
July 19, 2024 07:38 by
Peter
Angular's built-in Router module can be used to dynamically highlight the active menu item in an Angular application based on the current path. You can now apply a class to the active link as a result.
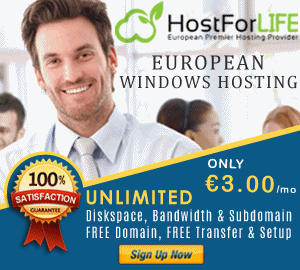
Here's how to accomplish it.
Step 1. Import the RouterModule
Ensure that your Angular module—typically app.module.ts—has the RouterModule set.
import { RouterModule } from '@angular/router';
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Step 2. Update your HTML
Use the routerLinkActive directive to dynamically apply a class to the active menu item. Here’s how you can update your navigation HTML.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Navbar Example</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<style>
.navbar-nav .nav-item a {
color: black;
text-decoration: none;
}
.navbar-nav .nav-item a:hover {
color: #0056b3; /* Optional: Change color on hover */
}
.navbar-nav .home-link i {
color: blue;
}
.active-link {
color: blue !important; /* Customize the active link color */
}
</style>
</head>
<body>
<section class="wrapper megamenu-wraper">
<div class="">
<nav class="container navbar navbar-expand-lg main-menu">
<div class="">
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav mr-auto">
<li class="nav-item">
<a routerLink="/private/po" routerLinkActive="active-link" class="home-link active">
<i class="fa fa-home"></i>
</a>
</li>
<li class="nav-item">
<a routerLink="dashboard" routerLinkActive="active-link">Dashboard</a>
</li>
<li class="nav-item">
<a routerLink="default" routerLinkActive="active-link">Student List</a>
</li>
<li class="nav-item">
<a routerLink="camps" routerLinkActive="active-link">Activities</a>
</li>
<li class="nav-item">
<a routerLink="camp-attendence" routerLinkActive="active-link">Attendance</a>
</li>
<li class="nav-item">
<a [routerLink]="['/private/po/actvity-camp', 0]" routerLinkActive="active-link">Create Activity</a>
</li>
</ul>
</div>
</div>
</nav>
</div>
</section>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
</body>
</html>
Explanation
- routerLinkActive Directive: This directive applies the specified class (active-link in this case) to the link that matches the current route.
- routerLink Directive: This binds the link to the router path.
- CSS: The active-link class is styled to indicate the active state.
Step 3. Ensure router module configuration
Make sure your Angular router is correctly configured in your application module (usually app-routing.module.ts).
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { DashboardComponent } from './dashboard/dashboard.component';
import { StudentListComponent } from './student-list/student-list.component';
import { ActivitiesComponent } from './activities/activities.component';
import { AttendanceComponent } from './attendance/attendance.component';
import { CreateActivityComponent } from './create-activity/create-activity.component';
const routes: Routes = [
{ path: 'dashboard', component: DashboardComponent },
{ path: 'default', component: StudentListComponent },
{ path: 'camps', component: ActivitiesComponent },
{ path: 'camp-attendence', component: AttendanceComponent },
{ path: 'private/po/actvity-camp/:id', component: CreateActivityComponent },
{ path: '**', redirectTo: 'dashboard', pathMatch: 'full' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
This setup ensures that when you navigate through your app, the active link will be highlighted, providing a better user experience.

