
May 27, 2015 08:08 by
Peter
Now, I am going to tell you about How to Filtering and Sorting HTML Table Data With AngularJS. There should be three cascading dropdowns to filter the data in the table. You should also be able to sort the table. And here is the following code:
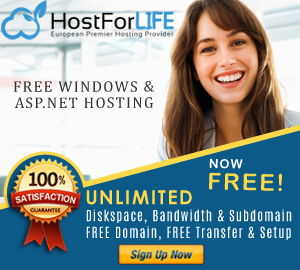
angular.module('tableApp', [])
.controller('tableCtrl', ['$scope', '$http', '$timeout',function($scope, $http)
{
$http.get('tableData/practionerData.json').success(function(data)
{
$scope.practionerData = data;
}
);
$http.get('tableData/practionerType.json').success(function(practionerdata)
{
$scope.practionerType = practionerdata;
}
);
$http.get('tableData/practionersByType.json').success(function(practionersByType)
{
$scope.practionersByType = practionersByType;
}
);
$http.get('tableData/OrganizationByPractioners.json').success(function(OrganizationByPractioners)
{
$scope.OrganizationByPractioners = OrganizationByPractioners;
}
);
}
])
Next step, I am going to populating the data in $scope with the JSON file. I can do it with the web service also. I can also post the scope data to the server with the web service. And here is the HTML page code:
<!DOCTYPE html>
<html lang='en' ng-app='tableApp'>
<head>
<title>Table Example</title>
<script src='https://ajax.googleapis.com/ajax/libs/angularjs/1.2.9/angular.js'></script>
<script src='js/controller.js' type='text/javascript'></script>
<link href='style.css' type='text/css' rel='stylesheet' />
</head>
<body ng-controller='tableCtrl'>
<div id="content">
<table id='practitioner_table' >
<tr>
<th colspan="7" style="text-align:right">
<i class="fa fa-search">search
<select ng-model="searchObj.practitionerType" ng-options="item.practitionerTypeID as item.practitionerTypeID for item in practitionerType">
<option value="">--Select--</option>
</select>
<select ng-disabled="!searchObj.practitionerType" ng-model="searchObj.practitioner_master_id" ng-options="item.practitioner_master_id as item.practitioner_name for item in practitionersByType| filter: {practitionerTypeID:searchObj.practitionerType}">
<option value="">--Select--</option>
</select>
<select ng-disabled="!searchObj.practitioner_master_id" ng-model="searchObj.organizationName" ng-options="item.organizationName as item.organizationName for item in OrganizationByPractitioners|filter:{practitioner_master_id:searchObj.practitioner_master_id}">
<option value="">--Select--</option>
</select>
</i>
</th>
</tr>
<tr>
<th><a href="#" ng-click="predicate = 'practitionerType';reverse=!reverse">Practitioner Type</a> </th>
<th><a href="#" ng-click="predicate = 'practitioner_name';reverse=!reverse">Practitioner Name</a></th>
<th><a href="#" ng-click="predicate = 'organizationName';reverse=!reverse">Organization Name</a></th>
</tr>
<tr ng-repeat="practitioner in practitionerData | orderBy:predicate:reverse| filter: {practitionerType:searchObj.practitionerType,practitioner_master_id:searchObj.practitioner_master_id,organizationName:searchObj.organizationName}">
<td ng-class-odd="'odd'" ng-class-even="'even'"> {{practitioner.practitionerType}}</td>
<td ng-class-odd="'odd'" ng-class-even="'even'"> {{practitioner.practitioner_name}}</td>
<td ng-class-odd="'odd'" ng-class-even="'even'"> {{practitioner.organizationName}}</td>
</tr>
</table>
</div>
</body>
Finally, I am populating the dropdown and table with scope data. Remember that the <select> tag and I am cascading the dropdowns using “filter” in “ng-options”. “ng-disabled” is used to keep the child dropdown disabled until the parent dropdown has the value.
AngularJS with Free ASP.NET Hosting
Try our AngularJS with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
