
February 28, 2020 11:08 by
Peter
Eager loading is the process whereby a query for one type of entity also loads related entities as part of the query, so that we don't need to execute a separate query for related entities.
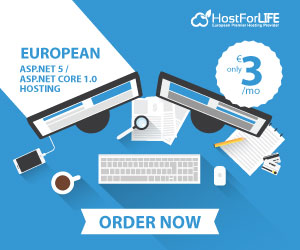
In simple language, Eager loading joins the entities which have foreign relation and returns the data in a single query.
Now, the question is how to properly handle the eager-loading problem for complex object graphs within the Repository pattern.
Let's get started.
Step 1: Add a method into your Interface which eager loads the entities which we specify:
public interface IProjectInterface<T> where T : class
{
Task<IEnumerable<T>> EntityWithEagerLoad(Expression<Func<T, bool>> filter, string[] children);
Task<List<T>> GetModel();
T GetModelById(int modelId);
Task<bool> InsertModel(T model);
Task<bool> UpdateModel(T model);
Task<bool> DeleteModel(int modelId);
void Save();
}
The method EntityWithEagerLoad() takes 2 arguments, one is filter criteria and another is an array of entities which we want to eager load.
Step 2: Define the method EntityWithEagerLoad in your base repository:
public async Task<IEnumerable<T>> EntityWithEagerLoad(Expression<Func<T, bool>> filter, string[] children)
{
try
{
IQueryable<T> query = dbEntity;
foreach (string entity in children)
{
query = query.Include(entity);
}
return await query.Where(filter).ToListAsync();
}
catch(Exception e)
{
throw e;
}
Step 3 : For using this method , call this function from your controller as shown below:
[HttpGet]
[Route("api/Employee/getDetails/{id}")]
public async Task<IEnumerable<string>> GetDetails([FromRoute]int id)
{
try
{
var children = new string[] { "Address", “Education” };
var employeeDetails=await _repository.EntityWithEagerLoad (d => d.employeeId==id,
children);
}
catch(Exception e)
{
Throw e;
}
The variable employeeDetails contains employee details with their Address and education details.