In this article, we will learn how to create a password and confirm password matching validation in an Angular application. Password and confirm password matching validation is generally required in the registration form and password reset or create form.
Prerequisites
Basic knowledge of Angular
Visual Studio Code
Node and NPM installed
Bootstrap
For this article, I have created an Angular project using Angular 12. For creating an Angular project, we need to follow the following steps:
Create an Angular Project
Let's create a new angular project by using the following command.
ng new PassValidationExample
Open a project in Visual Studio Code using the following commands.
cd PassValidationExample
Code .
Now in Visual Studio, your project looks as below.
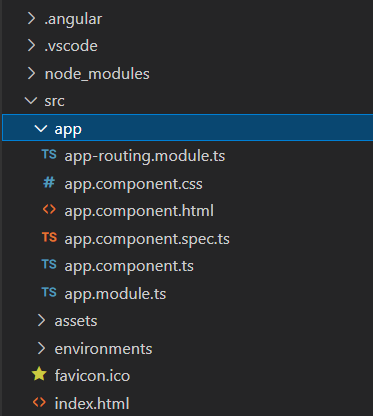
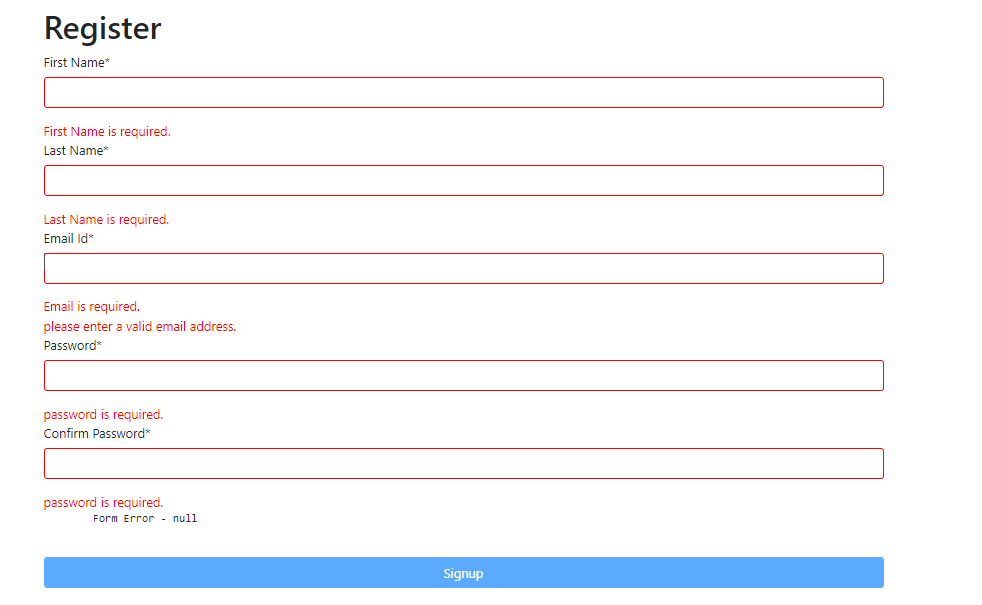
For validation, we need to import Validators from '@angular/forms'
import { FormGroup, FormControl, FormBuilder, Validators } from '@angular/forms';
App.component.html
<div class="container">
<div style="margin: 30px">
<h1 class="ion-text-center">Register</h1>
<form [formGroup]="loginForm">
<div class="form-group">
<label>First Name*</label>
<input class="form-control" formControlName="fname" type="text" />
</div>
<div class="error-messages">
<ng-container *ngFor="let error of error_messages.fname">
<div class="error-message" *ngIf="loginForm.get('fname').hasError(error.type) && (loginForm.get('fname').dirty || loginForm.get('fname').touched)">
{{ error.message }}
</div>
</ng-container>
</div>
<div class="form-group">
<label>Last Name*</label>
<input class="form-control" formControlName="lname" type="text" />
</div>
<div class="error-messages">
<ng-container *ngFor="let error of error_messages.lname">
<div class="error-message" *ngIf="loginForm.get('lname').hasError(error.type) && (loginForm.get('lname').dirty || loginForm.get('lname').touched)">
{{ error.message }}
</div>
</ng-container>
</div>
<div class="form-group">
<label>Email Id*</label>
<input class="form-control" formControlName="email" type="text" />
</div>
<div class="error-messages">
<ng-container *ngFor="let error of error_messages.email">
<div class="error-message" *ngIf="loginForm.get('email').hasError(error.type) &&
(loginForm.get('email').dirty || loginForm.get('email').touched)">
{{ error.message }}
</div>
</ng-container>
</div>
<div class="form-group">
<label>Password*</label>
<input class="form-control" formControlName="password" type="password" />
</div>
<div class="error-messages">
<ng-container *ngFor="let error of error_messages.password">
<div class="error-message" *ngIf="loginForm.get('password').hasError(error.type) && (loginForm.get('password').dirty || loginForm.get('password').touched)">
{{ error.message }}
</div>
</ng-container>
</div>
<div class="form-group">
<label>Confirm Password*</label>
