
September 22, 2023 09:05 by
Peter
What are Angular Signals?
Angular Signals is a new feature in Angular 16 that allows you to develop reactive code in a new way. Signals are a reactive primitive, which implies they can alert their dependents when their value changes. This makes them suitable for use in reactive applications, where it is critical to be able to automatically adjust the UI in reaction to data changes.
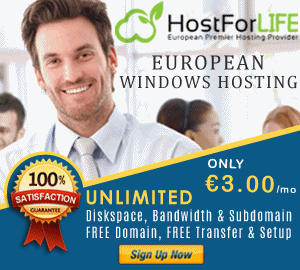
To begin with Angular Signals, import the Signals module from the @angular/core package. The signal() method is then used to create a signal. This function takes two arguments: the signal's initial value and an options object. The options object is used to determine whether the signal is written or read-only, as well as other parameters.
The subscribe() function
You can subscribe to a signal after you've produced it with the subscribe() method. As an argument, this method accepts a function that will be called whenever the signal changes. You can also use the unsubscribe() method to unsubscribe from a signal.
Signals can be utilized to construct a wide range of reactive patterns. You can, for example, use a signal to represent a component's state and then subscribe to the signal to update the UI whenever the state changes. Signals can also be used to provide two-way data coupling between components.
Here's a simple demonstration of how to use Angular Signals.
import { Signals } from '@angular/core';
export class MyComponent {
count = new Signals<number>(0);
increment() {
this.count.value++;
}
decrement() {
this.count.value--;
}
constructor() {
this.count.subscribe(value => {
// Update the UI here
});
}
}
In this case, we'll build a signal to indicate the current count. Then, we subscribe to the signal so that the UI may be updated anytime the count changes. Angular Signals have several advantages over alternative methods of developing reactive Angular programs. They are more granular than observables, for example, and can be used to track changes to individual values or states. They are also more synchronous, which makes them easier to debug and comprehend.
Overall, Angular Signals is a potent new tool for writing more reactive and efficient Angular programs.
Benefits of using Angular Signals
Here are some of the benefits of using Angular Signals.
- Granular updates: Angular Signals allow you to track changes to specific values or states rather than having to track changes to entire observables. This can lead to more efficient change detection and faster updates to the UI.
- A new reactive primitive: Angular Signals are a new reactive primitive, which means that they can be used to implement a variety of different reactive patterns in a simple and straightforward way.
- Simplified framework: Angular Signals simplify the Angular framework by providing a unified way to represent and track reactive values. This makes it easier to write and maintain reactive Angular code.
- No side effects: Angular Signals are designed to be pure, which means that they do not have any side effects. This makes them easier to reason about and debug.
- Automatic dependency tracking: Angular Signals automatically track their dependencies, so you don't have to worry about manually unsubscribing from them. This can help to prevent memory leaks and improve the overall performance of your application.
If you are writing reactive Angular code, I encourage you to try using Angular Signals. They are a powerful new tool that can make your code more efficient, simpler, and more reliable.