
September 8, 2021 08:32 by
Peter
Angular provides two approaches,
Reactive-driven forms
Template-driven forms
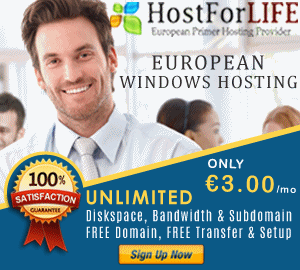
Understanding the difference between template and reactive forms
Template driven forms are asynchronous while, reactive forms are synchronous
In the Reactive driven approach, most of the logic is from the components whereas, in the template-driven approach most of the logic resides in the template.
When to use template-driven approach
When no complex validations are required in the form.
When you migrate from angular js to angular 2+, it’s easier to consider this approach
When to use reactive driven approach
When form, becomes the key part of the app we must use a reactive-driven approach as it is predictable, reusable, testable.
How to build a reactive form in 4 steps
We need to import ReactiveFormMoudle in the root folder,
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { ReactiveFormsModule } from '@angular/forms';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
ReactiveFormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Create form module in the component using - FormGroup, FormControl, and FormArrays
In the reactive form, we will use FormGroup, FormControl, FormArray as they are the building block of an angular form.
First, let us try to understand what does those terminology means in simple words
FormControl - It is a class that is used to get and set the value and validate the form control.
FormGroup and FormArray - It represents the collections of form controls.
Now we need to go to app.compotents.ts and import FormGroup, FormControl & validator from the @angular/forms
import { FormGroup, FormControl, Validators } from '@angular/forms'
The next step is to create a FormGroup and Add FormControl inside the FormGroup.
schoolForm = new FormGroup({})
As you can see, we have an instance for form group and named it as schoolForm.
Under the schoolForm, we have three formControl instances representing the properties' first name, last name, country.
schoolForm = new FormGroup({
firstname: new FormControl(),
lastname: new FormControl(),
country: new FormControl()
})
Building of HTML form
You would have noticed that we have enclosed it in a <form> and included three input felids first name, last name, country.
<form [formGroup]=" schoolForm " (ngSubmit)="onSubmit()">
<p>
<label for="firstname">First Name </label>
<input type="text" id="firstname" name="firstname" formControlName="firstname" >
</p>
<p>
<label for="lastname">Last Name </label>
<input type="text" id="lastname" name="lastname" formControlName=" lastname " >
</p>
<p>
<label for="country">country </label>
<input type="text" id=" country " name=" country " formControlName=" country " >
</p>
<p>
<button type="submit">Submit</button>
</p>
</form>
To connect the model and the template, add the following,
<form [formGroup]="schoolForm">
Next, we need to bind form fields to the FormControl models and use the formControlName directive.
<input type="text" id="firstname" name="firstname" formControlName="firstname" >
Lastly, we have to submit the form
(ngSubmit)="onSubmit()"
We have already got the submit button in our form. The ngSubmit binds itself to the click event of the submit button.
Get data in the Component class
To receive the form data in the component class. We have to create the onSubmit method in the component class.
onSubmit() {
console.log(this. schoolForm.value);
}
We are using the,
console.log(this.contactForm.value)
To send the value of our form data to the console window.
I hope you understand about forms and how to use the reactive form in angular.