
June 29, 2022 08:01 by
Peter
Pagination is a very basic function that we need in every application. So let's try to understand how we can apply pagination in our angular app. To implement pagination we are using PrimeNG DataTable Pagination
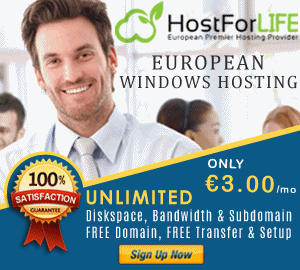
What is PAGINATION?
Pagination is a component that displays page informations like page-number | total-records. To implement pagination we need to manipulate our data and split it into page collection.
Getting Started
First Install the required libraries
npm install bootstrap
npm install primeng
npm install primeicons
npm install @angular/cdk
Second add the reference in styles
"styles": [
"node_modules/bootstrap/dist/css/bootstrap.min.css",
"node_modules/primeng/resources/themes/saga-blue/theme.css",
"node_modules/primeng/resources/primeng.min.css",
"node_modules/primeicons/primeicons.css",
"src/styles.scss"
],
Third register the module in app.module.ts file
import { TableModule } from 'primeng/table';
imports: [
// PrimeNG Modules
TableModule
]
Fourth step is to define the datatable. Now open the component.html file and add the ptable
<p-table></p-table>
Rows and TotalRecords
Rows, Rows Per Page, Current Page Report Template and other datatable options we need to define how many pages the paginator should display. Paginator below will have 10 rows per page.
<p-table [value]="userList" [paginator]="true" [rows]="10" [showCurrentPageReport]="true" responsiveLayout="scroll" currentPageReportTemplate="Showing {first} to {last} of {totalRecords} entries" [rowsPerPageOptions]="[10,25,50]">
</p-table>
Defining the table header
<ng-template pTemplate="header">
<tr>
<th>Name</th>
<th>Email</th>
<th>Mobile</th>
<th>DOB</th>
<th>isActive</th>
<th>Action</th>
</tr>
</ng-template>
Defining the table body
<ng-template pTemplate="body" let-user>
<tr [ngClass]="{'inActive': user.isActive == false}">
<td>{{user.name}}</td>
<td>{{user.email}}</td>
<td>{{user.mobile}}</td>
<td>{{user.dob | date:'d/M/yyyy'}}</td>
<td>{{user.isActive}}</td>
<td>
<a pButton pRipple type="button" [routerLink]="['/update-user',user.id]" icon="pi pi-user-edit"
class="p-button-rounded"></a>
<a pButton pRipple type="button" (click)="remove(user.id)" style="margin-left: 5px;"
icon="pi pi-trash" class="p-button-rounded"></a>
</td>
</tr>
</ng-template>
Defining table footer where we add various options like add new user
<ng-template pTemplate="paginatorleft">
<a [routerLink]="['/add-user']" class="btn btn-primary">Add New</a>
</ng-template>
Complete Code
<div class="container" style="margin-top: 20px;">
<p-table [value]="userList" [paginator]="true" [rows]="10" [showCurrentPageReport]="true" responsiveLayout="scroll"
currentPageReportTemplate="Showing {first} to {last} of {totalRecords} entries"
[rowsPerPageOptions]="[10,25,50]">
<ng-template pTemplate="header">
<tr>
<th>Name</th>
<th>Email</th>
<th>Mobile</th>
<th>DOB</th>
<th>isActive</th>
<th>Action</th>
</tr>
</ng-template>
<ng-template pTemplate="body" let-user>
<tr [ngClass]="{'inActive': user.isActive == false}">
<td>{{user.name}}</td>
<td>{{user.email}}</td>
<td>{{user.mobile}}</td>
<td>{{user.dob | date:'d/M/yyyy'}}</td>
<td>{{user.isActive}}</td>
<td>
<a pButton pRipple type="button" [routerLink]="['/update-user',user.id]" icon="pi pi-user-edit"
class="p-button-rounded"></a>
<a pButton pRipple type="button" (click)="remove(user.id)" style="margin-left: 5px;"
icon="pi pi-trash" class="p-button-rounded"></a>
</td>
</tr>
</ng-template>
<ng-template pTemplate="paginatorleft">
<a [routerLink]="['/add-user']" class="btn btn-primary">Add New</a>
</ng-template>
</p-table>
</div>
Open the component file and add the below mentioned methods for pagination
import { Component, OnInit } from '@angular/core';
import { UserService } from '../service/user.service';
import { User } from '../User';
@Component({
selector: 'app-user-list',
templateUrl: './user-list.component.html',
styleUrls: ['./user-list.component.css']
})
export class UserListComponent implements OnInit {
userList: User[] = [];
first = 0;
rows = 10;
constructor(private userService: UserService) {}
ngOnInit(): void {
// Get Users from UserService
this.userList = this.userService.getUsers();
}
//****************PrimeNG DataTable Pagination method Start*********************** */
//***************Reference: https://primefaces.org/primeng/showcase/#/table/page********** */
next() {
this.first = this.first + this.rows;
}
prev() {
this.first = this.first - this.rows;
}
reset() {
this.first = 0;
}
isLastPage(): boolean {
return this.userList ? this.first === (this.userList.length - this.rows) : true;
}
isFirstPage(): boolean {
return this.userList ? this.first === 0 : true;
}
//****************PrimeNG DataTable Pagination Method End*********************** */
// ********************User To Remove User from User List*************************/
remove(id: number) {
this.userService.removeUser(id);
this.userList = this.userService.getUsers();
}
}
Run the application using npm start.