
March 18, 2024 07:59 by
Peter
AG-Grid is a popular module for building data grids and tables in Angular apps. To add controls or features to an AG-Grid in an Angular application, follow the steps below:
Install AG-Grid. If you haven't already, you should install AG-Grid and its Angular wrapper. You can accomplish this with npm:
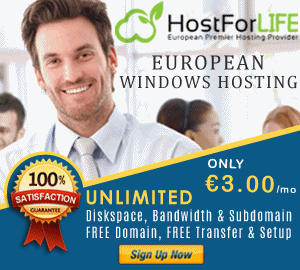
npm install --save ag-grid-angular ag-grid-community
Import AG-Grid Module
Import the AG-Grid module in your Angular application. In your Angular module (e.g., app.module.ts), add the following imports:
import { AgGridModule } from 'ag-grid-angular';
@NgModule({
imports: [
AgGridModule.withComponents([]), // You can specify custom components here if needed
// other modules
],
// ...
})
Create an AG-Grid Component
Create a component where you want to add your AG-Grid. You can use the Angular CLI to generate a component:
ng generate component grid
Set up the Grid in Your Component
In your newly created component (e.g., grid.component.ts), define your AG-Grid configuration. You can customize your grid by specifying column definitions, data, and other settings. Here's a basic example:
import { Component } from '@angular/core';
@Component({
selector: 'app-grid',
templateUrl: './grid.component.html',
styleUrls: ['./grid.component.css']
})
export class GridComponent {
gridOptions: any;
constructor() {
this.gridOptions = {
columnDefs: [
{ headerName: 'ID', field: 'id' },
{ headerName: 'Name', field: 'name' },
// Add more column definitions as needed
],
rowData: [
{ id: 1, name: 'Gajendra' },
{ id: 2, name: 'Sachin' },
// Add more data as needed
]
};
}
}
Render the Grid in Your Component's Template
In your component's HTML file (e.g., grid.component.html), add the AG-Grid component using the <ag-grid-angular> tag and bind it to your gridOptions:
<ag-grid-angular
style="width: 100%; height: 500px;"
class="ag-theme-alpine"
[gridOptions]="gridOptions"
></ag-grid-angular>
Add Controls and Features
To add controls or features, you can manipulate the gridOptions object in your component. For example, you can add custom buttons or other UI elements outside the grid to trigger actions or manipulate data within the grid.
Style Your Grid
You can style your grid by using CSS classes and themes. In the example above, the class "ag-theme-alpine" is applied to style the grid. You can use different themes or customize the styles as needed.
Build and Run
Finally, build and run your Angular application to see the AG-Grid with controls and features.
This is a basic example of how to set up AG-Grid in an Angular application. Depending on your requirements, you can further enhance the grid by adding more features, custom components, and event handling to make it more interactive and functional.