
May 19, 2015 10:31 by
Peter
In this tutorial, I will explain you how to make facebook style autocomplete in AngulaJS with MVC. First step, you must include the following libraries, AngularJS and ui-bootstrap-tpls.
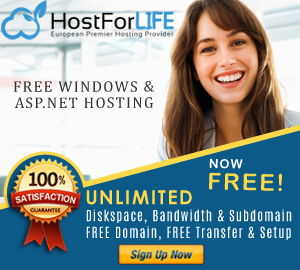
After that, create a new ASP.NET MVC project and then Add a Home Controller. Add a Index view. Now, write the following code to your index view:
<html ng-app="myApp">
<head>
<script src="~/Scripts/angular.min.js"></script>
<script src="~/Scripts/ui-bootstrap-tpls.min.js"></script>
<script src="~/Scripts/AutoCompleteDemo.js"></script>
<link href="~/Css/bootstrap.min.css" rel="stylesheet" />
</head>
<body>
<div class="container">
<div ng-controller="myCtrl" class="row-fluid">
<form class="row-fluid">
<div class="container-fluid">
Code <input type="text" ng-model="selectedCode" />
City <input type="text" typeahead-on-select="setcode($item)" ng-model="selected" typeahead="Cityx.CityName for Cityx in City | filter:$viewValue" />
</div>
</form>
</div>
</div>
</body>
</html>
Now, binding typeahead directive to our input field. Make a js file and name it as AutoCompleteDemo.
Write the below code to it:
angular.module('myApp', ['ui.bootstrap'])
.controller("myCtrl", function ($scope) {
$scope.selected = '';
$scope.City = [
{ code: 'AL', CityName: 'Alabama' },
{ code: 'ID', CityName: 'Idaho' },
{ code: 'CA', CityName: 'California' },
{ code: 'NV', CityName: 'Nevada' },
{ code: 'NY', CityName: 'New York' },
{ code: 'FL', CityName: 'Florida' },
{ code: 'KS', CityName: 'Kansas' },
{ code: 'OH', CityName: 'Ohio' },
{ code: 'TX', CityName: 'Texas' },
];
$scope.setcode = function (selection) {
$scope.selectedCode = selection.code;
};
});
In above code.
a) First Inject the dependency on ui.bootstrap module.
b) Create sample list of city with their code to see AutoComplete.
AngularJS with Free ASP.NET Hosting
Try our AngularJS with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
