Routing in Angular enables you to move between several parts (or views) in a single-page application (SPA). To do this, routes that associate URL pathways with particular components are built up. The relevant component loads and displays in a preset area of your application (typically designated by a <router-outlet>) when a user navigates to a URL.
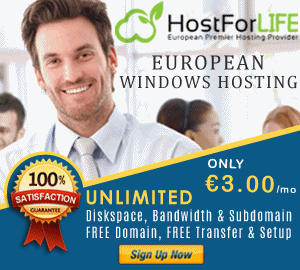
to use the robust routing system of Angular to move between components in an efficient manner. This article covers everything from configuring routes to using Angular's Router service programmatically and utilizing RouterLink for template-based navigation. You will learn how to map URLs to components and render them dynamically using <router-outlet>, providing smooth navigation inside your Angular applications, with concise explanations and functional code examples.
Key Concepts
- Router Module: This is Angular's mechanism for handling routing. You define the routes in a routing module (often AppRoutingModule), where each route maps a URL path to a component.
- RouterLink Directive: This directive is used in HTML templates to bind a URL to an anchor tag or button. When clicked, Angular will navigate to the route specified by the RouterLink.
- Router Service: This service provides methods to navigate to a route programmatically from within a component’s TypeScript file.
- Router Outlet: This is a directive that acts as a placeholder where the routed component will be rendered.
Step-by-Step Implementation with Code Example
1. Setting Up Routes
First, create your components if you haven't already.
ng generate component component-a
ng generate component component-b
Then, define the routes in the AppRoutingModule.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { ComponentA } from './component-a/component-a.component';
import { ComponentB } from './component-b/component-b.component';
const routes: Routes = [
{ path: 'component-a', component: ComponentA },
{ path: 'component-b', component: ComponentB },
{ path: '', redirectTo: '/component-a', pathMatch: 'full' } // Default route
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
2. Navigating Using RouterLink
In ComponentA, you can create a link that navigates to ComponentB.
<!-- component-a.component.html -->
<h2>This is Component A</h2>
<a [routerLink]="['/component-b']">Go to Component B</a>
When the user clicks on this link, Angular will navigate to /component-b and load ComponentB into the router outlet.
3. Navigating Programmatically Using the Router Service
You can also navigate programmatically from within a component’s TypeScript file.
// component-a.component.ts
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-component-a',
templateUrl: './component-a.component.html',
styleUrls: ['./component-a.component.css']
})
export class ComponentA {
constructor(private router: Router) { }
goToComponentB() {
this.router.navigate(['/component-b']);
}
}
In the corresponding HTML.
<!-- component-a.component.html -->
<h2>This is Component A</h2>
<button (click)="goToComponentB()">Go to Component B</button>
4. Using Router Outlet
Ensure you have a <router-outlet> in your main HTML template (usually app.component.html).
<!-- app.component.html -->
<router-outlet></router-outlet>
This directive is where the routed components will be displayed based on the URL.
5. Putting It All Together
Here’s how everything ties together.
- AppRoutingModule: Defines the routes.
- ComponentA: Has a link and a button to navigate to ComponentB.
- ComponentB: Displays content when navigated to.
Example Code Structure// app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { ComponentA } from './component-a/component-a.component';
import { ComponentB } from './component-b/component-b.component';
const routes: Routes = [
{ path: 'component-a', component: ComponentA },
{ path: 'component-b', component: ComponentB },
{ path: '', redirectTo: '/component-a', pathMatch: 'full' } // Default route
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
// component-a.component.ts
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'app-component-a',
templateUrl: './component-a.component.html',
styleUrls: ['./component-a.component.css']
})
export class ComponentA {
constructor(private router: Router) { }
goToComponentB() {
this.router.navigate(['/component-b']);
}
}
// component-a.component.html
<h2>This is Component A</h2>
<a [routerLink]="['/component-b']">Go to Component B</a>
<button (click)="goToComponentB()">Go to Component B</button>
// component-b.component.html
<h2>This is Component B</h2>
// app.component.html
<router-outlet></router-outlet>
Summary
- Routes: Define in AppRoutingModule to map URLs to components.
- RouterLink: Use templates for simple navigation.
- Router Service: Use for programmatic navigation in TypeScript.
- Router Outlet: The placeholder for rendering routed components.
This setup allows you to navigate between components effectively, using both template-based and programmatic methods in Angular.