
May 2, 2024 08:45 by
Peter
This blog post will explain how to use the pyshorteners module to integrate Node.js and Python to build a basic URL shortening application. We can take advantage of Node.js's robust features for controlling the entire application and Python's URL-shortening functionality by leveraging the strengths of both languages.
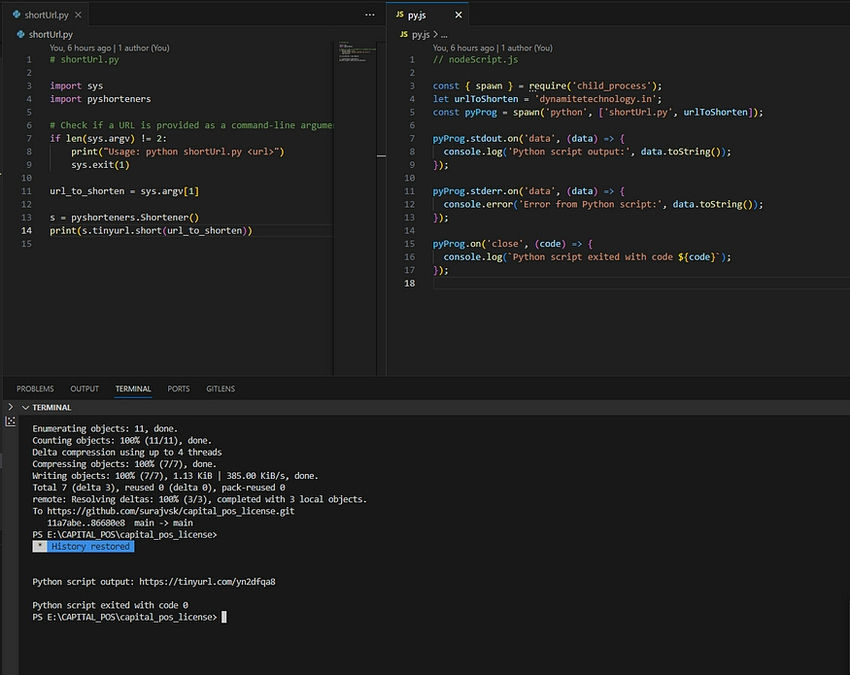
Node.js Script (nodeScript.js)
Let's begin by examining nodeScript.js, a Node.js script. This script is in charge of starting a Python process and sending a command-line argument with a URL to be abbreviated.
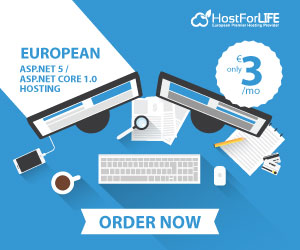
const { spawn } = require('child_process');
let urlToShorten = 'dynamitetechnology.in';
const pyProg = spawn('python', ['shortUrl.py', urlToShorten]);
pyProg.stdout.on('data', (data) => {
console.log('Python script output:', data.toString());
});
pyProg.stderr.on('data', (data) => {
console.error('Error from Python script:', data.toString());
});
pyProg.on('close', (code) => {
console.log(`Python script exited with code ${code}`);
});
Explanation
The spawn function from the child_process module is used to execute the Python script (shortUrl.py).
The URL to be shortened ('dynamitetechnology.in' in this case) is passed as a command-line argument when spawning the Python process.
Event listeners are set up to capture the standard output and standard error streams from the Python process.
Python Script (shortUrl.py)
Now, let’s take a look at the Python script, shortUrl.py. This script utilizes the pyshorteners library to perform URL shortening.
import sys
import pyshorteners
# Check if a URL is provided as a command-line argument
if len(sys.argv) != 2:
print("Usage: python shortUrl.py <url>")
sys.exit(1)
url_to_shorten = sys.argv[1]
s = pyshorteners.Shortener()
print(s.tinyurl.short(url_to_shorten))
Explanation
The Python script checks whether a URL is provided as a command-line argument. If not, it prints a usage message and exits.
The provided URL is then passed to the pyshorteners library, and the shortened URL is printed to the console using the TinyURL service.
Conclusion
Python and Node.js were used to develop a straightforward but powerful URL-shortening tool. Python performs the URL shortening functionality, and Node.js provides the orchestration and communication with the Python script. This integration demonstrates the adaptability and compatibility of many programming languages, enabling programmers to take advantage of each language's advantages for particular tasks inside a single application.