Today, I will show you how to upload a picture using AngularJS with ASP.NET 5. First, you must create a new project and then write the following code:
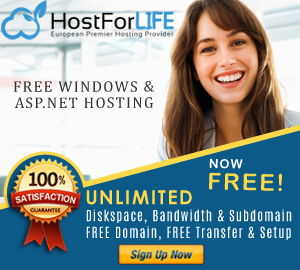
Web.config File
<appSettings>
<add key="Filesize" value="50KB"/>
<add key="ChartImageHandler" value="storage=file;timeout=20;dir=c:\TempImageFiles\;" />
</appSettings>
Asp File
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Try
Response.Clear()
Dim fileid As String = System.Guid.NewGuid.ToString()
Dim imgsize As String = ConfigurationManager.AppSettings("Filesize")
Dim a As String = Context.Request.Files.Get(0).ContentLength.ToString
Dim b As String = Math.Round(a / 1024)
Dim c As String = imgsize.Replace(("KB").ToString, "")
If Val(b) < Val(c) Then
Context.Request.Files.Get(0).SaveAs("D:\manish\Project\Project\upload\" & fileid & ".jpg")
Response.Write("True|" & fileid)
Else
Response.Write("False|Select File Less Then " + imgsize + " || Your File Size is " + b + " KB")
End If
Response.End()
Catch ex As ArgumentOutOfRangeException
MsgBox("'Select A File' Then Click On Upload Button")
End Try
End Sub
JS File
var myApp = angular.module('myApp', ['ui.bootstrap']);
//upload a file code
myApp.directive('fileModel', ['$parse', function($parse) {
return {
restrict: 'A',
link: function(scope, element, attrs) {
var model = $parse(attrs.fileModel);
var modelSetter = model.assign;
element.bind('change', function() {
scope.$apply(function() {
modelSetter(scope, element[0].files[0]);
});
});
}
};
}]);
myApp.service('fileUpload', ['$http', function($http) {
this.uploadFileToUrl = function(file, uploadUrl) {
var fd = new FormData();
fd.append('file', file);
$http.post(uploadUrl, fd, {
transformRequest: angular.identity,
headers: {
'Content-Type': undefined
}
})
.success(function(data) {
if (data.split("|")[0] == "True") {
$("#getimg").attr('src', 'upload/' + data.split("|")[1] + '.jpg');
$("#hid").val(data.split("|")[1] + ".jpg");
var img = $("#hid").val();
if (img == "noimage.jpg") {
$("#Remov").hide();
} else {
$("#Remov").show();
}
} else if (data.split("|")[0] == "False") {
alert(data.split("|")[1]);
var ab = $("#hid").val();
$("#getimg").attr('src', 'upload/' + ab);
var img = $("#hid").val();
if (img == "noimage.jpg") {
$("#Remov").hide();
} else {
$("#Remov").show();
}
}
})
.error(function() {
if ($(myFile).val() == "") {
alert("Select a file");
} else {
alert("Select a image file");
}
});
}
}]);
$scope.uploadFile = function() {
var fileval = ['jpeg', 'jpg', 'png', 'gif', 'bmp'];
if ($(myFile).val() == "") {
alert("Select a file");
} else if ($.inArray($(myFile).val().split('.').pop().toLowerCase(), fileval) == -1) {
alert("Select a image file");
$(myFile).val('');
$("#hid").val("noimage.jpg");
} else {
var file = $scope.myFile;
var uploadUrl = "Image_Upload.aspx";
fileUpload.uploadFileToUrl(file, uploadUrl);
$scope.myFile = "";
$scope.remove = true;
var reader = new FileReader();
reader.onload = function(e) {
scope.image = e.target.result;
scope.$apply();
}
}
elem.on('change', function() {
reader.readAsDataURL(elem[0].files[0]);
});
return false;
};
I hope this post wil works for you. Good luck!
AngularJS with Free ASP.NET Hosting
Try our AngularJS with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
