This article demonstrates how to share data between components using @Input(), @Output(), and @ViewChild().
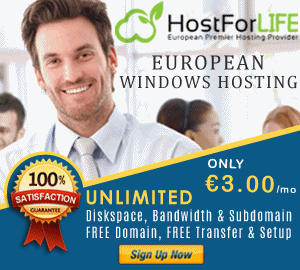
In Angular, @Input() and @Output() are decorators that allow a component to share data with its parent or child components, respectively. @ViewChild() is a decorator that allows a component to access a child component, directive, or DOM element.
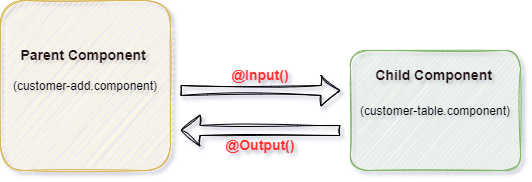
Understanding the use of @Input()
@Input() allows a component to receive data from its parent component. For example, a child component might use @Input() to receive data from its parent component like this:
Here, whenever I added a new entry as "Add Customer", the updated information is passed to the child component (e.g. Customer Table) and the child is acting as a term component. And the parent is acting like smart component.
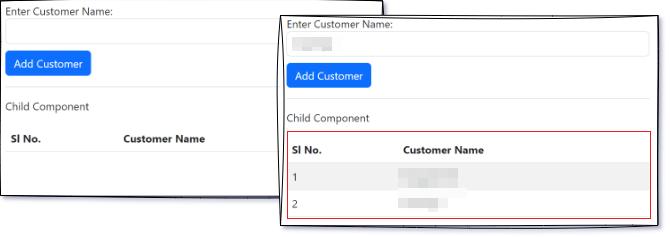
customer-add.component acts as a Parent Component
In this example, the parent component contains Add customer sections and a sub-component like a customer table. When we click on Add Customer that information being passed to the child component (customers table) and displayed on the table.
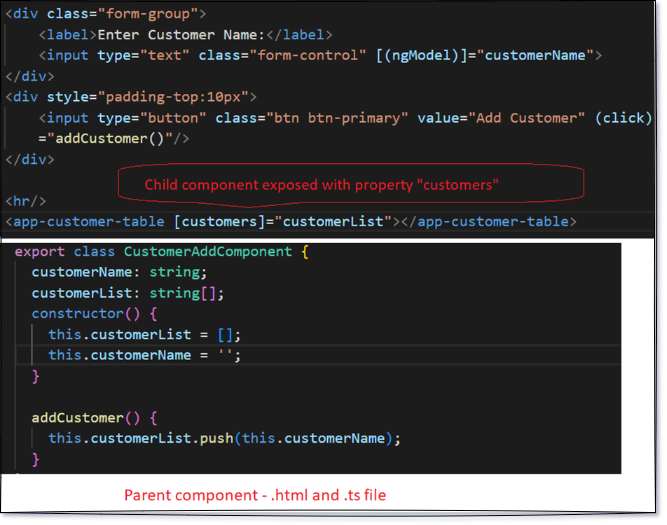
customer-table.component acts as a Child Component,
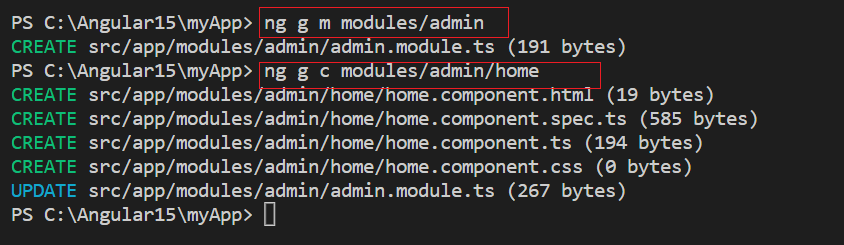
In the above example, the parent component uses the @Input() decorator to bind the "customers" property to a value passed from the parent component.
And the child component uses the @Input() decorator to receive the value passed from the parent component.
So, @Input() decorator is used to pass the data from the parent to the child component with help of property.
Understanding the use of @Output()
@Output() allows a component to emit events to its parent component. For example, a child component might use @Output() to emit an event when a button is clicked like this:
In the above example, whenever we select data from the customer table which acts as a child, that data should pass to the customer’s name textbox which acts as the parent. It’s basically sending data from child to parent. To do that, we need to expose an event with an output decorator.
