
July 25, 2024 07:31 by
Peter
Working with APIs is a standard chore in modern web development. Angular's HttpClient module offers a reliable method for managing HTTP requests. You will learn how to create an Angular service that will call an API and manage the responses in this tutorial.
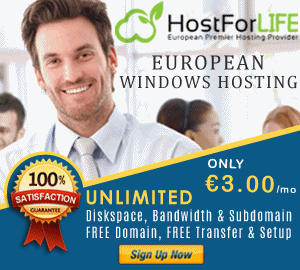
Configuring Angular Project
Make sure you have Angular CLI installed before we begin. If not, you can use the following command to install it.
npm install -g @angular/cli
Create a new Angular project.
ng new api-service-demo
cd api-service-demo
Add HttpClientModule to your project.
ng add @angular/common/http
Creating the Service
Generate a new service using Angular CLI.
ng generate service api
This command will create a file named api.service.ts in the src/app directory.
Importing HttpClient
First, import HttpClient and HttpClientModule in your app.module.ts.
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
// Your components
],
imports: [
BrowserModule,
HttpClientModule,
// Other modules
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Implementing the API Call in the Service
Next, implement the API call in your service (api.service.ts).
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class ApiService {
private apiUrl = 'https://jsonplaceholder.typicode.com/posts'; // Replace with your API URL
constructor(private http: HttpClient) { }
getPosts(): Observable<any> {
return this.http.get<any>(this.apiUrl);
}
}
In this example, getPosts is a method that sends a GET request to the specified API URL and returns an Observable of the response.
Using the Service in a Component
Now, let's use this service in a component to fetch data and display it. Generate a new component.ng generate component post-list
Injecting the Service
Inject the ApiService in your post-list.component.ts.import { Component, OnInit } from '@angular/core';
import { ApiService } from '../api.service';
@Component({
selector: 'app-post-list',
templateUrl: './post-list.component.html',
styleUrls: ['./post-list.component.css']
})
export class PostListComponent implements OnInit {
posts: any[] = [];
constructor(private apiService: ApiService) { }
ngOnInit(): void {
this.apiService.getPosts().subscribe(data => {
this.posts = data;
});
}
}
Displaying Data in the TemplateUpdate the template (post-list.component.html) to display the fetched data.<div *ngIf="posts.length > 0">
<h2>Posts</h2>
<ul>
<li *ngFor="let post of posts">
<h3>{{ post.title }}</h3>
<p>{{ post.body }}</p>
</li>
</ul>
</div>
<div *ngIf="posts.length === 0">
<p>No posts available.</p>
</div>
Running the Application
Serve the Angular application using the following command.
ng serve
Navigate to http://localhost:4200 in your browser to see the list of posts fetched from the API.
Conclusion
In this article, we've covered how to make API calls in Angular using a service. We created a service to handle HTTP requests and used this service in a component to fetch and display data. This approach ensures that your API logic is centralized and reusable across different components.