
November 18, 2020 07:38 by
Peter
In this article we will observe uploading a file on a web server made use Node.js. Stream in Node.js makes this task super simple to upload files or so far as that is concerned working with any information exchange between a server and a client. To transfer a file we will work with two modules, HTTP and fs. So let us begin with stacking these two modules in an application:
var http = require('http');
var fs = require('fs')
When modules are loaded proceed to and make a web server as below:
http.createServer(function(request,response){
}).listen(8080);
So far we are great and now we wish to utilize the accompanying procedure:
Make a destination write stream. In this stream the substance of the uploaded file will be written. We need to compose once again to the client the rate of data being uploaded.
The first requirement could be possible utilizing a pipe. A pipe is an event of stream in Node.js. And the request is a readable stream. So we will use a pipe event to write a request to a readable stream.
var destinationFile = fs.createWriteStream("destination.md");
request.pipe(destinationFile);
The second necessity is to give back an of data uploaded. To do that first read the aggregate size of the file being uploaded. That could be possible by reading the content-length (line number 1 in the accompanying code snippet). At that point in the data occasion of request we will update uploadedBytes that starts at zero (line number 2). In the data event of the request we are calculating the percentage and writing it back in the response.
Now, It’s time to putting it all together your app should contain the following code to upload a file and return the percentage uploaded.
var http = require('http');
var fs = require('fs');
http.createServer(function(request,response){
response.writeHead(200);
var destinationFile = fs.createWriteStream("destination.md");
request.pipe(destinationFile);
var fileSize = request.headers['content-length'];
var uploadedBytes = 0 ;
request.on('data',function(d){
uploadedBytes += d.length;
var p = (uploadedBytes/fileSize) * 100;
response.write("Uploading " + parseInt(p)+ " %\n");
});
request.on('end',function(){
response.end("File Upload Complete");
});
}).listen(8080,function(){
console.log("server started");
});
On a command prompt start the server as in the picture below:
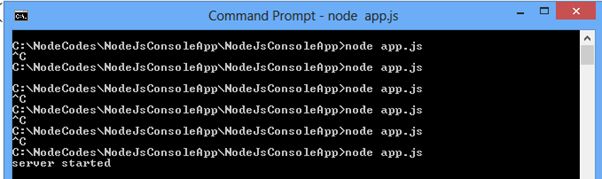
Presently let us utilize curl -upload-file to upload a file on the server.
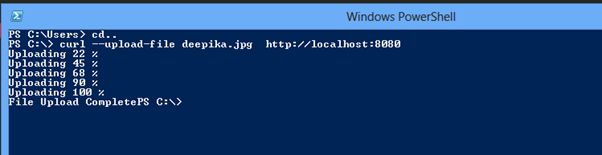
As you see, while the file is being uploaded the percentage of data uploaded is returned once again to the client. So thusly you can upload a file to the server made utilizing Node.js. Hope this tutorial works for you!