The JSON Web Token (JWT) authentication method is a popular security measure for Node.js applications. A token is created upon login and is used for subsequent request authentication. This tutorial shows you how to implement JWT authentication in a Node.js application using MySQL and Express. You'll learn how to set up the database, create authentication routes, and oversee token generation and validation. By securing your API endpoints and restricting access to protected resources to only authorized users, you may increase the security and scalability of your service.
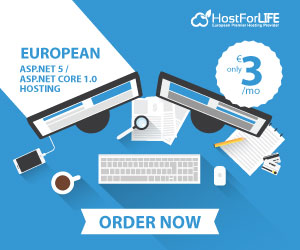
Steps for Creating a project
Step 1. Initialize a new Node.js project ;(CMD Command)
mkdir Node_JWT_Project
cd Node_JWT_Project
npm init -y
Step 2. Install the required packages ;(CMD Command)
npm install express mysql2 jsonwebtoken bcryptjs body-parser
Step 3. Create the project structure
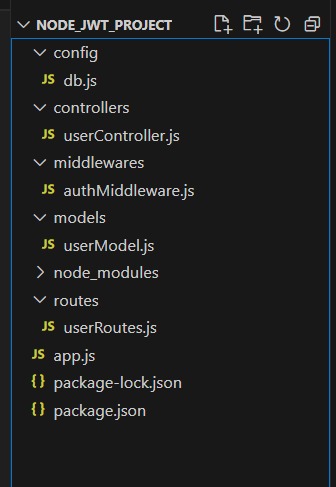
Step 4. Database Configuration (config/db.js)
const mysql = require('mysql2');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'my_database'
});
connection.connect((err) => {
if (err) {
console.error('Error connecting to the database:', err.stack);
return;
}
console.log('Connected to the database.');
});
module.exports = connection;
Step 5. Create a Model folder (models/userModel.js)
const db = require('../config/db');
const User = {};
User.create = (user, callback) => {
const query = 'INSERT INTO users (username, email, password) VALUES (?, ?, ?)';
db.query(query, [user.username, user.email, user.password], callback);
};
User.findById = (id, callback) => {
const query = 'SELECT * FROM users WHERE id = ?';
db.query(query, [id], callback);
};
User.findByEmail = (email, callback) => {
const query = 'SELECT * FROM users WHERE email = ?';
db.query(query, [email], callback);
};
User.update = (id, user, callback) => {
const query = 'UPDATE users SET username = ?, email = ? WHERE id = ?';
db.query(query, [user.username, user.email, id], callback);
};
User.delete = (id, callback) => {
const query = 'DELETE FROM users WHERE id = ?';
db.query(query, [id], callback);
};
module.exports = User;
Step 6. Controller (controllers/userController.js)
const User = require('../models/userModel');
const bcrypt = require('bcryptjs');
const jwt = require('jsonwebtoken');
const userController = {};
userController.register = async (req, res) => {
const { username, email, password } = req.body;
const hashedPassword = await bcrypt.hash(password, 10);
User.create({ username, email, password: hashedPassword }, (err, result) => {
if (err) {
return res.status(500).json({ error: err.message });
}
res.status(201).json({ message: 'User registered successfully!' });
});
};
userController.login = (req, res) => {
const { email, password } = req.body;
User.findByEmail(email, async (err, results) => {
if (err || results.length === 0) {
return res.status(400).json({ error: 'Invalid email or password' });
}
const user = results[0];
const isMatch = await bcrypt.compare(password, user.password);
if (!isMatch) {
return res.status(400).json({ error: 'Invalid email or password' });
}
const token = jwt.sign({ id: user.id }, 'your_jwt_secret', { expiresIn: '1h' });
res.json({ token });
});
};
userController.getUser = (req, res) => {
