
March 20, 2023 09:36 by
Peter
RxJS has two main approaches to handling asynchronous data streams: procedural and declarative.
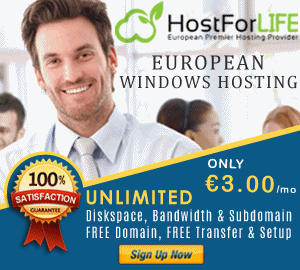
I have used the below tools to prepare this tutorial.
Angular CLI - 15.1.6
Node: 18.14.0
NPM – 9.3.1
Visual Studio Code
Procedural programming involves using imperative code to handle asynchronous operations, such as making HTTP requests and updating the UI. Procedural code typically involves chaining together methods and callbacks to create a sequence of steps that must be executed in a specific order. Procedural code can be challenging to read, understand, and maintain, especially as the codebase grows.
Sample code snippet for Procedural Approach,
// All users
getUsers(): Observable<User[]> {
return this.http.get<User[]>(this.userUrl).pipe(
catchError(this.handleError)
);
}
The code defines a function called getUsers() that returns an Observable of an array of User objects. Overall, this code defines a procedure in Angular to retrieve a list of users from a specific URL using the HttpClient and handle any errors that may occur during the HTTP request.
On the other hand, declarative programming involves using a functional approach to handle asynchronous data streams. With declarative programming, you create Observables that emit data and use operators to transform, filter, and combine these data streams. Declarative code is typically easier to read and understand and can be more modular and reusable.
Code snippet for Declarative Approach,
/All Users
users$ =this.http.get<User[]>(this.userUrl).pipe(
catchError(this.handleError)
)
The code defines a property called users$ using the declarative approach in Angular.
The $ symbol at the end of the property name is a convention used in Angular to indicate that the property is Observable. The property is being initialized with the result of an HTTP GET request made using the HttpClient. The request is made to this.userUrl URL and expects to receive an array of User objects in the response. Overall, this code uses the declarative approach in Angular to define an Observable property that retrieves a list of users from a specific URL using the HttpClient.
In Angular, the declarative approach to RxJS is generally preferred over the procedural approach. Angular provides many built-in directives and pipes that allow you to use declarative code to handle common use cases, such as displaying data from an HTTP request or handling user input.