
August 1, 2024 08:52 by
Peter
Web applications frequently need users to copy text to the clipboard in order to share links, copy codes, or accomplish other tasks. We will look at how to add a copy-to-clipboard feature to an AngularJS application in this article.
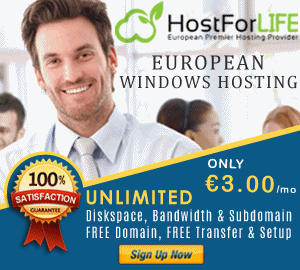
Required conditions
You need know the fundamentals of JavaScript and AngularJS in order to follow along with this lesson.
Step 1: Configuring the AngularJS Program
Let's start by configuring a basic AngularJS application. Ensure that your HTML file has the AngularJS library.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Copy to Clipboard in AngularJS</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body ng-app="clipboardApp" ng-controller="clipboardController">
<!-- Your AngularJS app content will go here -->
</body>
</html>
Step 2. Creating the AngularJS Module and Controller
Next, let's create an AngularJS module and a controller.
<script>
var app = angular.module('clipboardApp', []);
app.controller('clipboardController', ['$scope', function($scope) {
$scope.textToCopy = "Hello, this is the text to be copied!";
$scope.copyToClipboard = function() {
var textArea = document.createElement("textarea");
textArea.value = $scope.textToCopy;
document.body.appendChild(textArea);
textArea.select();
try {
document.execCommand('copy');
alert('Text copied to clipboard');
} catch (err) {
console.error('Unable to copy', err);
}
document.body.removeChild(textArea);
};
}]);
</script>
Step 3. Adding the HTML Elements
Now, let's add the HTML elements to allow users to copy the text.
<div class="container">
<h1>Copy to Clipboard Example</h1>
<div class="form-group">
<label for="textToCopy">Text to Copy:</label>
<input
type="text"
id="textToCopy"
ng-model="textToCopy"
class="form-control"
readonly
>
</div>
<button
ng-click="copyToClipboard()"
class="btn btn-primary"
>
Copy to Clipboard
</button>
</div>
Explanation
- Creating a Textarea Element: In the copy to clipboard function, we create a textarea element dynamically and set its value to the text we want to copy.
- Appending Textarea to Body: The textarea is appended to the body of the document to ensure it is part of the DOM.
- Selecting the Text: We then select the text inside the text area.
- Copying the Text: Using document.execCommand('copy'), we copy the selected text to the clipboard.
- Removing the Textarea: Finally, we remove the textarea element from the document.
Step 4. Styling the Application
You can add some CSS to style the application.
<style>
.container {
margin: 50px;
}
.form-group {
margin-bottom: 20px;
}
.btn-primary {
background-color: #007bff;
border-color: #007bff;
}
</style>
Example 1
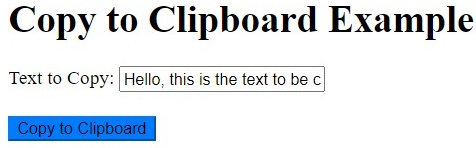
Conclusions
You can incorporate copy-to-clipboard capabilities into your AngularJS application with ease by following these steps. This feature makes it easier for users to copy text without having to pick and paste it by hand, which improves user experience. Play around with this implementation and adjust it to suit the requirements of your application.