
June 26, 2024 07:59 by
Peter
You may use the built-in features of the Kendo Grid in conjunction with Angular's component lifecycle hooks to apply dynamic filters in a Kendo Grid based on the data type of each column. Here's how you can make this happen.
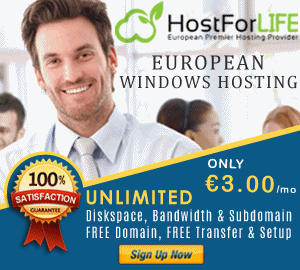
Install the required packages
Make sure the Angular Grid package's Kendo UI is installed.
npm install @progress/kendo-angular-grid \
@progress/kendo-angular-inputs \
@progress/kendo-angular-l10n \
@progress/kendo-angular-dateinputs \
@progress/kendo-angular-dropdowns --save
Import Kendo Modules
Import the necessary Kendo UI modules into your Angular module.
import { GridModule } from '@progress/kendo-angular-grid';
import { DateInputsModule } from '@progress/kendo-angular-dateinputs';
import { InputsModule } from '@progress/kendo-angular-inputs';
import { DropDownsModule } from '@progress/kendo-angular-dropdowns';
@NgModule({
imports: [
GridModule,
DateInputsModule,
InputsModule,
DropDownsModule
// other imports
],
// other configurations
})
export class AppModule {}
Define the grid in your component template
Create the Kendo Grid in your component template and bind it to a data source.
<kendo-grid [data]="gridData" [filterable]="true">
<kendo-grid-column *ngFor="let column of columns"
[field]="column.field"
[title]="column.title"
[filter]="getFilterType(column)">
</kendo-grid-column>
</kendo-grid>
Determine the filter type dynamically
In your component, write a method to return the filter type based on the column's data type.
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-dynamic-filter-grid',
templateUrl: './dynamic-filter-grid.component.html',
styleUrls: ['./dynamic-filter-grid.component.css']
})
export class DynamicFilterGridComponent implements OnInit {
public gridData: any[] = [
{ name: 'John', age: 30, birthday: new Date(1990, 1, 1) },
{ name: 'Jane', age: 25, birthday: new Date(1995, 2, 1) },
// more data
];
public columns: any[] = [
{ field: 'name', title: 'Name', type: 'string' },
{ field: 'age', title: 'Age', type: 'number' },
{ field: 'birthday', title: 'Birthday', type: 'date' }
];
constructor() { }
ngOnInit(): void { }
getFilterType(column: any): string {
switch (column.type) {
case 'date':
return 'date';
case 'number':
return 'numeric';
default:
return 'text';
}
}
}
Add the component template
In your component HTML file, use the Kendo Grid and call the dynamic filter method.
<kendo-grid [data]="gridData" [filterable]="true">
<kendo-grid-column *ngFor="let column of columns"
[field]="column.field"
[title]="column.title"
[filter]="getFilterType(column)">
</kendo-grid-column>
</kendo-grid>
Conclusion
With these steps, your Kendo Grid will apply filters dynamically based on the data type of each column.