
November 29, 2024 08:39 by
Peter
In this walk, you will learn about running and calling javascript functions from the Angular component.
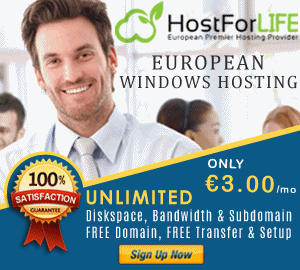
How it’s implemented and what is the description?
- Create Angular project - AnguWalk.
- Run the application and check that the project was created successfully.
- Create JS file with function.
- Adding JS file link/reference to angular.json
- Component (TS file) coding.
- Execute the Project
- Output
Create an Angular Project called “AnguWalk” using the following CLI command.Commandng new AnguWalkBashExample
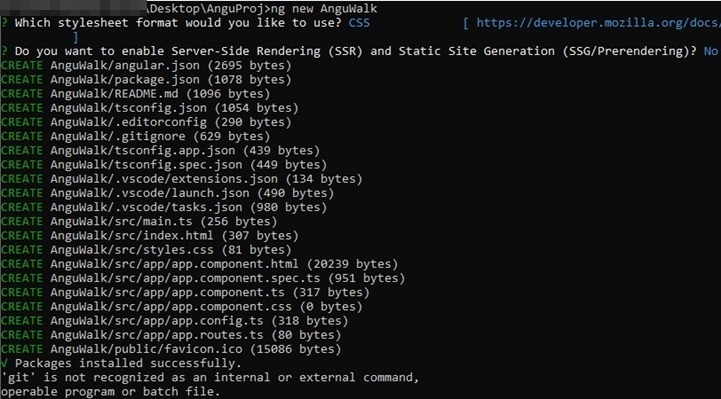
Go inside the project folder and open the Visual Studio code.
Command
cd anguwalk
<enter>
code .
<enter>
Example

Note. Visual Studio code will get started only if your system is configured with path and settings.
Create Javascript file
- First, create a JS folder inside the SRC folder.
- Right-click on SRC and select the option New Folder.
function HelloMsg(arg) {
alert("Welcome to Angular Class - " + arg);
}
Reference JS File in angular.json file
The Angular.json file is located inside the project root folder. Open and update the SCRIPTS array which is inside the builds object.
"scripts": [
"src/js/WelcomeMsg.js"
]
Open app.component.html and update the code.
Note. Remove the default code of app.component.html.
import { Component, OnInit } from '@angular/core';
import { RouterOutlet } from '@angular/router';
declare function HelloMsg(arg: any): void;
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet],
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
ngOnInit() {
HelloMsg("Peter, Scott");
}
title = 'AnguWalk';
}
Now, run the project to check the output.
Command
ng serve --open
Output
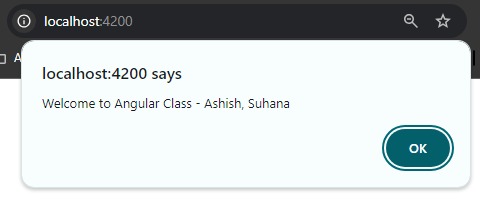
You see the javascript HelloMsg function executed and the alert window displayed successfully. Happy Coding!