In this article, we are going to discuss the most important feature in the Angular Framework called “Featured Modules”. This article can be accessed by beginners, intermediates, and professionals.
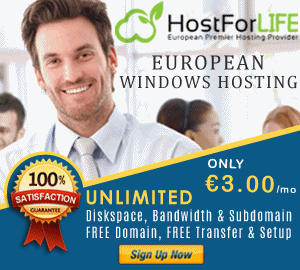
Featured Module
Modules are the most important part of the Angular Application.
Modules are the place where we are grouping components, directives, services, and pipes.
app.module.ts module is a root module of the Angular Application.
Whenever we create a new application, the root module will be added by default.
Let's see the below image to understand the problem first,
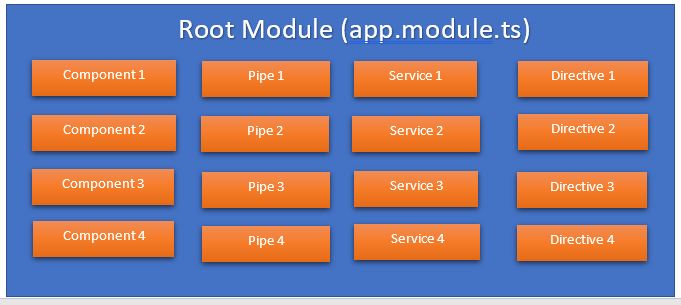
Suppose we have a large angular application with thousands of components, directives, services, pipes, etc., and all are maintained in the Root module (“app.module.ts”). In such a scenario, the Root module becomes very mashie and non-maintainable.
What will you do to solve this problem? You will break the root module into small modules and separate it right? So that separated small module is called a “Featured module” in Angular.
Let’s see the below image for more understanding,
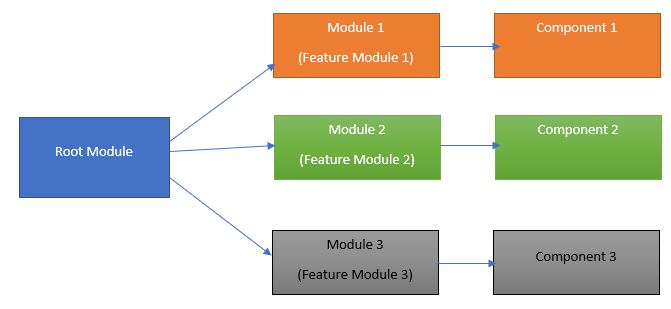
In the above image, we have separated “module1”,” module2” and “module3” from the Root module. Each module content component of that module. Eg. Module1 has Component1
Now we have a basic idea of the Featured module, now we will see how many types of featured modules are available in the angular application.
Types of Featured Modules
Domain - It is based on the application navigation eg. Emp or customer features modules etc
Routed - All Lazy loaded modules.
Routing - Routing and its related functionality like Route guard.
Service - This is related to API request – Response.
Widget - Widget-related modules which may contain utility, pipes, directives, etc.
Let’s discuss a few important advantages before we will discuss an example of a featured module.
Advantages of the Featured Module
Code Maintainability
Scalability
Lazy Loading
Abstraction
Code separation
Now we will create a new angular application and learn feature modules,
Step 1
Create a new Angular Application “ProductDemo”.
ng new "FeaturedDemo"
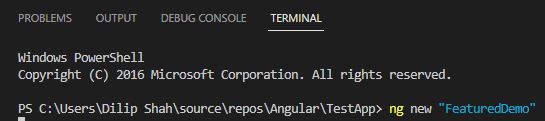
Step 2
Add two modules “ProductModule” and “PriceModule” components.
ng g m "Product\Product.module"
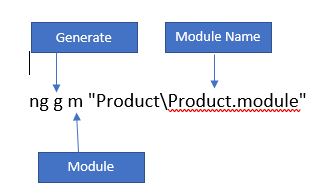
Above command will create product folder and product module.
Now we will create new module for price. We will use the below command for the same.
ng g m price\price
Price folder and price module will be created.
Step 3 - Create two components for “Product” and “price”.
Now we will add two components for product and price.
ng g c product\product
Product components will be created. Now we will create price component.
ng g c price\price
Price component will be created and added in the price folder.
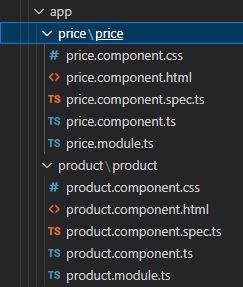
Step 4 - Configure “ProductModule” and “PriceModule” in the app.module.ts file.
Now we will configure newly added modules in the app.module.ts file.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { PriceModule } from './price/price/price.module';
import { ProductModule } from './product/product/product.module';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
ProductModule,
PriceModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
We have added “ProductModule” and “PriceModule” in the imports array and imported references in the app.module.ts file.
app.module.ts file has “BrowserModule” but other modules have “CommonModule” for common functionalities.
Step 5
Let's see “ProductModule” and “PriceModule”.
Product Module
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { ProductComponent } from './product.component';
@NgModule({
declarations: [
ProductComponent
],
imports: [
CommonModule
],
exports:[ProductComponent]
})
export class ProductModule { }
We need to export ProductComponent to export the component. Need to add components to the exports array.
Price Module
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { PriceComponent } from './price.component';
@NgModule({
declarations: [
PriceComponent
],
imports: [
CommonModule
],
exports:[PriceComponent]
})
export class PriceModule { }
Step 6
Add the below code in the “app.component.html” file.
<router-outlet></router-outlet>
<app-price></app-price>
<app-product></app-product>
Now we will execute the angular application and see the results.
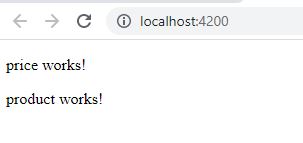
That's all for this article. Hope you enjoyed it.