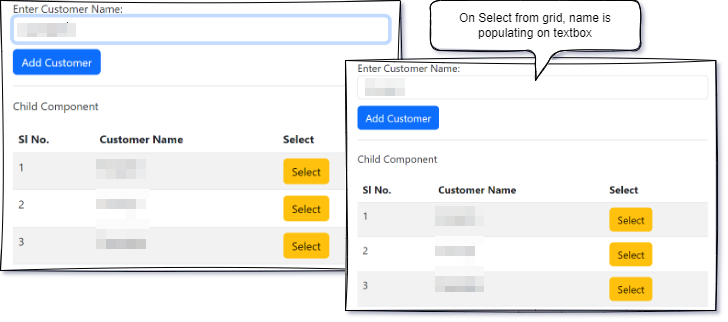
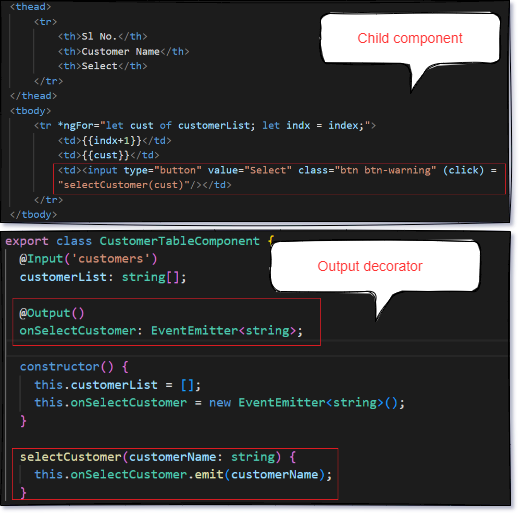
@Output() decorator to listen for an event emitted by the child component, which is then handled in the parent component.
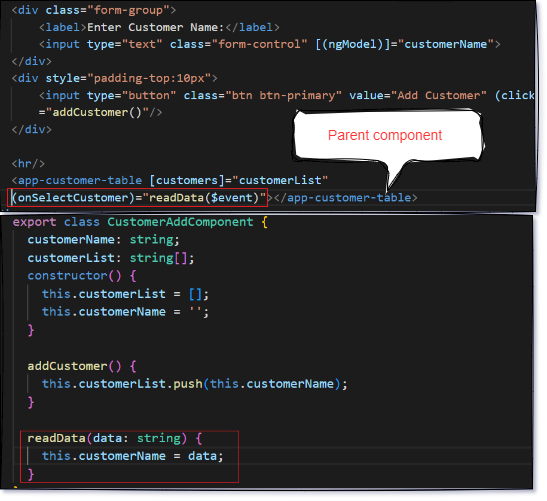
So, @Ouput() decorator is used to pass the data from child to parent with the help of the event.
Understanding the use of @ViewChild()
@ViewChild() allows a component to access a child component or DOM element. For example, a parent component might use @ViewChild() to access a child component like this:
In order to use the @ViewChild() decorator, we need to reference the child component in the parent component's class, and then we can use to query the child component's properties and methods or manipulate the DOM directly.
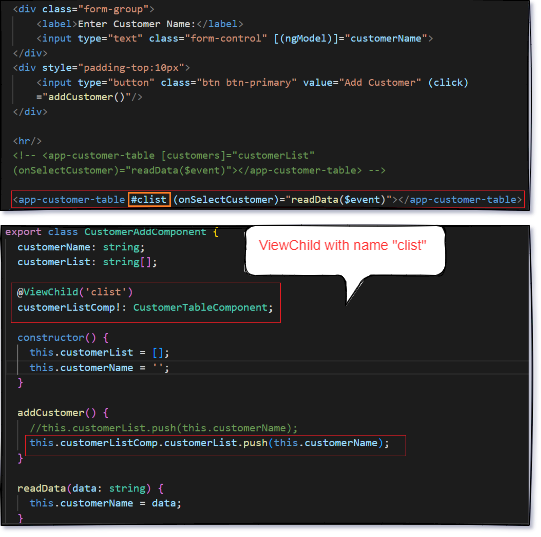
Another example where @ViewChild() can be used to access the DOM element as like below:
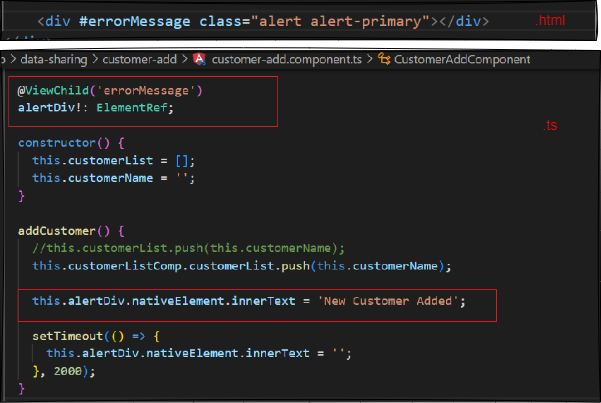
In summary, ViewChild is used to access child components or elements in the template, while @Input and @Output are used to pass data between components. If you need to access a specific child component or element in the template, ViewChild will be more useful, while if you need to pass data between components, @Input and @Output will be more useful.