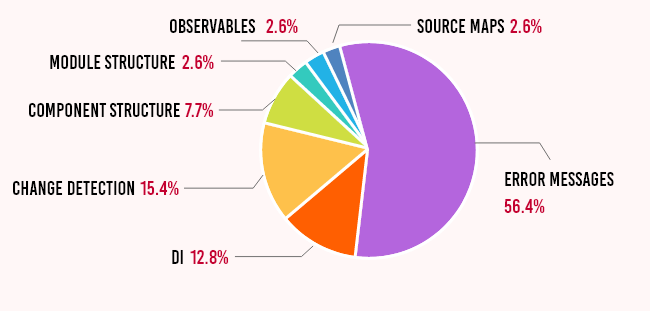
09. MDC-based Components
With the release of Angular 15, we can use the much more stable Angular material-based components. Now, most of the Angular material components s designed in such a way that we can use them in web applications as well. Most of the changes regarding these components are based on DOM and CSS related. Now, in Angular 15, many Angular Material components related to old implementations have been deprecated or moved to the legacy section. So, if we still want to use them then we need to use the “legacy” import option for that.
import {MatLegacyButtonModule} from '@angular/material/legacy-button';
10. Improved ES-Build Supports
In the Angular 14 release, the Angular team announced one experimental functionality support called esBuild support to enable the faster build time of the application. Now, with Angular 15, the same esBuild support is extended with some new functionality like SVG template, and file replacement. Now, it also supports the ng build –watch command. The command for upgrading the angular.json builder is:-
"builder": "@angular-devkit/build-angular:browser-esbuild",
How to Upgrade to Angular 15?
So, in the above section, we have discussed the new functionality and features of Angular 15. Now, let's discuss how we can upgrade any existing angular application to the Angular 15 version. Before going to upgrade to Angular 15, we need to know some dependencies related to Angular 15 like –
- Angular 15 has provided support for node.js version 14.20.x, 16.13.x and 18.10.x. And it terminates the support related to version 14.[15-19].x and 16[10-12].x.
- Angular 15 now only support TypeScript version 4.8 or older than that.
So, we can use the below commands to update Angular 14 to Angular 15 –
ng update @angular/cli @angular/core
Conclusion
This article discusses some key functionalities of Angular 15. As Angular team is majorly focusing on the performance improvement of the framework for this version like server-side rendering, code reusability, etc. This type of improvement always helps the easier learning curve more simplified. So, let's upgrade the application to the Angular 15 version and use its new features and functionality of it.