
August 21, 2023 08:54 by
Peter
RxJS (Reactive Extensions for JavaScript) is a sophisticated tool used in Angular applications for handling asynchronous and event-based programming with observables. RxJS provides a diverse set of operators for manipulating, transforming, combining, and managing observables in a flexible and functional manner. These operators facilitate reactively working with data streams and asynchronous processes.
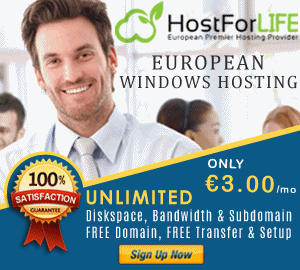
Here's a rundown of some of the most common RxJS operators and how they can be utilized in Angular:
map
The map operator is used to transform observable values into new ones. This is frequently used to do data transformations.
import { map } from 'rxjs/operators';
observable.pipe(
map(data => data * 2)
).subscribe(result => console.log(result));
filter
The filter operator is used to remove values from an observable depending on a criterion.
import { filter } from 'rxjs/operators';
observable.pipe(
filter(data => data > 5)
).subscribe(result => console.log(result));
mergeMap (flatMap)
The mergeMap operator is used to merge multiple observables into a single observable by applying a function to each emitted value and flattening the resulting observables.
import { mergeMap } from 'rxjs/operators';
observable.pipe(
mergeMap(data => anotherObservable(data))
).subscribe(result => console.log(result));
switchMap
The switchMap the operator is similar to mergeMap, but it switches to a new inner observable whenever a new value is emitted from the source observable, canceling the previous inner observable.
import { switchMap } from 'rxjs/operators';
observable.pipe(
switchMap(data => anotherObservable(data))
).subscribe(result => console.log(result));
debounceTime
The debounceTime operator delays emitted values for a specified amount of time and only emits the last value within that time frame.
import { debounceTime } from 'rxjs/operators';
inputObservable.pipe(
debounceTime(300)
).subscribe(value => console.log(value));
combineLatest
The combineLatest operator combines the latest values from multiple observables whenever any of the observables emit a new value.
import { combineLatest } from 'rxjs/operators';
combineLatest(observable1, observable2).subscribe(([value1, value2]) => {
console.log(value1, value2);
});
catchError
The catchError operator is used to handle errors emitted by an observable by providing an alternative observable or value.
import { catchError } from 'rxjs/operators';
import { of } from 'rxjs';
observable.pipe(
catchError(error => of('An error occurred: ' + error))
).subscribe(result => console.log(result));
These are just a few examples of the many RxJS operators available. Using RxJS operators effectively can help you manage complex asynchronous workflows, handle data transformations, and create more responsive and reactive Angular applications.
Happy Learning :)