
August 8, 2023 08:27 by
Peter
In this article, we will go over the fundamentals of observable and subject. The distinction between them is further demonstrated through practical examples and the various types of subjects.
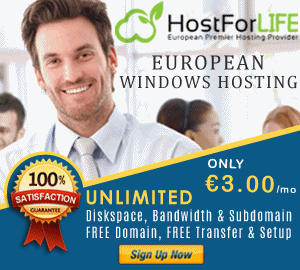
What exactly is Angular?
Angular is a popular open-source JavaScript web application framework. It was created by Google and is presently maintained by the Google Angular Team. Angular enables developers to create dynamic, single-page applications (SPAs) and offers a disciplined way to developing complicated online apps.
Visible in Angular
- Observables are a component of the Reactive Extensions for JavaScript (RxJS) library in Angular.
- Observables allow you to send messages between components of your program.
- Observables are a useful tool in reactive programming that is widely used to handle asynchronous actions and data streams.
- Observables allow you to subscribe to and get notifications when new data or events are emitted, allowing you to react in real-time to changes.
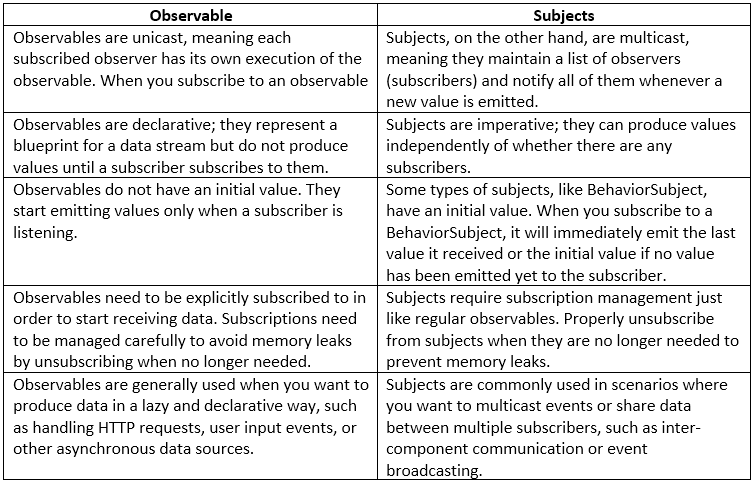
//------------------Observables are unicast-----------------
//observable
let observable = new Observable<number>(ele =>
ele.next(Math.random()))
//first subscriber
observable.subscribe(result => {
this.first_subscriber_observable = result;
console.log(result)
})
//second subscriber
observable.subscribe(result => {
this.second_subscriber_observable = result;
console.log(result)
})
//third subscriber
observable.subscribe(result => {
this.thrid_subscriber_observable = result;
console.log(result)
})
//--------------------------------------------------------
//------------------Subjects are multicast-----------------
//subject
let subject = new Subject<number>()
//first subscriber
subject.subscribe(result => {
this.first_subscriber_subject = result;
console.log(result)
})
//second subscriber
subject.subscribe(result => {
this.second_subscriber_subject = result;
console.log(result)
})
//third subscriber
subject.subscribe(result => {
this.third_subscriber_subject = result;
console.log(result)
})
subject.next(Math.random())
//--------------------------------------------------------
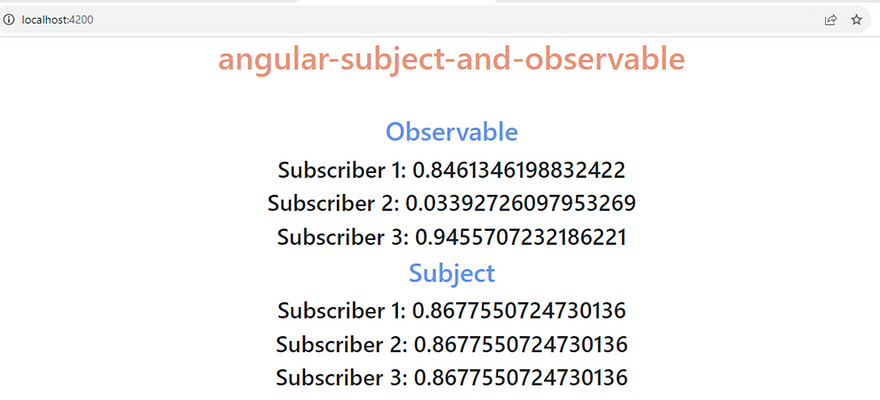
2. BehaviorSubject
The behavior subject is another type of subject in RxJS.
