
December 13, 2023 06:14 by
Peter
This post will teach us how to use an Angular application to design a custom search filter pipe.
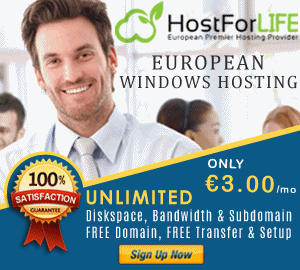
Required conditions
Basic familiarity with Angular 2 or later; installation of Node and NPM; Visual Studio Code; Bootstrap (Optional)
The command to create an Angular project is as follows.
ng new angapp
Now install Bootstrap by using the following command.
npm install bootstrap --save
Now open the styles.css file and add Bootstrap file reference. To add a reference in the styles.css file add this line.
@import '~bootstrap/dist/css/bootstrap.min.css';
Create Search Filter Pipe
Now create a custom pipe by using the following command.
ng generate pipe searchfilter
Now open searchfilter.pipe.ts file and add following code.
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({ name: 'searchFilter' })
export class FilterPipe implements PipeTransform {
transform(value: any, args?: any): any {
if (!value) return null;
if (!args) return value;
args = args.toLowerCase();
debugger;
return value.filter(function(item:any) {
return JSON.stringify(item)
.toLowerCase()
.includes(args);
});
}
}
Now import this pipe into the app module.Add following code in app.module.ts.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { HttpClientModule } from '@angular/common/http';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { FilterPipe } from './searchfilter.pipe';
import { SearchlistComponent } from './searchlist/searchlist.component';
@NgModule({
declarations: [
AppComponent,FilterPipe,SearchlistComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Now create a new component by using the following command.
ng g c searchlist
Now open searchlist.component.html file and add the following code.
<div class="container">
<div class="card">
<div class="form-group">
<label for="search-text">Search Text</label>
<input type="email" class="form-control" id="search-text" aria-describedby="search-text"
[(ngModel)]="searchText" placeholder="Enter text to search" autofocus>
</div>
<ul class="list-group list-group-flush">
<li class="list-group-item" *ngFor="let user of userlist | searchFilter: searchText">
{{user.firstName}}
</li>
</ul>
</div>
</div>
Now open searchlist.component.ts file and add the following code.
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-searchlist',
templateUrl: './searchlist.component.html',
styleUrls: ['./searchlist.component.css']
})
export class SearchlistComponent implements OnInit {
data: any;
searchText = '';
userlist: any;
constructor(private httpclient: HttpClient) {
}
ngOnInit(): void {
this.httpclient.get('https://dummyjson.com/users').subscribe((res: any) => {
this.data = res
this.userlist = this.data.users;
console.log(this.userlist);
})
}
}
Now open app.component.html file and add the following code.
<div class="container" style="margin-top:10px;margin-bottom: 24px;">
<div class="col-sm-12 btn btn-info">
Create Custom Search filter pipe in Angular Application
</div>
</div>
<app-searchlist></app-searchlist>
Now run the application using npm start and check the result.
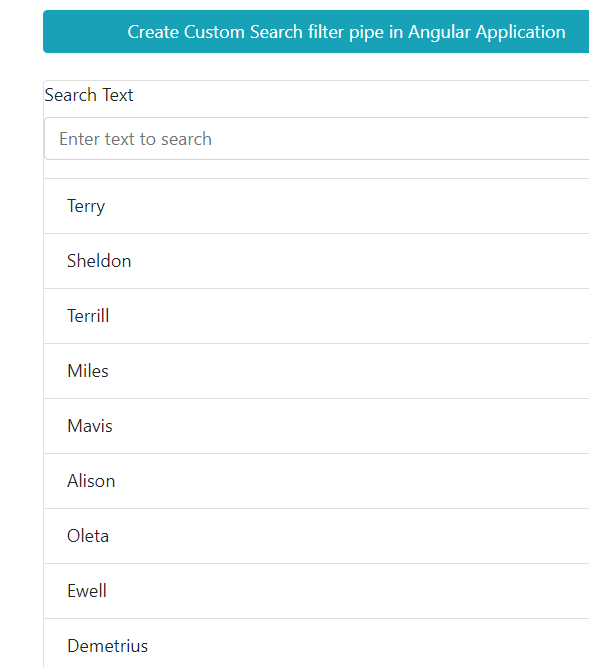
Now type some text into the box and run a search.