<input class="form-control" formControlName="confirmpassword" type="password" />
</div>
<div class="error-messages">
<ng-container *ngFor="let error of error_messages.confirmpassword">
<div class="error-message" *ngIf="loginForm.get('confirmpassword').hasError(error.type) && (loginForm.get('confirmpassword').dirty || loginForm.get('confirmpassword').touched)">
{{ error.message }}
</div>
</ng-container>
</div>
<pre>
Form Error - {{ loginForm.errors | json }}
</pre>
<button class="form-control btn btn-primary" [disabled]="!loginForm.valid">Signup</button>
</form>
</div>
</div>
App.component.ts
import { Component } from '@angular/core';
import { FormGroup, FormControl, FormBuilder, Validators } from '@angular/forms';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
loginForm: FormGroup;
error_messages = {
'fname': [
{ type: 'required', message: 'First Name is required.' },
],
'lname': [
{ type: 'required', message: 'Last Name is required.' }
],
'email': [
{ type: 'required', message: 'Email is required.' },
{ type: 'minlength', message: 'Email length.' },
{ type: 'maxlength', message: 'Email length.' },
{ type: 'required', message: 'please enter a valid email address.' }
],
'password': [
{ type: 'required', message: 'password is required.' },
{ type: 'minlength', message: 'password length.' },
{ type: 'maxlength', message: 'password length.' }
],
'confirmpassword': [
{ type: 'required', message: 'password is required.' },
{ type: 'minlength', message: 'password length.' },
{ type: 'maxlength', message: 'password length.' }
],
}
constructor(
public formBuilder: FormBuilder
) {
this.loginForm = this.formBuilder.group({
fname: new FormControl('', Validators.compose([
Validators.required
])),
lname: new FormControl('', Validators.compose([
Validators.required
])),
email: new FormControl('', Validators.compose([
Validators.required,
Validators.minLength(6),
Validators.maxLength(30)
])),
password: new FormControl('', Validators.compose([
Validators.required,
Validators.minLength(6),
Validators.maxLength(30)
])),
confirmpassword: new FormControl('', Validators.compose([
Validators.required,
Validators.minLength(6),
Validators.maxLength(30)
])),
}, {
validators: this.password.bind(this)
});
}
ngOnInit() {
}
password(formGroup: FormGroup) {
const { value: password } = formGroup.get('password');
const { value: confirmPassword } = formGroup.get('confirmpassword');
return password === confirmPassword ? null : { passwordNotMatch: true };
}
}
App.component.css
p {
font-family: Lato;
}
.error-message {
color: red;
}
input.ng-touched.ng-invalid {
border: 1px solid red;
}
Now run the project using the following command
npm start
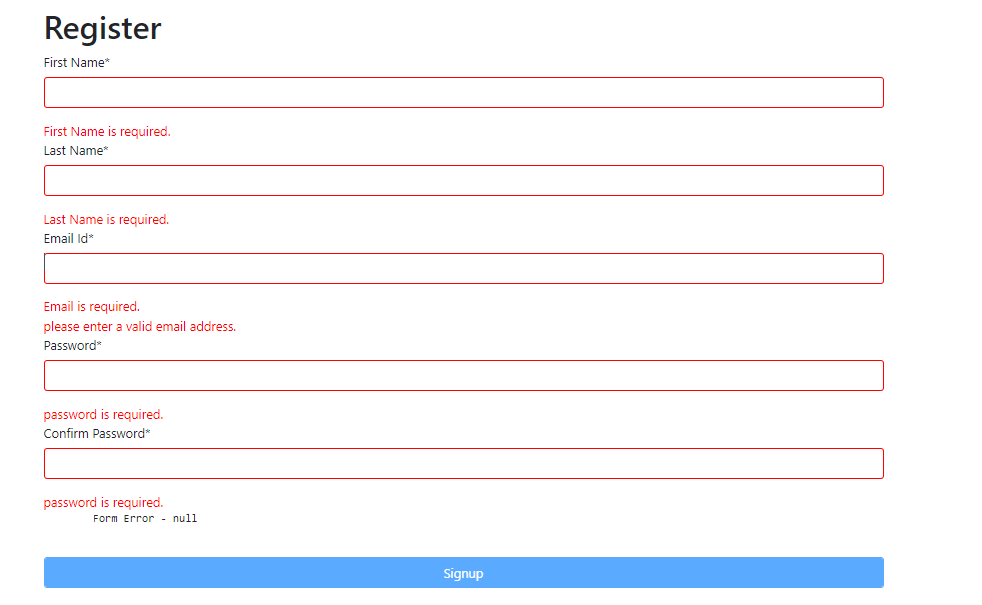
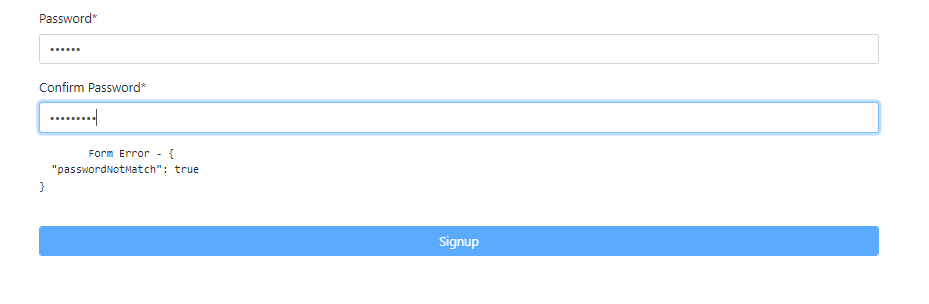
In this article, I have discussed the implementation of password and confirm password matching validation in Angular application.