
January 22, 2024 07:12 by
Peter
In Angular, key modifiers are special keys that you can use in combination with other keys to capture specific keyboard events. These modifiers help you distinguish between different key combinations and provide more flexibility in handling user input.
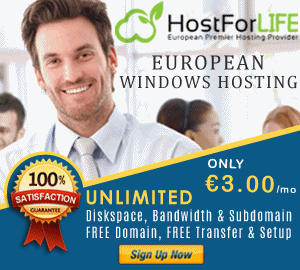
Key modifiers in Angular
Here are some key modifiers commonly used in Angular.
$event: This is a special variable that you can use to access the native event object in your event handler. For example.
<input (keyup)="onKeyUp($event)">
In the component:
onKeyUp(event: KeyboardEvent): void {
// Access event properties, such as event.keyCode, event.key, etc.
}
(keyup.enter) and (keyup.esc): These are examples of key modifiers for specific keys. They allow you to handle events triggered by the Enter key or the Escape key.
<input (keyup.enter)="onEnterKey()" (keyup.esc)="onEscapeKey()">
In the component
onEnterKey(): void {
// Handle Enter key press
}
onEscapeKey(): void {
// Handle Escape key press
}
(keydown.space): Similar to (keyup.enter), this allows you to handle events triggered by the Space key.
<button (keydown.space)="onSpaceKey()">Click me with Space</button>
In the component
onSpaceKey(): void {
// Handle Space key press
}
(keyup.shift): You can use this to handle events triggered when the Shift key is released.
<input (keyup.shift)="onShiftKeyUp()">
In the component
onShiftKeyUp(): void {
// Handle Shift key release
}
(keydown.ctrl) and (keydown.meta): These modifiers allow you to handle events when the Ctrl (Control) key or the Meta key (Command key on Mac) is pressed.
<input (keydown.ctrl)="onCtrlKeyDown()" (keydown.meta)="onMetaKeyDown()">
In the component:
onCtrlKeyDown(): void {
// Handle Ctrl key press
}
onMetaKeyDown(): void {
// Handle Meta key press
}
With the help of these key modifiers, it is possible to record particular key-related events and carry out actions in response to user input. They make your Angular applications more interactive by enabling you to react to keyboard inputs in a more sophisticated