
May 26, 2023 07:41 by
Peter
Step 1. Define Model
Create your entity models as usual to begin. These models should represent your entities and contain all of the necessary properties. Suppose, for instance, we have a "Product" entity:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public bool IsDeleted {get; set; }
}
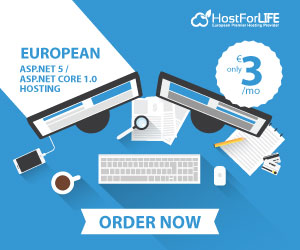
Configure the Global Query Filter
Implement an override for the OnModelCreating method in your DbContext class. Using the Entity and HasQueryFilter methods, you can configure global query filters within this method. Here's an example of excluding soft-deleted products using a global query filter:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>().HasQueryFilter(p => !p.IsDeleted);
}
3. Execute Filtered Queries
Once the global query filter is configured, it will be automatically applied to any query involving the Product entity. For instance, soft-deleted products will be excluded by default when retrieving products.
activeProducts is equal to dbContext.Products.ToList();
Advantages of Global Query Filters in C#
Data Integrity: Ensure that all queries involving the entity adhere to the desired filtering conditions, thereby fostering the consistency and integrity of the data.
By using global query filters, you can centralize the filtering logic, making your code simpler and easier to maintain.
Global query filters enable the exclusion of superfluous data at the database level, resulting in improved query performance and resource utilization.
Implementing global query filters in Entity Framework provides a potent tool for consistently applying filtering conditions to your entities. By configuring global query filters during the OnModelCreating method, you can ensure that the filters are applied automatically to all queries involving the specified entity, resulting in clearer code and enhanced data integrity. Test it out!