
May 11, 2022 09:02 by
Peter
This article provides a sample application using the Redis Cache with explanation. Please see this article on how to create an Express Node JS application, so that we can quickly jump into the topic without further delay.
Why is Caching required?
When the same response needs to be served multiple times, store the data in distributed server memory as and it can be retrieved faster than the data retrieved from the storage layer for every single call. Caching is an ability of an application to duplicate the values for a specified period of time and serve the web request instantly.
These techniques will be effective when
There is a need to call for a 3rd party API and the call counts
Cost of data transfer between cloud and the server.
Server response is critical based on the concurrent requests.
What is Redis Cache?
The data cache stored in a centralized distributed system such as Redis. Redis is also called a data structures server, which means a variety of processes can query and modify the data at the same time without much loading time.
Advantages of Redis
The special properties of data structures are
Even though the data is often requested, served and modified, the data will be stored in disk rather than storing in RAM.
Unlike high-level programming language, the implementation will highlight on memory optimal usage.
Also offers features like replication, levels of durability, clustering and high availability (HA).
Redis can handled complexed Memcached operations like Lists, Sets, ordered data set, etc.,
Using Redis Client in local system
To use the Redis, you need to install Node JS Redis Client in the local system. The msi file (v3.0.504 stable version as of this article published date) can be downloaded from the Github and proceed with normal installation process (for Windows OS).
The latest Redis Client can be also be installed with Windows Subsystem for Linux (WSL) and install the Redis 6.x versions. Also, for dockerizing, Mac and Linux OS’ follow the instructions on downloads
Run the Redis Client by the following command
redis-server
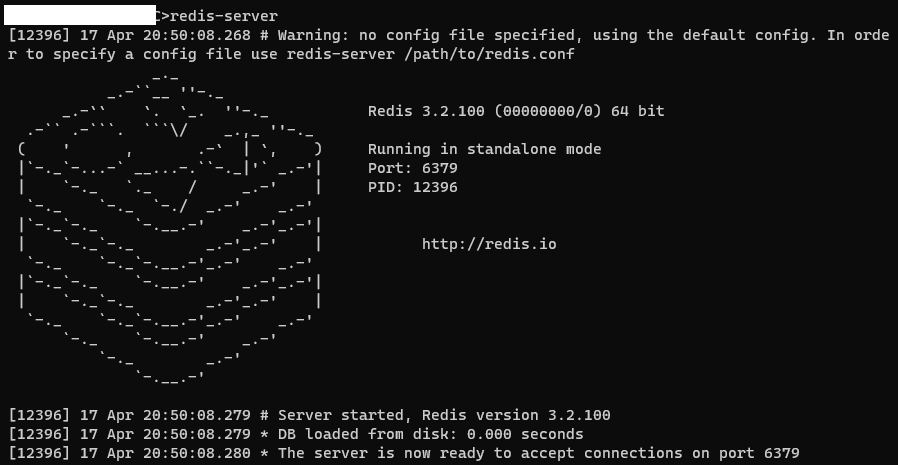
Create an Express Node JS application
Open the PowerShell or cmd prompt window and go to the destined folder.
Execute the following command
npx express expressRedisCaching –hbs

Install the modules using the command
npm i
and install the following additional packages
npm i redis
npm i isomorphic-fetch
Once all the packages are installed and ready to start. I have created a new route page called “getCached.js” and mapped in the “App.js” page.
app.use('/getCached', require('./routes/getCached'));
Upon calling the endpoint, the logic is to fetch the response from JSON placeholder (mock API) site, store the data in Redis Cache and respond to the request. On further request, cached data will be served.
const express = require('express')
require('isomorphic-fetch')
const redis = require('redis')
const router = express.Router()
const client = redis.createClient({
host:'127.0.0.1',
port:6379
})
router.get('/',async(req, res)=>{
await client.connect();
const value = await client.get('todos');
if(value){
console.log("from cached data")
res.send(JSON.parse(value))
}
else{
const resp = await fetch(sourceURL)
.then((response) => response.json());
await client.set('todos', JSON.stringify(resp));
console.log("from source data")
res.send(resp);
}
await client.disconnect();
})
module.exports = router
Object created using the redis is
redis.createClient({ host:’127.0.0.1’, port:6379});
With options
host : currently the Redis is available in the local system and so the host is default 127.0.0.1
port : by default Windows OS is allocating 6379 port to Redis, but the same can be customized.
Now it’s time to run the app by executing the command
npm start
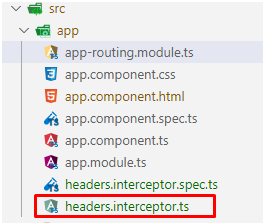
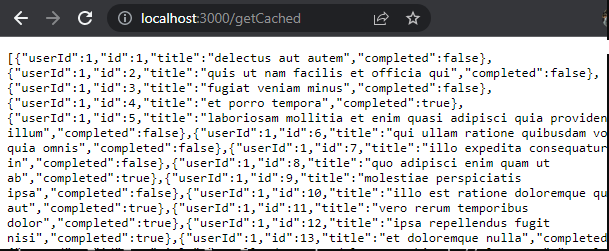
On the first hit, the data is fetched from the source API with the response time of approximately 1156ms and the same has been stored in the in-memory data store Redis Cache. The second hit gets the data from the cached with the remarkable reduction in fetch time of just ~10 ms or even ~6ms.
Thus, the objective is met with very few easy steps.