Angular Interceptor helps us modify the HTTP request by intercepting it before the request is sent to the backend. You can also change the incoming response from the backend. The Interceptor globally captures all incoming and outgoing requests in one place. We can use it to add custom headers to the outgoing request, log the incoming response, etc.
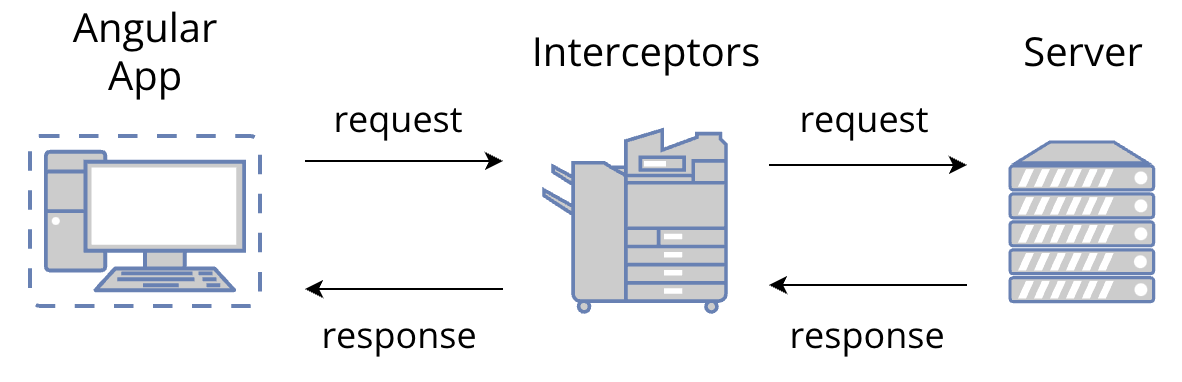
What is HTTP Interceptor?
An Angular HTTP interceptor sits between your application and the backend. When the application makes a request, the interceptor sees the request (HttpRequest) before sending it to the backend. You can access the request headers and body by intercepting the request. This allows us to transform the request before sending it to the server.
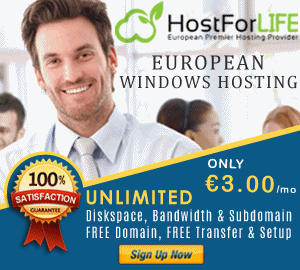
When the response (HttpResponse) arrives from the backend, interceptors can transform it before forwarding it to the application. One of the main advantages of HTTP interceptors is the ability to add an Authorization header to every request. You can do this manually, but it's tedious and error-prone. Another benefit is the ability to catch and log errors generated by requests.
How to Create Interceptor?
To implement an interceptor, we need to create an injectable service that implements the HttpInterceptor interface.
@Injectable() export class MyHttpInterceptor implements HttpInterceptor {
This class should implement the Intercept method
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
//Do the code whatever you want with the HTTP request
return next.handle(req);
}
This class is exposed in the root module using the HTTP_INTERCEPTORS injection token.
providers: [
{
provide: HTTP_INTERCEPTORS,
useClass: MyHttpInterceptor,
multi: true
}
],
Interface: HTTP Interceptor
At the guts of the Interceptor, logic is the HttpInterceptor Interface. we have a tendency to should Implement it in our Interceptor Service.
The interface contains one method Intercept with the subsequent signature
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>>
HTTP Request
The first argument is HttpRequest.
HttpRequest is an intercepted outgoing HTTP request. It integrates URLs, methods, headers, body, and various request configurations.
HttpRequest is an immutable class. In any way, we cannot accommodate genuine claims. To make adjustments, we want to duplicate the original request using the HttpRequest.clone
HTTP Handler
The second argument is httpHandler
HttpHandler sends the HttpRequest to the next Controller using the HttpHandler.handleMethod. The next handler can be another interceptor in on Thread or the Http Backend.
Let's see the example
Create MyHttpInterceptor.ts under /app folder and paste the below code
import {Injectable} from "@angular/core";
import {HttpEvent, HttpHandler, HttpInterceptor,HttpRequest} from "@angular/common/http";
import {Observable} from "rxjs/Observable";
@Injectable()
export class MyHttpInterceptor implements HttpInterceptor {
constructor() {
}
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
return next.handle(req);
}
}
In the body of the method, you should be able to modify the HttpRequest object. Once done, you can select the HttpHandler.handle method from the HttpHandler with the HttpRequest object. The HttpHandler.handle method calls the fighter thus or sends a request to the main server.
App.Module
Whole code from the app module
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule,HTTP_INTERCEPTORS} from '@angular/common/http';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { GitHubService } from './github.service';
import {MyHttpInterceptor} from './MyHttpInterceptor';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [GitHubService,
{
provide: HTTP_INTERCEPTORS,
useClass: MyHttpInterceptor,
multi: true
}
],
bootstrap: [AppComponent]
})
export class AppModule { }
If you have faced any error regarding the import module please check your package.json file and install the required package if not installed.
So, here interceptor-adding process is done let's run the app and you can see every HTTP calls interceptor, You can put the debugger in the interceptor and see.
Let's add content type using interceptor
Content Type Adding
To modify the request we want to clone it using HttpRequest.clone
req = req.clone({ headers: req.headers.set('Content-Type', 'application/json') });
The header object is also unchanged. Therefore, we need to duplicate it using the headers.set method. The header.set method copies the current header and adds/modifies the new header value and returns the copied header.
We can also use the header.append. The append technique continually appends the header albeit the worth is already present.
And we can also remove the header like the one below shown
req = req.clone({ headers: req.headers.delete('Content-Type','application/json') });
If you want to add authorization token then we can add a token as shown below
const bearerToken: string =authService.Token; // here you can use your service method where get token
if (token) {
req = req.clone({ headers: req.headers.set('Authorization', 'Bearer ' + bearerToken) });
}
So we can change or add code as per our requirement like adding logging, modifying response, catching the errors, etc...