It has an initial value and will immediately emit the initial value to any subscriber as soon as they subscribe, even if no values have been emitted yet using the next() method.
After the initial value is emitted, it behaves like a regular Subject and notifies subscribers about new values emitted using next().
This is useful when you want to provide the last known value to new subscribers, such as the current state of an application or the latest data fetched from an API.
//----------Behavior Subject has default or last emitted value---------------
var behaviorSubject = new BehaviorSubject<number>(123)
behaviorSubject.subscribe(ele => {
this.first_subscriber_behaviorSubject = ele
console.log(`first subscriber ${ele}`)
})
behaviorSubject.next(456)
behaviorSubject.subscribe(ele => {
this.second_subscriber_behaviorSubject = ele
console.log(`second subscriber ${ele}`)
})
//--------------------------------------------------------------------------

3. ReplaySubject
The ReplaySubject is a subject that can buffer and replay a specific number of values to new subscribers.
When you create a ReplaySubject, you can specify the buffer size, which determines how many previous values should be replayed to new subscribers.
This is useful when you want to provide a history of values to new subscribers or when you need to cache values for later use.
//---------------Replay subject buffers old values not default one-----------
const replaySuject = new ReplaySubject(2) //If we we want to show only last 2 buffered value otherwise it will show all
replaySuject.next(111)
replaySuject.next(222)
replaySuject.next(333)
replaySuject.subscribe(e => {
console.log(`First Subscriber ${e}`)
this.first_subscriber_replaySubject.push(e);
})
//new values show to existing subsriber
replaySuject.next(444)
replaySuject.subscribe(e => {
console.log(`Second Subscriber ${e}`)
this.second_subscriber_replaySubject.push(e);
})
replaySuject.next(555)
//--------------------------------------------------------------------------
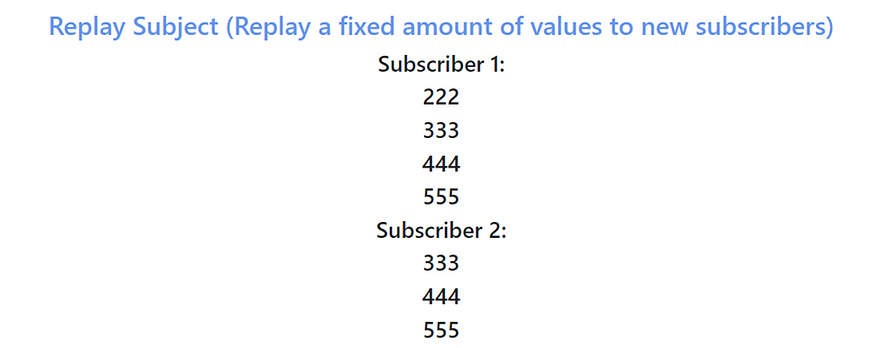
4. AsyncSubject
- The AsyncSubject is a subject that only emits the last value when it completes.
- It will not emit any values until the subject’s complete() method is called. When completed, it will emit the last value (if any) to subscribers.
- This is useful when you need to wait for an operation to complete before emitting a final value, such as waiting for an HTTP request to finish and emitting the response as a single value.
//---------------Async subject sends the latest value to subscribers when it's completed-----------
const asyncSubject = new AsyncSubject<number>();
asyncSubject.subscribe(e =>
{
console.log(`First Subscriber: ${e}`)
this.first_subscriber_asyncSubject=e;
});
asyncSubject.next(111);
asyncSubject.next(222);
asyncSubject.next(333);
asyncSubject.next(444);
asyncSubject.subscribe(e => {
console.log(`Second Subscriber: ${e}`)
this.second_subscriber_asyncSubject=e;
});
asyncSubject.next(555);
asyncSubject.complete();
//--------------------------------------------------------------------------
