HTTP Interceptor
HTTP Interceptors is a special type of angular service that we can implement. It's used to apply custom logic to the central point between the client-side and server-side outgoing/incoming HTTP request and response. Keep in mind that the interceptor wants only HTTP requests.
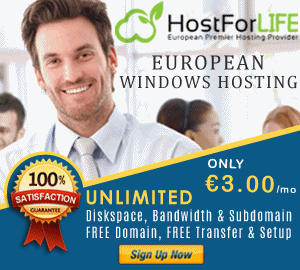
Operations of HTTP Interceptor
Modify HTTP headers
Modifying the request body
Set authentication/authorization token
Modify the HTTP response
Error handling
Add HTTP Interceptor
Execute this CLI command to add an interceptor service: ng generate interceptor headers

Successfully added headers interceptor
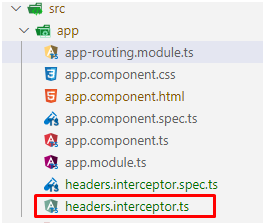
After opening the interceptor service you can see a default view like this:
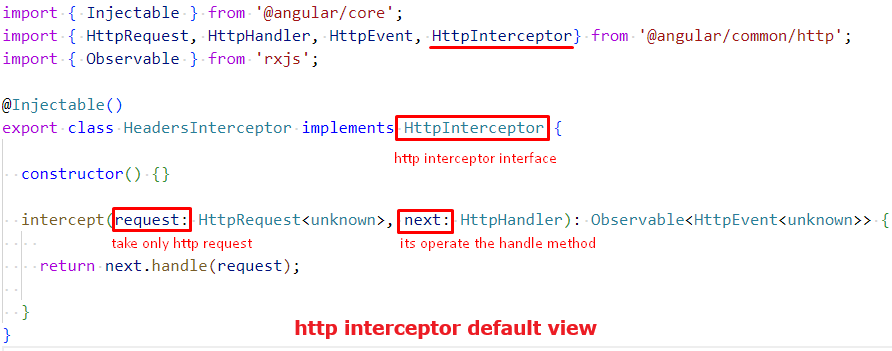
Interceptor registration
We need to register the HTTP interceptor to use in the application. So, open the app.module.ts file and go to the provider section.
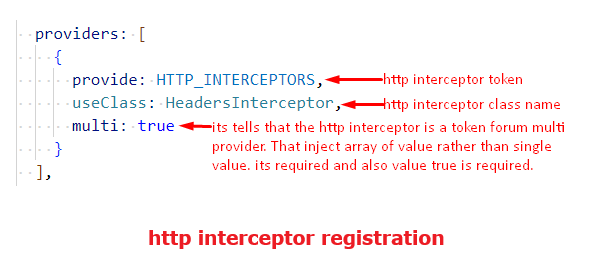
This interceptor will execute for every HTTP request of a client.
Modifying HTTP Headers By interceptor
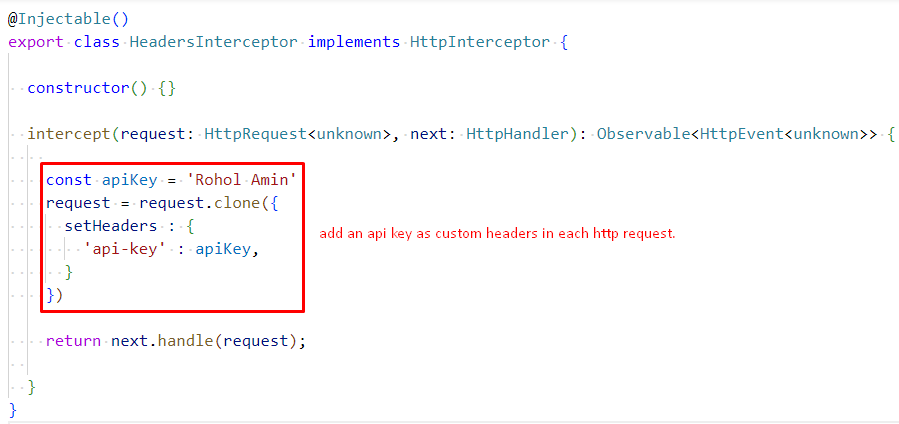
const apiKey = 'Rohol Amin'
request = request.clone({
setHeaders: {
'api-key': apiKey,
}
})
Now serve the project. Open the browser inspect window then go to the Network tab and click the load data button. Then click the todos API of xhr type. Then go to the HTTP request headers,
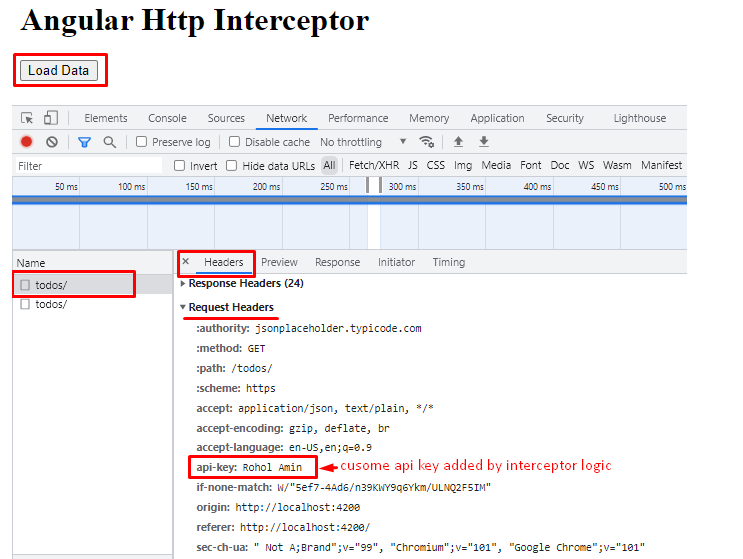
Multiple interceptors
It is also possible to use multiple interceptors simultaneously. Just need to maintain the serial which service will be executed before and after. This serialization is defined in provider registration. Meaning the interceptor service will execute according to the provider registration serialization.
