
September 6, 2024 09:21 by
Peter
In contemporary web development, integrating many libraries and frameworks is frequently necessary to provide a seamless user experience. Combining AngularJS with jQuery Select2 to improve dropdown menus is one typical use case. This post looks at how to properly handle dropdown changes and synchronize AngularJS models with Select2.
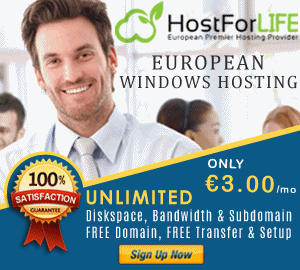
Overview
For creating dynamic online applications, AngularJS is a strong framework. On the other hand, jQuery Select2 is a well-liked library that offers improved dropdown menu features like searching, tagging, and more. By combining these technologies, a strong user interface with cutting-edge features can be produced.
Setting up the Environment
First, ensure you have included the necessary libraries in your project.
- AngularJS: For data binding and MVC functionality.
- jQuery: Required by Select2 for DOM manipulation.
Select2: For advanced dropdown functionality
code
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.5/angular.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.3/js/select2.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.3/css/select2.min.css" />
<div class="col-md-3 mt-3">
<label class="text-muted font-weight-bold">demoDis*</label>
<select id="txtID" name="txtID" class="form-control select2" ng-model="Refundval" ng-options="lobjdata.Value as lobjdata.Name for lobjdata in ListMaster">
<option value="" disabled selected hidden>Select</option>
</select>
<input type="hidden" id="hdRefundID" ng-model="hdRefundID" value="" />
</div>
app.controller('MyController', function($scope) {
// Function to initialize Select2 and handle changes
$scope.demoBan = function() {
debugger;
var Reta = JSON.parse(localStorage.getItem("Get"));
console.log("RetailerId", RetailerId);
$scope.ListMaster = Reta;
angular.element(document).ready(function() {
// Initialize Select2
$('#txtID').select2();
$('#txtID').on('change.select2', function() {
var selectedValue = $(this).val();
var selectedText = $('#txtID option:selected').text(); // Get the text of the selected option
$scope.$applyAsync(function() {
// Update AngularJS model
$scope.Id = selectedValue;
$scope.Text = selectedText.trim(); // Store the text of the selected option
console.log("Selected Value:", selectedValue);
console.log("Selected Text:", $scope.Text);
});
});
});
};
});
Explanation
- Initialization: Use angular. element(document).ready() to ensure that the DOM is fully loaded before initializing Select2.
- Handling Changes: Bind to Select2’s change event to capture user selections. Use $scope.$applyAsync() to update AngularJS models and ensure the changes are reflected in the view.
Conclusion
Integrating AngularJS with Select2 can significantly enhance the user experience by combining Angular’s data binding capabilities with Select2’s advanced dropdown features. By following the steps outlined, you can effectively synchronize models between AngularJS and Select2, providing a seamless interaction for your users.