Dependency Injection (DI) is a powerful front-end framework developed by Google that makes it easy to manage services and components inside an application. Dependencies can be received by components instead of being created directly thanks to DI, a design paradigm that encourages loose coupling. In order to better understand DI in Angular, this post will go through its core ideas, benefits, and real-world application using code samples.
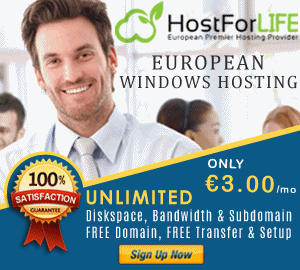
Injecting Dependency in Angular
A software design technique called dependency injection makes it possible to create easily maintained, loosely linked components. DI is a method used with Angular that allows a component to obtain its dependencies from outside sources instead of generating them on its own. The Angular Injector, which is in charge of instantiating and maintaining the dependencies of different components, is usually this external source.
Dependency Injection's Advantages in Angular
- Loose Coupling: DI encourages loose coupling, which makes it simpler to update or replace dependencies without impacting the whole program.
- Testability: Dependencies can be easily injected to mock or test during unit testing, making DI-patterned components more testable.
- Reusability: DI makes services and components more reusable because it makes it simple to inject them into various application sections without requiring any changes.
- Maintainability: The codebase is more maintainable because to the concern separation made possible by DI. It is less probable for changes made to one section of the application to affect other sections.
How Dependency Injection Operates in Angular?
The Angular Injector is used in Angular to construct the DI system. In order to control the scope of dependencies, the Injector upholds a hierarchical structure while generating instances of components, services, and other objects.
Here's a quick example to help you grasp how DI functions in Angular. Think about a service known as DataService.
// data.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class DataService {
getData(): string {
return 'This is data from the DataService';
}
}
To indicate that the DataService in this example is a service that may be injected into other components or services, the @Injectable decorator is used. Throughout the application, there will only be one instance of DataService thanks to the providedIn: 'root' setting.
Let's use this service to create an AppComponent component now.
// app.component.ts
import { Component } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
template: '<h1>{{ data }}</h1>',
})
export class AppComponent {
data: string;
constructor(private dataService: DataService) {
this.data = this.dataService.getData();
}
}
In this example, the AppComponent class has a constructor that takes an instance of DataService as a parameter. The Angular Injector automatically injects the appropriate instance when creating an AppComponent.
import { Component, Injector } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-root',
template: '<h1>{{ data }}</h1>',
})
export class AppComponent {
data: string;
constructor(private injector: Injector) {
const dataService = this.injector.get(DataService);
this.data = dataService.getData();
}
}
In this example, we inject the Injector itself and then use it to manually get an instance of the DataService. While manual injection is rarely needed, it provides a way to have more control over the injection process.
In summary
A key idea in Angular that enhances the framework's adaptability, testability, and maintainability is dependency injection. You may build scalable and modular Angular applications by comprehending DI and applying it into your application architecture. The hierarchical structure enables for fine-tuning the scope of dependencies, while the automatic injection offered by the Angular Injector makes dependency management easier.
Adopting and understanding Dependency Injection will definitely improve the overall architecture and code quality as you continue to develop Angular applications.