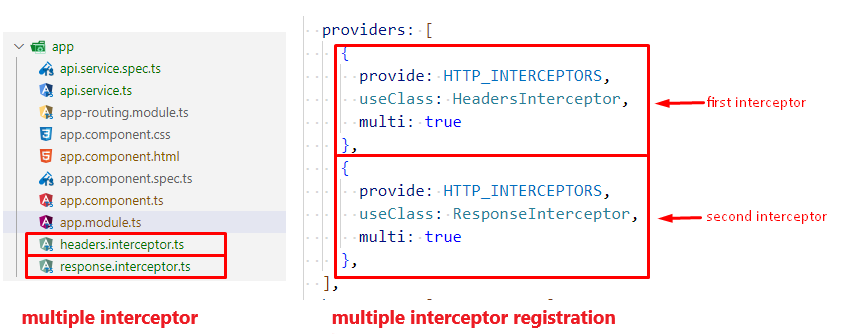
{
provide: HTTP_INTERCEPTORS,
useClass: ResponseInterceptor,
multi: true
}
Response Interceptor Implementation
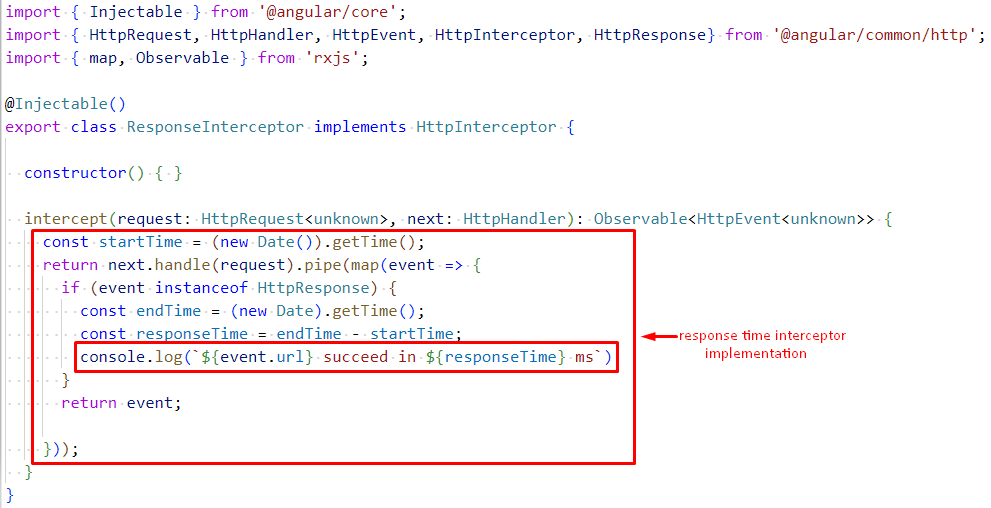
const startTime = (new Date()).getTime();
return next.handle(request).pipe(map(event => {
if (event instanceof HttpResponse) {
const endTime = (new Date).getTime();
const responseTime = endTime - startTime;
console.log(`${event.url} succeed in ${responseTime} ms`)
}
return event;
}));
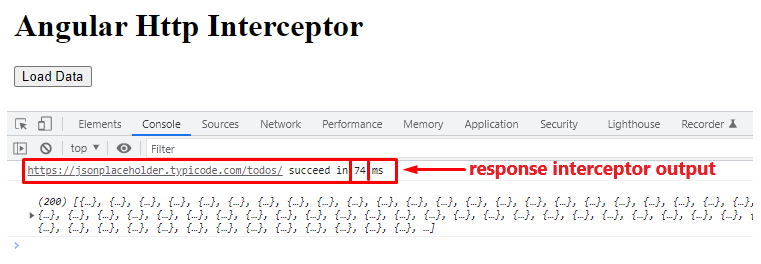
Note
This HTTP interceptor is most used when authentication and authorization are applied in the application.
Hope this article would have helped you to understand about Angular Interceptor. Here I have tried to explain very simply some uses of the angular interceptor. Happy coding and thanks for reading my article!!!