Entity Framework (EF) is Object Relational Mapper(ORM) for .NET. In simple words, it is a collection of libraries that connect the objects in code with the schema. EF is a bit different than other ORMs. It has a mapping layer between domain classes and schema. It is Microsoft’s recommended data access technology. Of course, data access can be achieved by writing your own DAL (Data Access Layer) using ADO.NET or by using third-party mappers like Dapper but EF is a persistent and open source framework supported by Microsoft. It is possible to perform full CRUD (Create, Read, Update, Delete) operations. EF can help in the consistency of task, developer's productivity, and LINQ syntax helps to use any RDBMS (regardless of SQL writing style ie: Oracle or SQL Server). Actually, the purpose of EF is to let developers focus on the domain, not on the database.
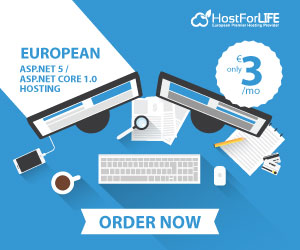
There are mainly three workflows for Entity Framework.
Database-first approach
In this workflow, a database is created first with all tables and related objects (i.e, stored procedures). Entity Framework creates domain classes using the Entity Data Model Wizard. In this approach, most of the efforts are focused on designing database structures. It is a traditional approach which many developers are doing for years.
This approach suites when different teams like DBA design the database and programmers are supposed to integrate the database with an application or when requirements/goal are not clear and changes in the database are incremental. Or, if you have already one long-lasting stable database and you have a scenario to use an existing database – well, it is still possible to convert an existing database to code-first approach (reverse engineering is challenging in some scenarios but it is doable).
Advantages
- You can start initial development soon.
- Existing databases can be utilized easily
- If there are requirements like to have stored procedures, triggers, and table columns in a certain order (this feature is added in EF 2.1 now) then this workflow has an advantage over code first
- If you need GUI for designing. For example: In SQL Server you can generate database diagrams and create or update objects from there.
Disadvantages
- The sync of changes is not easy. For example, you make changes in schema or table which you need to sync on your different working environments. You would need an external tool or do it manually.
- If you are interested in version control like Git or SVN, then you cannot do it with database first. EDMX has no history of changes.
- Sometimes, changes in structures of the database are complicated to update EDMX
- Classes generated in this approach are auto-generated
Code-first approach
In this approach, we first create domain classes and then EF generates database tables. It is possible to create POCO classes (business objects) and you have complete control of it. You can write classes with properties which generate tables with columns using Migration process. Along with migration, the History table is also generated which can give you a version control feature.
This is a good approach if you are the programmer and you are the one who designs the database as well. This approach is useful if changes in the database are more, the application is scalable and needs tracking as well.
Advantages
The database sync is easy for environments using migration. It is a really needed feature which makes it possible to upgrade or downgrade any change/commit.
You get control on the code so you can validate fields from classes as well
Disadvantages
No GUI so you need programming experience
We do not have real full control on the database, of course, EF is releasing a new version with many new features but let's say: It is not possible to create or remove trigger or stored procedures from EF until we use SQL in Context class.
Model-first approach
In simple words, this approach is based on the GUI. All you need is to create entities and relationships on EDMX design surface so it is like a UML diagram. From the Entity Data Model wizard, you can choose the Designer model and create your entities all in GUI. When you are done with your design, you can choose “Generate Database from model” to auto-generate domain classes and database. This approach can be useful if a data structure is big and you need very little control on the database. For example, if you need triggers or stored procedures or custom business logic on fields of entities, then you should consider either database first or code first.
Advantages
The visual design interface can help to create entities easily in a short time
Programming experience not needed
Disadvantages
Auto-generated code is a limitation here
Little control on database
Sometimes, EMDX auto-generated scripts still need modification which needs good SQL level of expertise to get a workaround.
How Entity Framework Core is different than Entity Framework?
Entity Framework Core(EF Core) is lightweight (collection of composable API), cross-platform (Linux, Windows, UWP) and extensible (with modern software practices). EF Core works with .NET Core but with .NET Core, it is recommended to use EF Core. Of course, all the features are not released yet and there are many features which are missing. The EDMX/Designer is missing in EF Core so model first is not possible with EF Core without using third party tools. EF Core supports both, database-first and code-first, approaches. Database first with EF Core is like; it reverse engineers the existing database which later can be used as code first. So, if you want to say strictly then database first is not fully supported. EF Core supports not only RDBMS(SQL Server, Oracle, etc.) but also non-relational stores and in-memory databases which can be used for unit testing.
Conclusion
In my opinion about workflows, there is no good or bad approach but our specific requirement helps to decide which one to choose. But, whatever flow you choose - never mix different workflows in one project.