const userId = req.user.id;
User.findById(userId, (err, results) => {
if (err) {
return res.status(500).json({ error: err.message });
}
if (results.length === 0) {
return res.status(404).json({ message: 'User not found' });
}
res.json(results[0]);
});
};
userController.updateUser = (req, res) => {
const userId = req.user.id;
const { username, email } = req.body;
User.update(userId, { username, email }, (err, results) => {
if (err) {
return res.status(500).json({ error: err.message });
}
res.json({ message: 'User updated successfully!' });
});
};
userController.deleteUser = (req, res) => {
const userId = req.user.id;
User.delete(userId, (err, results) => {
if (err) {
return res.status(500).json({ error: err.message });
}
res.json({ message: 'User deleted successfully!' });
});
};
module.exports = userController;
Step 7. Routes (routes/userRoutes.js)
const express = require('express');
const router = express.Router();
const userController = require('../controllers/userController');
const authMiddleware = require('../middlewares/authMiddleware');
router.post('/register', userController.register);
router.post('/login', userController.login);
router.get('/profile', authMiddleware, userController.getUser);
router.put('/profile', authMiddleware, userController.updateUser);
router.delete('/profile', authMiddleware, userController.deleteUser);
module.exports = router;
Step 8. Middleware (middlewares/authMiddleware.js)
const jwt = require('jsonwebtoken');
const authMiddleware = (req, res, next) => {
const token = req.header('Authorization').replace('Bearer ', '');
if (!token) {
return res.status(401).json({ message: 'Access denied. No token provided.' });
}
try {
const decoded = jwt.verify(token, 'your_jwt_secret');
req.user = decoded;
next();
} catch (error) {
res.status(400).json({ message: 'Invalid token.' });
}
};
module.exports = authMiddleware;
Step 9. Main Application (app.js)
const express = require('express');
const bodyParser = require('body-parser');
const userRoutes = require('./routes/userRoutes');
const app = express();
app.use(bodyParser.json());
app.use('/api/users', userRoutes);
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Step 10. Create a Database and Table
CREATE DATABASE my_database;
USE my_database;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL,
password VARCHAR(255) NOT NULL
);
Step 11. Running the project (CMD Command)
node app.js
Step 12. Check-in Postman
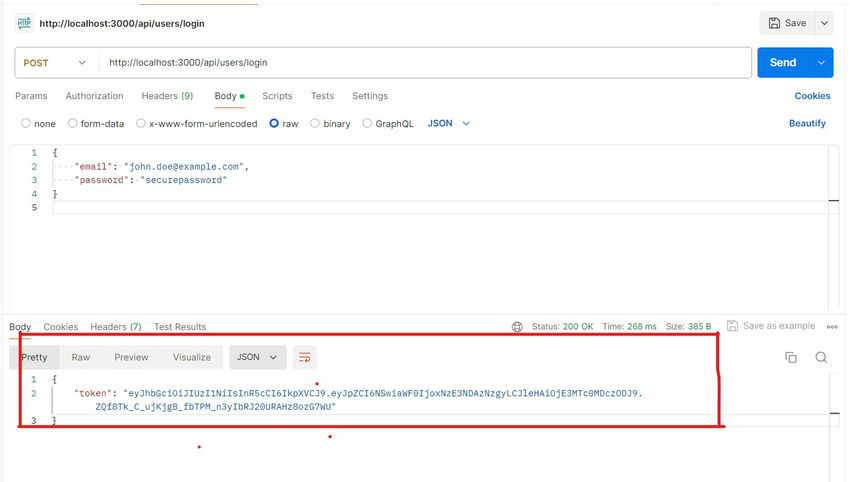
Similarly, we can use Endpoint Update, Insert, and Delete.
Conclusion
Only authenticated users can access protected resources, which improves security and scalability when JWT authentication is implemented in a Node.js application using MySQL. You may create a strong authentication system by following the procedures listed, which include setting up the database, making authentication routes, and creating and validating tokens. This method offers a scalable solution for user management in your application in addition to securing your API endpoints. You can make your application more dependable and user-friendly by maintaining a seamless and secure user experience with JWT authentication.
HostForLIFE.eu Node.js Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
