In this tutorial, I will show you how to create a 'Lightbox' effect for your Joomla website using the plugin YOOeffects. Using a Lightbox is a great way to show off your images on your website, as a slideshow or for individual pictures. Videos can also be shown using the Lightbox javascript - both from your own website or streamed from another site (such as YouTube).
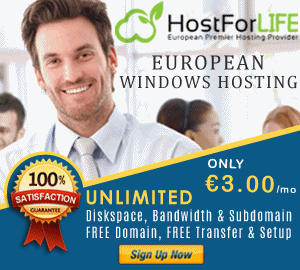
What is Lightbox?
Lightbox is a simple javascript that creates an effect that when a user clicks on the image, it pops up, is magnified using a smooth animation and is set on a darkened background. Lightbox is triggered by a 'rel' attribute in the HTML which is used in the <a> tag which surrounds the target image. The Lightbox script is great because it works on all browsers and eliminates the need for Flash animation. Flash although popular is notoriously bad for SEO as Google's bots struggle to read the data within the flash animation.
Here is an example of the lightbox:
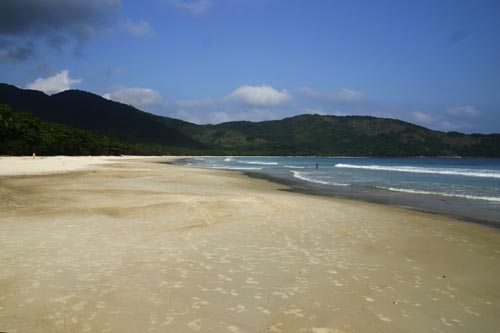
Lightbox effect for Images
If the YOOEffects plugin has been successfully installed, it's time to see how to write the code to ensure the plugin works for your chosen images. The code looks a little daunting, but it is really quite simple once you break it down, especially if you are used to using HTML.
The following is an images is the Lopes Mendes beach, on Ihla Grande, Brazil. If you click on the image you will see the lightbox effect.
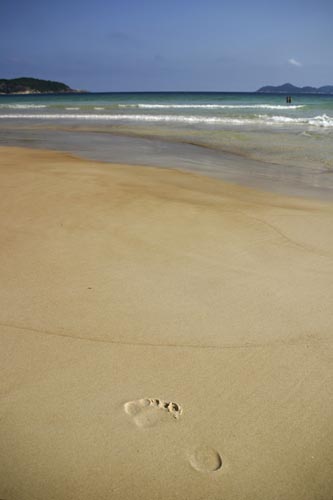
The code should be entered into the article that you are wanting to display the image in. It is key not to copy and paste this code as text into your article, but click on the 'HTML' editor within your chosen editor then paste it.
<a href="/images/stories/lopes-mendes-beach.jpg"
title="Lopes Mendes Beach" rel="shadowbox;width=500;height=333"
alt="Lopes Mendes Beach" title="Ihla Grande" width="250" height="165" />
You will notice i have changed the title of the image for each part of the code. This is to show you that the 'Lopes Mendes Beach' is the description that appears when the lightbox is engaged, and Ihla Grande appears when you hover over the image in its present state. It can be a useful way of providing more information about your chosen image to your users.
The second part of the code determines the size of the thumbnail that is displayed to click on, be sure to keep the same proportions, to ensure the image is not distorted!
Lightbox Image Slideshow
Having slideshows of images is a clean, easy and convenient way to display images and this can be done using a Lightbox effect too.
The code you would insert to create a slideshow of images is the following:
title="Lightbox Slideshow"rel="lightbox[group];width=500;height=333">
Click here to see a lightbox slideshow.
title="butterfly photo"rel="lightbox[group];width=500;height=333">
title="waterfall photo"rel="lightbox[group];width=333;height=500">
The best way to construct a slideshow is to add the "rel=lightbox" attribute to your links first so that they open in lightbox. Once you have done this, you need to remove the names of the links so they become invisible. The final step is to add in [group] in the rel attribute. You can name this anything you want, but all of the links must have to same name, so they appear in the same slideshow. witYou can see how i have done this in the code above. You can add in as many images as you like, and you can do this with videos too, not just images. The code is very simple, but it can be easy to make mistakes, so check through your work carefully if it does not work first time!
Display Videos using Lightbox
The great thing about this plugin is that you can use the same script to show videos on your website. It is far easier for users to focus on a video if the rest of the screen is darkened, so this plugin can be used to great effect. Importantly, this plugin means that people can watch videos hosted on other sites within your own - so there is no reason for visitors to leave you site.
The code is very similar and is as follows:
<a href="http://www.youtube.com/v/jtK6qITij5k&hl=en&autoplay=1"
title="Open Source Content Management Systems"
rel="shadowbox;width=425;height=355">Joomla and Google's Summer of Code</a>
You can play any type of video file as long as your browser can, so this tool is very useful. The key is to ensure you have the linked to the video directly - make sure you are not just linking to the page, otherwise lightbox will not play the video.
HostForLIFE.eu Joomla 3.4 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
